How to Create Props Proxy for HOC Components ?
Last Updated :
05 Dec, 2023
We will delve into the process of creating a props proxy for higher-order components (HOCs). This approach enables the addition of props to wrapped components before rendering, proving useful in various scenarios such as logging, modifying data passed to components, and managing authentication in diverse applications.
Prerequisites:
Approach:
- Developing a functional component named “MyComponent” that receives props and returns them as an HTML element.
- In the main file ‘App.js,’ responsible for rendering, we import the aforementioned components.
- MyComponent is passed as a prop to the WithPropsProxy component, stored in a constant variable ‘WrappedComponent.’
- Subsequently, we render the ‘WrappedComponent’ by providing specific props to it.
Steps to Create the React Application:
Step 1: Create a React application using the following command.
npx create-react-app foldername
Step 2: After creating your project folder i.e. foldername, move to it using the following command.
cd foldername
Project Structure:
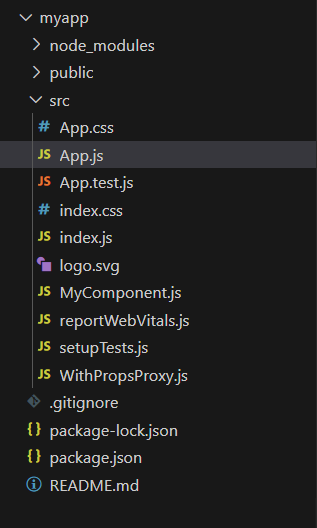
Project Structure
The updated dependencies in package.json file will look like:
"dependencies": {
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4",
}
Example: Creating a component so that we can pass it to another function component and you will get to now that how props proxy works in HOC components.
Javascript
import React from 'react' ;
const WithPropsProxy = (WrappedComponent) => {
return function (props) {
const newProps = {
Name: 'High order component' ,
Topic: 'ReactJs' ,
Level: 'easy'
};
return <WrappedComponent {...props}
{...newProps} />;
};
};
export default WithPropsProxy;
|
Javascript
import React from 'react' ;
const MyComponent = (props) => {
return (
<div>
<p>{props.Name}</p>
<p>{props.Topic}</p>
<p>{props.Level}</p>
<p>{props.otherProp}</p>
</div>
);
};
export default MyComponent;
|
Javascript
import './App.css' ;
import WithPropsProxy from './WithPropsProxy' ;
import MyComponent from './MyComponent' ;
const WrappedComponent = WithPropsProxy(MyComponent);
function App() {
return (
<div className= "center" >
<h3>
How to create props proxy for HOC component?
</h3>
<WrappedComponent otherProp= "GeekForGeeks" />
</div>
);
}
export default App;
|
Steps to Run the application:
npm start
Output:
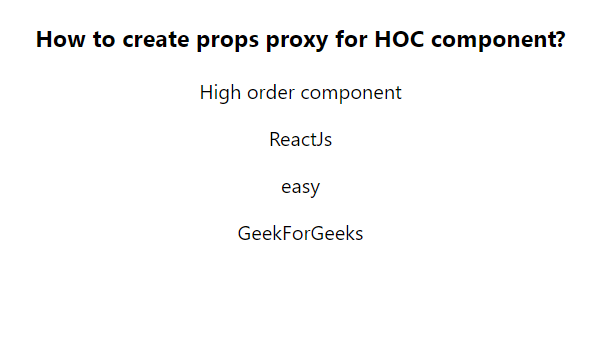
Output
Share your thoughts in the comments
Please Login to comment...