How to use array of objects in EJS Template Engine ?
Last Updated :
06 Mar, 2024
Arrays of objects are collections of JavaScript objects stored within an array. Each object within the array can contain multiple key-value pairs, making them versatile for organizing various types of data. For example, you might have an array of objects representing users, products, or posts in a social media application.
Here’s an example of an array of objects representing users:
Javascript
const users = [
{ id: 1, name: 'Ram' , age: 30 },
{ id: 2, name: 'Shyam' , age: 25 },
{ id: 3, name: 'Hari' , age: 40 }
];
|
Benefits:
- Dynamic Content: Using an array of objects in EJS templates allows for dynamic rendering of data, making it easy to display lists, tables, or any other structured data.
- Reusable Templates: Once created, EJS templates can be reused across multiple pages or components, making it easy to maintain consistent UI elements throughout the application.
- Flexibility: EJS provides flexibility in how data is displayed and formatted, allowing developers to customize the presentation of array of objects based on specific requirements.
Integrating Arrays of Objects into EJS Templates
EJS templates allow you to embed JavaScript code directly into your HTML markup. This makes it straightforward to iterate over arrays of objects and dynamically generate HTML content based on the data.
Step 1: Create a project folder using the following command.
mkdir ejs-array-example
cd ejs-array-example
Step 2: Initialize the node project.
npm init -y
Step 3: Install the required dependencies:
npm install express ejs
Folder Structure:

The updated dependencies in package.json file will look like:
"dependencies": {
"ejs": "^3.1.9",
"express": "^4.18.3"
}
Example: Create the required files as suggested in folder structure and add the following code.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content = "width=device-width, initial-scale=1.0" >
< title >User List</ title >
</ head >
< body >
< h1 >User List</ h1 >
< ul >
<% users.forEach(user=> { %>
< li >ID: <%= user.id %>, Name: <%= user.name %>, Age: <%= user.age %>
</ li >
<% }); %>
</ ul >
</ body >
</ html >
|
Javascript
const express = require( 'express' );
const app = express();
const port = 3000;
app.set( 'view engine' , 'ejs' );
app.get( '/' , (req, res) => {
const users = [
{ id: 1, name: 'Ram' , age: 30 },
{ id: 2, name: 'Shyam' , age: 25 },
{ id: 3, name: 'Hari' , age: 40 }
];
res.render( 'users' , { users: users });
});
app.listen(port, () => {
console.log(`Server is running on http:
});
|
To start the application run the following command:
node server.js
Output:
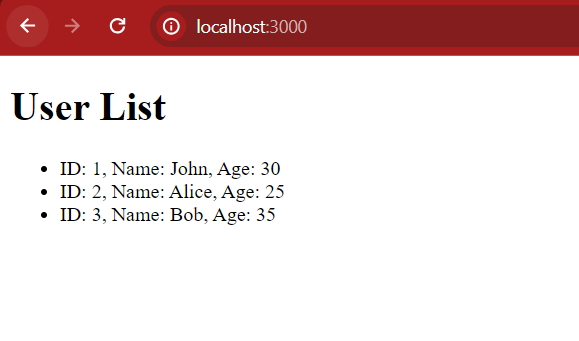
Share your thoughts in the comments
Please Login to comment...