The Next JS stack, often referred to as the “N stack” (Next JS stack), is a comprehensive web development framework designed to streamline the creation of modern web applications. It is built around Next JS, a powerful React-based framework that adds additional capabilities for server-side rendering, routing, and more.
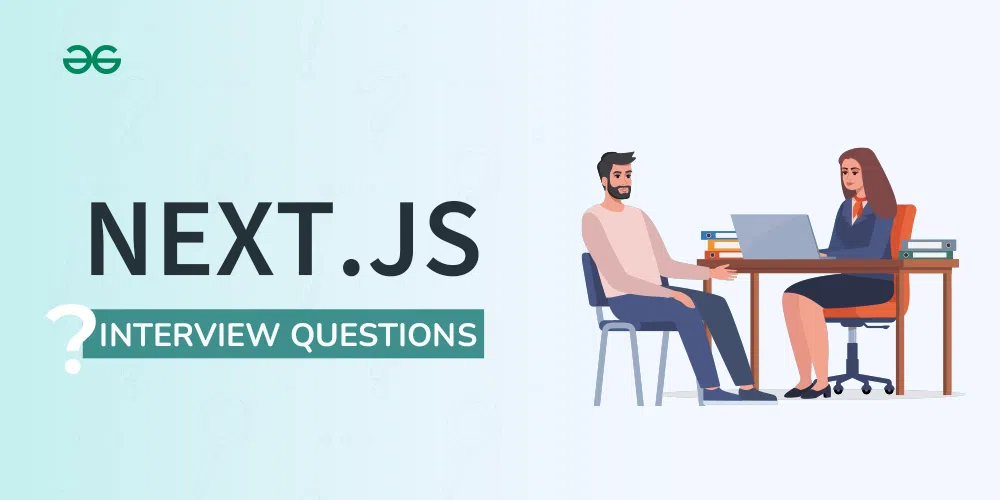
Let’s discuss some common Next JS interview questions that will help to clear interviews, particularly for frontend development roles. These questions are designed to assess your proficiency in Next JS and your ability to work on the front end of web applications.
1. What is Next JS?
Next JS is an open-source web development React-based framework created by Vercel, which is famous for its unique features such as Server-side rendering and enhanced SEO. It has some additional features such as data fetching utilities, dynamic API routes, optimized builds, etc. It is a framework built upon React, Webpack, and Babel.
2. How Next is different from other JavaScript frameworks?
Next.js is a JavaScript framework that is primarily designed for building React applications. Here are some key ways in which Next JS differs from other JavaScript frameworks:
- Server-Side Rendering (SSR): One of the significant distinctions of Next JS is its built-in support for server-side rendering. This allows pages to be rendered on the server rather than the client, providing benefits like improved SEO and faster initial page loads.
- Automatic Code Splitting: Next.js automatically splits the JavaScript code into smaller chunks, allowing for efficient loading of only the necessary code for a particular page.
- API Routes: Next.js makes it easy to create API routes within the same project, simplifying the development of backend functionality alongside the frontend.
- Built-in Image Optimization: The
next/image
component provides built-in support for image optimization, handling tasks like lazy loading and responsive images without the need for additional configuration.
- Easy Deployment: Next.js simplifies the deployment process with various options, including static site hosting, serverless deployment, and more. This ease of deployment is not always as straightforward in other frameworks.
3. What is the process of installing Next JS?
Below is the step by step process of installing the Next JS:
Steps to Install the Next JS:
Step 1: Node JS should be already installed in the system.
Step 2: Now create the next js app using the below command:
npx create-next-app myapp
Step 3: Now switch to the project directory:
cd myapp
Step 4: Next JS app is initialized by updating the package.json:
{
“scripts”: {
“dev”: “next”,
“build”: “next build”,
“start”: “next start”
}
}
4. Write a Hello World Program in Next JS?
In Next.js, creating a “Hello World” program involves setting up a simple React component within a file in the app
directory. Here’s a basic example:
Javascript
import React from 'react' ;
const HomePage = () => {
return (
<div>
<h1>Hello, Next.js!</h1>
</div>
);
};
export default HomePage;
|
5. Mention some features of Next JS.
Next.js is a powerful React framework that offers various features to simplify and enhance the development of web applications. Here are some key features of Next.js:
- Server-Side Rendering (SSR): Next.js allows server-side rendering, improving initial page load performance by rendering HTML on the server and sending it to the client.
- Static Site Generation (SSG): Next.js supports static site generation, enabling the pre-rendering of pages at build time, resulting in faster loading times and better SEO.
- File System-Based Routing: The routing system is based on the file structure of the “pages” directory, making it intuitive and easy to organize code.
