template.js in Next JS
Last Updated :
13 Feb, 2024
Next JS is said to be the production-grade framework for React as it can handle both backend and frontend. One of the fundamental concepts is the ‘templates’. In this article, we will see about the template.js and how we can use it in our projects.
Prerequisites:
The “template.js” file was introduced in the app router and is similar to the “layout.js” file as it also wraps all the child pages or child routes and renders them to the user. The difference is that it creates a new instance of the page for each of its children while navigating, on the other hand, the “layout.js” maintains all the states defined in it. That basically means it does not maintain a state between its children and re-renders for every request and returns a new instance.
Syntax:
To create a template, you just need to create a file named “template.js” inside any of your routes/pages and export the component from it as shown below:
// inside template.js
export default function MyTemplate({children}){
// your code
return (
<>{children}</>
)
}
This is useful when you want to perform a common action whenever a user visits a page, through the “useEffect” hook as if you use the “useEffect” inside “layout.js”, then it will run for the only first time (or whenever any of it’s dependency changes) but in case of “template” it would run on every page. Or you might want to perform some other action on each page render which you can achieve through templates.
Steps to create the project:
Step 1: Run the following command in your terminal to start creating your next app:
npx create-next-app@latest
Step 2: Provide the necessary details as shown below:
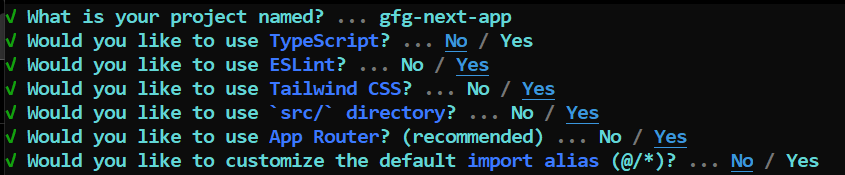
Providing necessary details to create-next-app
Step 3: Go into the create directory and start the development server:
cd gfg-next-app
npm run dev
Folder Structure:
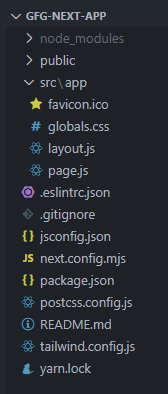
template.js Next.js Project Structure
The updated dependencies in package.json file will look like:
"dependencies": {
"react": "^18",
"react-dom": "^18",
"next": "14.1.0"
},
"devDependencies": {
"autoprefixer": "^10.0.1",
"postcss": "^8",
"tailwindcss": "^3.3.0",
"eslint": "^8",
"eslint-config-next": "14.1.0"
}
Example 1: Let us create two pages namely home page and contact page and share a common template throughout them by the “template.js”.
Javascript
import Link from "next/link" ;
export default function RootTemplate({ children }) {
return (
<div className= "p-5" >
<nav className= "mb-4" >
<h1 id= "logo" className= "text-2xl font-bold text-green-600" >
GeeksforGeeks
</h1>
<h3 className= "text-lg font-bold" >template.js | Next.js</h3>
<Link href= "/" className= "text-sm underline block" >
Home
</Link>
<Link href= "/contact" className= "text-sm underline block" >
Contact
</Link>
</nav>
{children}
</div>
);
}
|
Javascript
export default function Home() {
return <p className= "font-bold" >I am homepage.</p>;
}
|
Javascript
export default function Contact() {
return <p className= "font-bold" >I am contactpage.</p>;
}
|
Output:
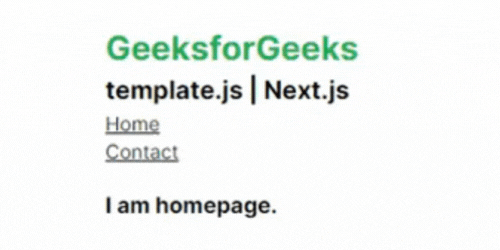
Output of example 1 template.js Next.js
Example 2: In this example, let’s add the code so that whenever user changes the page from home page to the about page, the background color of the page changes randomly between the specified colors. For this, we would need to make the “template.js” as a client component so as to use the react hooks in it.
Javascript
"use client" ;
import { useEffect } from "react" ;
import Link from "next/link" ;
export default function RootTemplate({ children }) {
useEffect(() => {
const colors = [ "#FFE4E1" , "#ADD8E6" , "#90EE90" ];
const randomColor = colors[Math.floor(Math.random() * colors.length)];
document.body.style.backgroundColor = randomColor;
}, []);
return (
<div className= "p-5" >
<nav className= "mb-4" >
<h1 id= "logo" className= "text-2xl font-bold text-green-600" >
GeeksforGeeks
</h1>
<h3 className= "text-lg font-bold" >template.js | Next.js</h3>
<Link href= "/" className= "text-sm underline block" >
Home
</Link>
<Link href= "/about" className= "text-sm underline block" >
About
</Link>
</nav>
{children}
</div>
);
}
|
Javascript
export default function Home() {
return <p className= "font-bold" >I am homepage.</p>;
}
|
Javascript
export default function AboutPage() {
return <p className= "font-bold" >I am aboutpage.</p>;
}
|
Output:
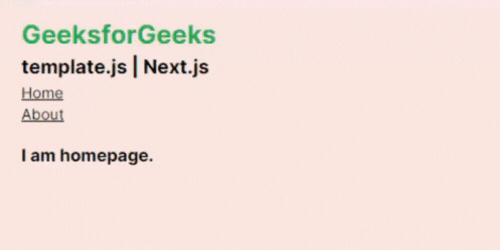
Output of example 2 template.js Next.js
Share your thoughts in the comments
Please Login to comment...