How to set up the Provider Component in a React application ?
Last Updated :
06 Feb, 2024
In React applications, the Provider component is often used in conjunction with context to provide data to components deep down in the component tree without having to explicitly pass props through every level of the tree.
Prerequisites:
Steps to Create React Application:
Step 1: Create a React application using the following command:
npx create-react-app foldername
Step 2: After creating your project folder i.e. foldername, move to it using the following command:
cd foldername
Step 3: After setting up react environment on your system, we can start by creating an App.js file and create a file by the name of components in which we will write our desired function.
Project Structure:
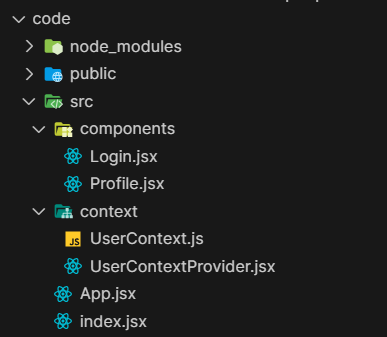
The updated dependencies in package.json file will look like:
"dependencies": {
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
Approach:
Follow the below steps to set up the Provider component in a React application:
- Create the Context:
- First, you need to create a context using the createContext function provided by React. This context will hold the data you want to provide to components.
- Create the Provider Component:
- Next, create a provider component that wraps around the components you want to provide the context to. This provider component will use the Provider component provided by the UserContext.
- Wrap Your App with the Provider:
- In your main App component or any parent component, wrap your component tree with the Provider component you just created.
- Use the Context in Components:
- Now, any component that needs access to the data provided by the context can access it by using the useContext hook or by consuming the context using the Consumer component.
Example: Below is the basic implementation of the above steps:
Javascript
import Login
from "./components/Login"
import Profile
from "./components/Profile"
import UserContextProvider
from "./context/UserContextProvider"
function App() {
return (
<UserContextProvider>
<h1>
React Provider
</h1>
<Login />
<Profile />
</UserContextProvider>
)
}
export default App
|
Javascript
import React from 'react'
const UserContext = React.createContext()
export default UserContext;
|
Javascript
import React from "react" ;
import UserContext from "./UserContext" ;
const UserContextProvider =
({ children }) => {
const [user, setUser] =
React.useState( null )
return (
<UserContext.Provider
value={{ user, setUser }}>
{children}
</UserContext.Provider>
)
}
export default UserContextProvider;
|
Javascript
import React,
{
useState,
useContext
} from 'react'
import UserContext
from '../context/UserContext'
function Login() {
const [username, setUsername] = useState( '' )
const [password, setPassword] = useState( '' )
const { setUser } = useContext(UserContext)
const handleSubmit =
(e) => {
e.preventDefault()
setUser({ username, password })
}
return (
<div>
<h2>Login</h2>
<input type= 'text'
value={username}
onChange={
(e) =>
setUsername(e.target.value)
}
placeholder= 'username' />
<input type= 'text'
value={password}
onChange={
(e) => setPassword(e.target.value)
}
placeholder= 'password' />
<button onClick={handleSubmit}>
Submit
</button>
</div>
)
}
export default Login
|
Javascript
import React,
{
useContext
} from 'react'
import UserContext
from '../context/UserContext'
function Profile() {
const { user } = useContext(UserContext)
if (!user) return <div>please login</div>
return <div>Welcome {user.username}</div>
}
export default Profile;
|
Output:
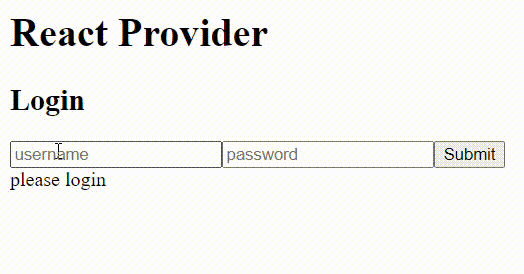
Output
Share your thoughts in the comments
Please Login to comment...