How to use conditional statements with EJS Template Engine ?
Last Updated :
01 Mar, 2024
Conditional statements in EJS templates allow you to dynamically control the content that is rendered based on specified conditions. Similar to JavaScript, EJS provides a syntax for using conditional logic within your HTML templates. In this article, we will see a practical example of using conditional statements with the EJS template.
What are Conditional Statements in EJS ?
EJS enables you to embed JavaScript code within HTML markup, providing the evaluation of conditions and the generation of dynamic content. By this you can apply a variety of conditional statements, including if-else blocks, ternary operators, and loops, to control the rendering of content based on specific conditions.
Syntax to use conditional statements in EJS :
<% if (condition) { %>
<!-- Code to be executed if the condition is true -->
<% } else { %>
<!-- Code to be executed if the condition is false -->
<% } %>
Approach to use conditional statements with EJS templates:
- We have a form in the EJS template that allows users to toggle a login status.
- In the server code, it handles the rendering of the template and updates the login status based on the user input.
- By using the condtional statement of <% if (isLoggedIn) { %> code <% } else { %>. We are managing the dynamic content to display on the screen.
Steps to use conditional statements with EJS templates:
Step 1: Firstly, we will make the folder named root by using the below command in the VScode Terminal. After creation use the cd command to navigate to the newly created folder.
mkdir root
cd root
Step 2: Once the folder is been created, we will initialize NPM using the below command, this will give us the package.json file.
npm init -y
Step 3: Once the project is been initialized, we need to install Express and EJS dependencies in our project by using the below installation command of NPM.
npm i express ejs
Step 4: Now create the below Project Structure in your project which includes app.js, and views/index.ejs.
Folder Structure:
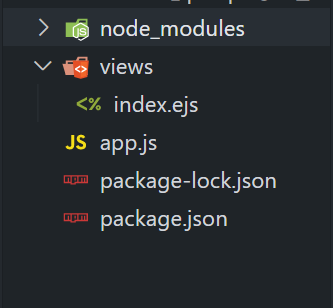
The updated dependencies in package.json will look like:
"dependencies": {
"express": "^4.18.2",
"ejs": "^3.1.9",
}
Example: We need to write the code for the app.js file, and code for views/index.ejs to include a conditional statement within a EJS template.
HTML
<!DOCTYPE html>
< head >
< title >Conditional Statements with EJS</ title >
</ head >
< body >
< h1 style = "color: green;" >GeeksforGeeks</ h1 >
< h3 >Conditional Statements with EJS</ h3 >
< form action = "/" method = "post" >
< label for = "loginStatus" >Toggle Login Status:</ label >
< select name = "loginStatus" id = "loginStatus" >
< option value = "true" >Logged In</ option >
< option value = "false" >Logged Out</ option >
</ select >
< button type = "submit" >Toggle</ button >
</ form >
<% if (isLoggedIn) { %>
< p >You are logged in!</ p >
<% } else { %>
< p >Please log in to access the content.</ p >
<% } %>
</ body >
</ html >
|
Javascript
const express = require( 'express' );
const bodyParser = require( 'body-parser' );
const app = express();
const port = 3000;
app.set( 'view engine' , 'ejs' );
app.use(bodyParser.urlencoded({ extended: true }));
let isLoggedIn = false ;
app.get( '/' , (req, res) => {
res.render( 'index' , { isLoggedIn });
});
app.post( '/' , (req, res) => {
const { loginStatus } = req.body;
isLoggedIn = loginStatus === 'true' ;
res.redirect( '/' );
});
app.listen(port, () => {
console.log(`Server is running at http:
});
|
To run the application, we need to start the server by using the below command.
node app.js
Output:
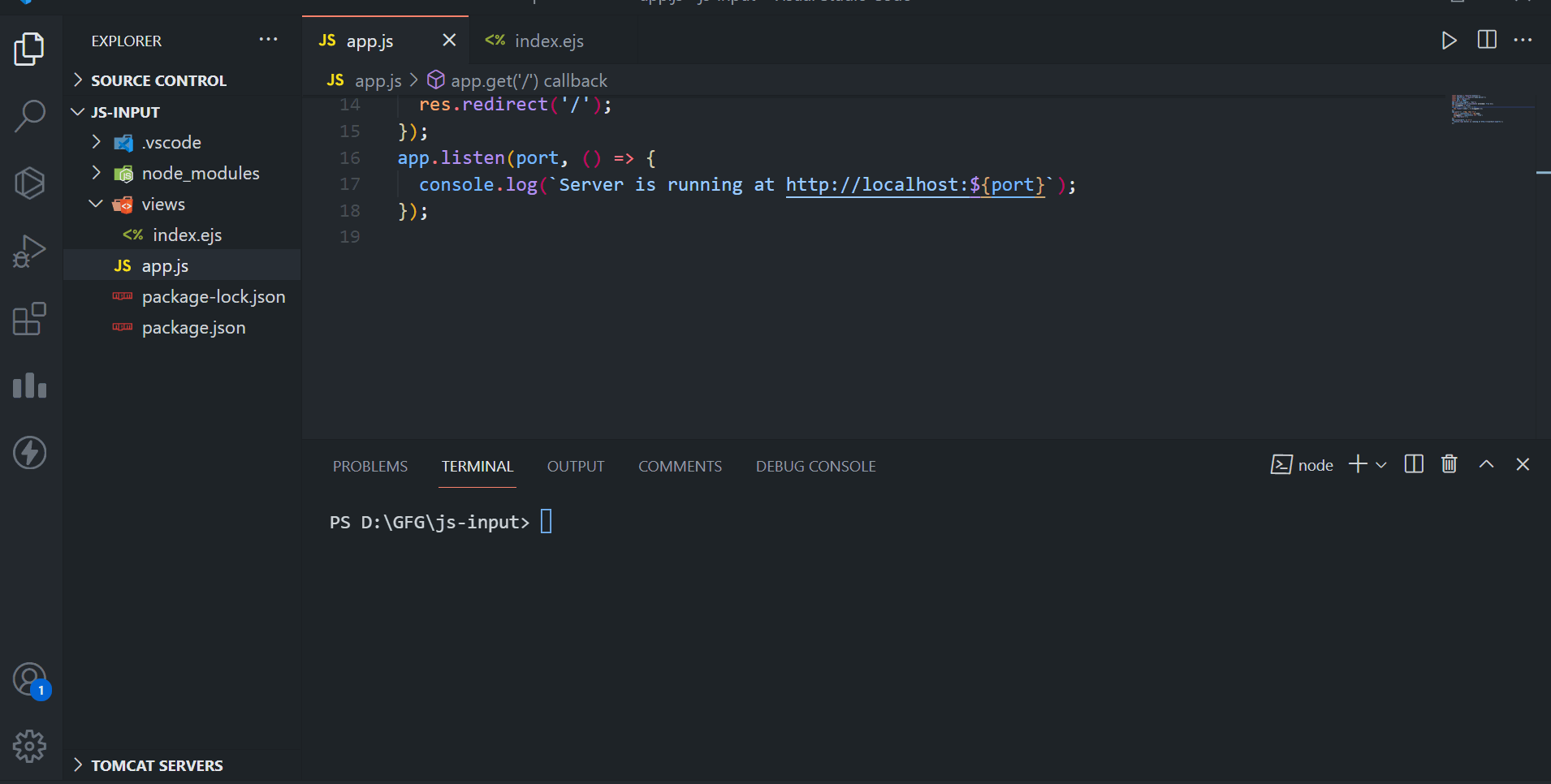
Share your thoughts in the comments
Please Login to comment...