Using Mixins with Pug View Engine
Last Updated :
07 Mar, 2024
Pug is a template/view engine that works with JavaScript libraries and frameworks. It simplifies the process of writing HTML by reducing traditional HTML syntax. Among all of these features, it also provides support for Mixins. Mixins is a block of code that can be reused in multiple templates.
In this article, we will learn how to use Mixins with its syntax and examples.
Approach to use Mixins with PugJS:
Mixins is a block of code that can be reused. It is declared by using the mixin keyword with the name of the mixin and inside that we will define a block of code that we are going to reuse. To reuse the mixin block we have to use +mixin_name.
Syntax of mixins:
//Declaration
mixin mixin_name
//block of code
//Using Declared Mixin
+mixin_name
+mixin_name
Adding a Mixins in Pug:
Suppose you have a form element intended for multiple instances across your application, each requiring independent styling. While you could employ conditional logic, this approach may lack efficiency. Instead, leveraging PugJS mixin functionality offers a more optimal solution. We’ll delve into an advanced example utilizing attributes in mixins. Let’s start by crafting a partial file to define our mixin. Within the partials folder, establish a new file named mixins.pug, housing the subsequent content.
mixin user-form(attributes)
form(action="/" method="post" class!=attributes.class)
input#user-email(type="email" name="user-email" class!=attributes.emailClass)
button(type="submit") Update
After declaring our user form mixin, the intriguing aspect lies in class!=attributes.class. As outlined in the Pug documentation, mixins receive an implicit ‘attributes’ argument, which we’ll utilize to append attributes to our form. Now, let’s integrate our form into action. Insert the following line at the top of main-layout.pug, just above the DOCTYPE declaration.
include ../partials/mixins
By following this approach, our mixin becomes accessible from any file that extends main-layout. To integrate the mixin into index.pug, append the following line at the end of the file (ensuring correct indentation):
+user-form
Upon visiting the home page in your browser, you’ll notice the form at the bottom of the page without any added class. Let’s now incorporate the form in a different location and assign it a class. In about.pug, append the following line as the last line of the file:
+user-form()(class='modal')
By specifying this usage, we’ve indicated the application of the ‘modal’ class to the form. Upon visiting the ‘about’ page, you should observe the form with the appropriate class applied.
Steps to use Mixins with Pug View Engine
Step 1: Create a NodeJS application using the following command:
npm init -y
Step 2: Install required Dependencies using the following command.
npm i pug express
Project Structure:
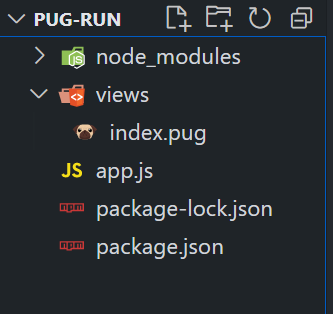
The updated dependencies in package.json file will look like:
"dependencies": {
"express": "^4.18.2",
"pug": "^3.0.2"
}
Example: This example demonstrate the use of Mixins in Pug.js with different approaches
HTML
// File path: views/index.pug
html
head
// Set the title of the HTML page
title Pug Mixin Example
// Internal CSS styles for the page
style.
h1 {
color: green;
}
body
// Display the main heading of the page
h1 GeeksForGeeks | Mixins Example
// Approach 1: Basic Mixin without Parameters
// Declaration
mixin list
ul
li Java
li Web Development
li DSA
// Usege
b Approach 1: Basic Mixin without Parameters
+list
// Approach 2: Mixin with Parameter
// Declaration
mixin course(name)
li= name
// Usage
b Approach 2: Mixin with Parameter
ul
+course('Java')
+course('Web Development')
+course('DSA')
// Approach 3: Mixin with Conditional Block and Additional Content
// Declaration
mixin content(title)
h3=title
if block
block
else
p Content is Not provided
// Usage
b Approach 4: Mixin with Conditional Block and Additional Content
// Without Content
+content('GeeksForGeeks')
// With Content
+content('GeeksForGeeks')
p This is provided content
// Approach 5: Mixin with Dynamic List
// Declaration
mixin dynamic_list(id, ...items)
ul(id=id)
each item in items
li=item
//- Usage
b Approach 5: Mixin with Dynamic List
+dynamic_list('list', 1, 2, 3, 4)
|
Javascript
const express = require( 'express' );
const path = require( 'path' );
const app = express();
const port = 3000;
app.set( 'view engine' , 'pug' );
app.set( 'views' , path.join(__dirname, 'views' ));
app.get( '/' , (req, res) => {
res.render( 'index' );
});
app.listen(port, () => {
console.log(`Server is running at http:
});
|
To run the application use the following command:
node app.js
Output: Now go to http://localhost:3000 in your browser:
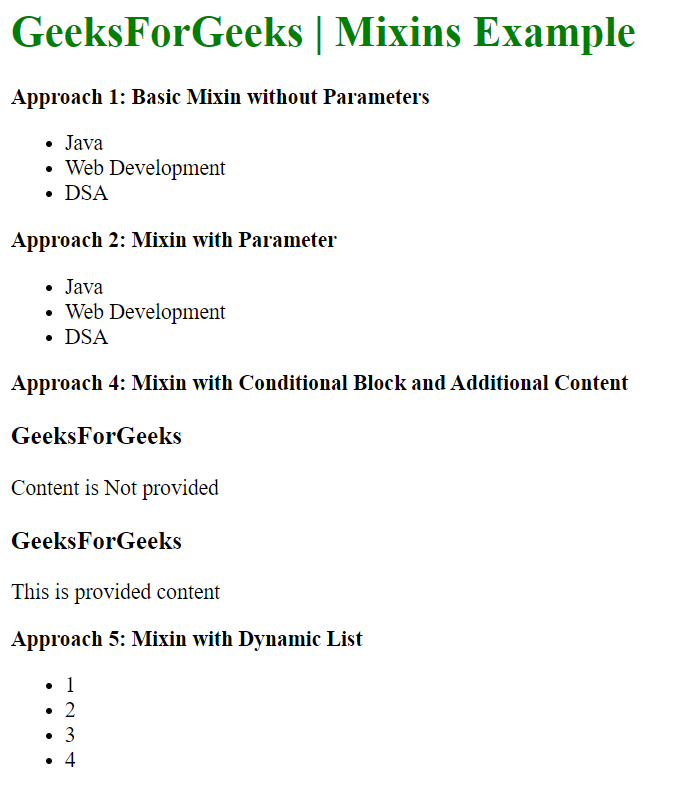
Share your thoughts in the comments
Please Login to comment...