Context Hooks in React
Last Updated :
20 Feb, 2024
Context Hooks are a feature in React that allows components to consume context values using hooks. Before Hooks, consuming context required wrapping components in Consumer
or using a Higher Order Component (HOC). Context Hooks streamline this process by providing a more intuitive and concise way to access context values within functional components.
We will learn about the following concepts of Context Hooks in React
Understanding Context Hooks in React:
In the realm of React development, managing state across various components efficiently is a pivotal aspect. Context API, introduced in React 16.3, revolutionized state management by providing a way to pass data through the component tree without having to pass props down manually at every level. However, working with Context API often involved boilerplate code and complexity. With the introduction of Context Hooks, specifically useContext
, React developers were bestowed with a simpler and more elegant solution.
Key Context Hooks:
- useContext: The
useContext
hook is the primary hook for consuming context in functional components. It takes a context object created by React.createContext()
and returns the current context value for that context. This hook allows components to access context values without nesting additional components or using render props.
- useContext in Practice: To utilize
useContext
, first, a context object needs to be created using React.createContext()
. This context object is then provided to the tree using a Provider
. Finally, within any functional component nested within the Provider
, useContext
can be invoked to access the context value.
Benefits of Context Hooks:
- Simplicity: Context Hooks simplify the process of consuming context in functional components, reducing the need for wrapper components or HOCs.
- Avoids Prop Drilling: Context Hooks eliminate the need for prop drilling, where props are passed down through multiple levels of components. This leads to cleaner and more maintainable code.
- Encourages Component Composition: By providing a cleaner way to consume context, Context Hooks encourage better component composition and organization within React applications.
useContext Hook:
Context enables passing data/state through the component tree without manual prop passing. It’s for sharing global data like user authentication or theme. Context API uses Provider and Consumer components, but it’s verbose. useContext hook, introduced in React 16.8, makes code more readable and removes the need for Consumers.
Syntax:
const authContext = useContext(initialValue);
Example: Below is the code example for the useContext Hook:
Javascript
import React,
{
useState
} from "react" ;
import Auth from "./Auth" ;
import AuthContext
from "./auth-context" ;
const App = () => {
const [authstatus, setauthstatus] = useState( false );
const login = () => {
setauthstatus( true );
};
return (
<React.Fragment>
<AuthContext.Provider
value={
{
status: authstatus,
login: login
}
}>
<Auth />
</AuthContext.Provider>
</React.Fragment>
);
};
export default App;
|
Javascript
import React,
{
useContext
} from "react" ;
import AuthContext from "./auth-context" ;
const Auth = () => {
const auth = useContext(AuthContext);
console.log(auth.status);
return (
<div>
<h1>Are you authenticated?</h1>
{
auth.status ?
<p>Yes you are</p> : <p>Nopes</p>
}
<button onClick={auth.login}>
Click To Login
</button>
</div>
);
};
export default Auth;
|
Javascript
import React from 'react' ;
const authContext = React.createContext({status: null ,login:()=>{}});
export default authContext;
|
Steps to Run the App:
npm start
Output:
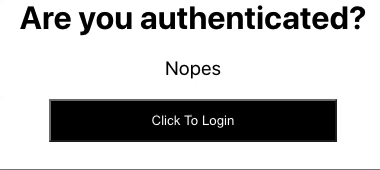
Share your thoughts in the comments
Please Login to comment...