Conditionals in Pug View Engine
Last Updated :
18 Mar, 2024
Pug supports JavaScript natively, hence using JavaScript expressions, we can format HTML code. This approach allows us to reuse static web pages which have dynamic data. Angular brackets are not used while specifying the tags.
Conditional Statement in Pug
Conditional statements allow you to execute specific blocks of code based on conditions. If the condition meets then a particular block of statements will be executed otherwise it will execute another block of statement that satisfies that particular condition.
Several methods used to perform Conditional Statements in Pug are:
- if statement: Executes a code block if a specified condition is true.
- else statement: Executes a block of code if the same condition of the preceding if statement is false.
- else if statement: Adds more conditions to the if statement, allowing multiple alternative conditions to be tested.
Syntax
if condition
// pug code
else
// pug code
Syntax for else if
if condition
// pug code
else if condition
//pug code
else
//pug code
Approach to implement Conditionals in Pug View Engine:
- Express Setup: Initializes an Express.js server.
- Setting View Engine: Configures Pug as the view engine for rendering templates.
- Routing: Defines a single route for the root URL (/). When accessed, it renders the Pug template.
- Pug Template: The Pug template defines the structure and content of the HTML page along with the attributes.
- Styling: Internal CSS is used within the Pug template (style.) to set margins and styles for headings and other components.
Steps to Install Pug in Node App:
Step 1: Create a NodeJS Application using the following command:
npm init -y
Step 2: Install the required dependencies using the following command:
npm i pug express
Step 3: Create a views folder that contains the pug file.
Folder Structure:
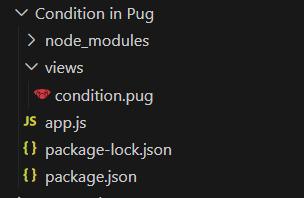
Folder Structure
The updated dependencies in package.json file will look like:
"dependencies": {
"express": "^4.18.2",
"pug": "^3.0.2"
}
Example 1: Conditional Statement in Pug
HTML
doctype html
html(lang="en")
head
meta(charset="UTF-8")
meta(name="viewport", content="width=device-width, initial-scale=1.0")
title GeeksforGeeks Pug
style.
body{
padding:1.2rem;
margin :2rem;
border:2px solid red;
text-align: center;
}
h1 {
color: green;
}
body
h1 Welcome to GeeksforGeeks
h3 Conditional Statement: if,else,else if
- let number = 27;
if number > 0
p The number #{number} is positive.
else if number < 0
p The number #{number} is negative.
else
p The number is zero.
Javascript
//app.js
const express = require("express");
const app = express();
const port = 3000;
app.set("view engine", "pug");
app.get("/", (req, res) => {
res.render("condition");
});
app.listen(port, () => {
console.log(`server is running at http://localhost:${port}`);
});
Output:
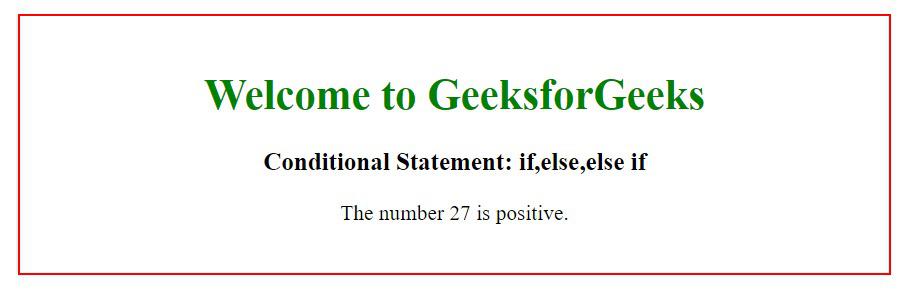
Conditional Statement in Pug
Example 2: Nested Conditional Statement in Pug
HTML
doctype html
html(lang="en")
head
meta(charset="UTF-8")
meta(name="viewport", content="width=device-width, initial-scale=1.0")
title GeeksforGeeks Pug
style.
body{
padding:1.2rem;
margin :2rem;
border:2px solid red;
text-align: center;
}
h1 {
color: green;
}
body
h1 Welcome to GeeksforGeeks
h3 Nested conditional Statement
- let number = 10;
if number == 10
if number < 15
p The number #{number} is smaller than 15.
if number < 12
p The number #{number} is smaller than 12 too.
else
p The number is greater than 15.
Javascript
//app.js
const express = require("express");
const app = express();
const port = 3000;
app.set("view engine", "pug");
app.get("/", (req, res) => {
res.render("condition");
});
app.listen(port, () => {
console.log(`server is running at http://localhost:${port}`);
});
Output:
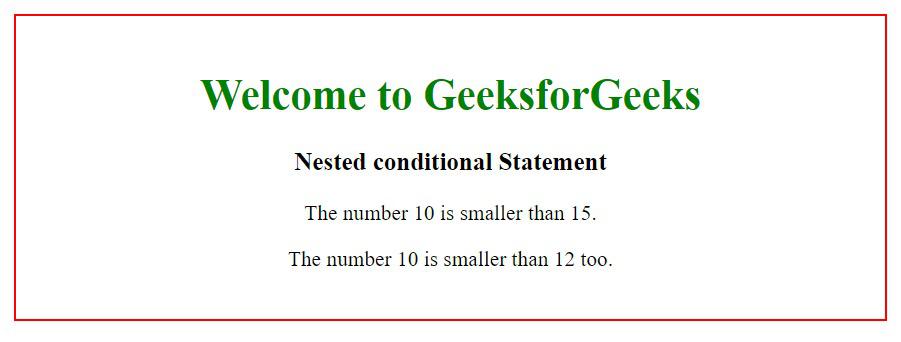
Nested Conditional Statement in Pug
Share your thoughts in the comments
Please Login to comment...