- Automatic Code Splitting: Next.js automatically splits code into smaller chunks, loading only what’s necessary for each page. This enhances performance by reducing initial bundle sizes.
- API Routes: Easily create serverless functions by defining API routes alongside your pages, simplifying the development of server-side logic.
6. What do you mean by SSR?
SSR stands for Server-Side Rendering. It’s a technique used in web development where the server processes the React or other JavaScript framework code and generates the HTML on the server side, sending the fully rendered HTML to the client’s browser.
Here’s a brief overview of the SSR process:
- Request from Client: When a user makes a request to a server for a web page, the server receives the request.
- Server-Side Processing: Instead of sending just a blank HTML shell or a minimal document, the server executes the JavaScript code associated with the requested page, fetches data if needed, and renders the complete HTML content on the server side.
- Sending Rendered HTML to Client: The fully rendered HTML, along with any necessary CSS and JavaScript, is sent as a response to the client’s browser.
- Client-Side Hydration: Once the HTML is received by the browser, any JavaScript code needed for interactive elements or further client-side rendering is executed. This process is known as “hydration.”
7. What are the benefits of using Next JS?
Next.js is a popular React framework that brings several benefits to web development. Here are some of the key advantages of using Next.js:
- Server-Side Rendering (SSR):
- Next.js supports server-side rendering out of the box. This means that pages can be rendered on the server and then sent to the client, providing better performance and SEO as search engines can crawl the fully rendered content.
- Static Site Generation (SSG):
- Next.js allows for static site generation, where pages can be pre-built at build time. This can significantly improve performance by serving static files directly from a CDN, reducing the load on servers and improving the user experience.
- Automatic Code Splitting:
- Next.js automatically splits the code into smaller chunks, allowing for efficient loading of only the necessary code for a particular page. This results in faster initial page loads and improved overall performance.
- Built-in CSS Support:
- Next.js provides built-in support for styling solutions, including CSS modules, styled-jsx, and support for CSS-in-JS libraries. This allows developers to choose their preferred styling approach without the need for additional configuration.
- API Routes:
- Next.js allows you to create API routes easily, enabling the development of serverless functions. This can be useful for handling backend logic without the need for a separate server.
8. What is DOM?
DOM stands for Document Object Model. It is a programming interface for web documents. The DOM represents the structure of a document as a tree of objects, where each object corresponds to a part of the document, such as elements, attributes, and text.
Here are some key points about the Document Object Model:
- Tree Structure: The DOM represents an HTML or XML document as a tree structure. Each element, attribute, and piece of text in the document is represented by a node in the tree.
- Object-Oriented: The DOM is an object-oriented representation of a document. Each node in the tree is an object, and these objects can be manipulated using programming languages like JavaScript.
- Dynamic: The DOM is dynamic, meaning it can be modified programmatically. Developers can use scripting languages like JavaScript to manipulate the content, structure, and style of a document in real-time.
- Interface for Web Browsers: The DOM serves as an interface between web browsers and web documents. Browsers use the DOM to render and display web pages, and developers use it to interact with and modify the content of those pages.
9. How does Next JS handle client-side navigation?
Next.js uses a client-side navigation approach that leverages the HTML5 History API. This enables smooth transitions between pages on the client side without a full page reload. The framework provides a built-in Link
component that facilitates client-side navigation, and it supports both traditional anchor (<a>
) tags and programmatically navigating through the next/router
module.
Here’s an overview of how Next.js handles client-side navigation:
Link Component:
- The
Link
component is a core part of client-side navigation in Next.js. It is used to create links between pages in your application.
- Using the
Link
component, when users click the link, Next.js intercepts the navigation event and fetches the necessary resources for the new page without triggering a full page reload.
Javascript
import Link from 'next/link' ;
const MyComponent = () => (
<Link href= "/another-page" >
<a>Go to another page</a>
</Link>
);
|
Programmatic Navigation:
- In addition to using the
Link
component, Next.js provides a useRouter
hook and a router
object to allow for programmatic navigation. This is useful when you want to navigate based on user interactions or in response to certain events.
Javascript
import { useRouter }
from 'next/router' ;
const MyComponent = () => {
const router = useRouter();
const handleClick = () => {
router.push( '/another-page' );
};
return (
<button onClick={handleClick}>
Go to another page
</button>
);
};
|
10. Explain the concept of dynamic routing in Next JS:
Dynamic routing in Next.js refers to the ability to create routes for pages with dynamic parameters, allowing you to build pages that can handle different data or content based on the values of these parameters. Instead of creating a separate page for each variation, you can use a single page template and dynamically generate content based on the provided parameters.
11. What is meant by Styled JSX in Next JS?
We employ the Styled JSX CSS-in-JS library to create encapsulated and scoped styles for styling Next JS components. This ensures that the styles introduced to a component have no impact on other components, enabling seamless addition, modification, and removal of styles without affecting unrelated parts of the application.
12. Is Next JS backend, frontend, or full-stack?
Next JS is considered a full-stack framework, offering the capability to render content on both the client-side and server-side. This feature is particularly valuable in the context of React, as React by itself primarily focuses on frontend development without built-in server-side rendering capabilities.
13. Difference between the pre-rendering types available in Next JS.
HTML is generated at build time.
|
HTML is generated on each request.
|
The pre-generated HTML can be reused on every request.
|
HTML is generated anew for each request.
|
Recommended for performance and efficiency.
|
Suitable for cases where content changes frequently or cannot be determined at build time.
|
Export the page component or use ‘getStaticProps‘
|
Export ‘getServerSideProps‘
|
Less dependent on server resources during runtime.
|
Depends on server resources for generating content dynamically.
|
Typically faster as HTML is pre-generated.
|
Can introduce higher server load due to on-the-fly HTML generation.
|
Easily cache static HTML.
|
Requires server-side caching mechanisms.
|
Scales well as static content can be served efficiently.
|
May require additional server resources to handle dynamic content generation.
|
14. What is client-side rendering, and how does it differ from server-side rendering?
Client-side rendering (CSR) involves rendering a web page on the client’s browser through JavaScript after the initial delivery of HTML, CSS, and JavaScript from the server. The primary distinction between SSR and CSR lies in the fact that SSR transmits a completely rendered HTML page to the client’s browser, whereas CSR delivers an initially empty HTML page that is then populated using JavaScript.
15. How do you pass data between pages in a Next JS application?
Next JS provides several ways to pass data between pages in a Next JS application, including URL query parameters, the Router
API, and state management libraries like Redux or React Context. You can also use the getServerSideProps
function to fetch data on the server and pass it as props to the page component.
Executes on every request.
|
Executes at build time.
|
Used for server-side rendering (SSR).
|
Used for static site generation (SSG).
|
Suitable for pages with frequently changing or dynamic content.
|
Ideal for pages with relatively static content that can be determined at build time.
|
Fetches data on every request, allowing for real-time updates.
|
Fetches data at build time, so the data is static until the next build.
|
Receives a context object containing information about the request.
|
Also receives a context object, but primarily used for dynamic parameters.
|
Handles errors during each request.
|
Errors during data fetching result in a build-time error.
|
Returns an object with a props key.
|
Returns an object with a props key, but may also include a revalidate key for incremental static regeneration.
|
Tends to be slower due to on-the-fly data fetching on each request.
|
Generally faster as data is fetched at build time, reducing server load.
|
The `getStaticPaths` function is employed to create dynamic paths for pages that involve dynamic data. This function is invoked during the build process, allowing the generation of a list of potential values for the dynamic data. The data produced by `getStaticPaths` is subsequently utilized to produce static files for each conceivable value.
18. What is the purpose of the useEffect
hook in React, and how does it relate to Next JS?
The useEffect
hook is used to perform side effects in a functional component, such as fetching data from an API or updating the document title. In Next JS, the useEffect
hook can be used to perform client-side data fetching using the fetch
API or third-party libraries like Axios or SWR.
19. What do you understand by code splitting in Next JS?
In general, code splitting stands out as one of the most compelling features provided by webpack. This functionality allows us to divide our code into multiple bundles, which can be loaded either on-demand or in parallel. Its primary purpose is to create smaller bundles and enables us to manage the prioritization of resource loading, ultimately contributing significantly to improved load times.
There are mainly three approaches to code splitting:
- Entry Points: Employed for manual code splitting by configuring entry points.
- Avoiding Redundancy: Utilizes entry dependencies or the SplitChunksPlugin to deduplicate and divide chunks.
- Dynamic Imports: Achieves code splitting through inline function calls within modules.
Its primary purpose is to facilitate the creation of pages that never load unnecessary code.
20. How do you handle data fetching in Next JS?
In Next.js, data retrieval from an external API or database can be achieved using the built-in functions, namely, `getStaticProps` or `getServerSideProps`. The `getStaticProps` function fetches data during the build process and provides it as props to the page, whereas `getServerSideProps` fetches data for each incoming request. Alternatively, client-side data fetching libraries such as `axios` or `fetch` can also be employed in conjunction with the `useEffect` or `useState` hooks.
21. What are the different options for styling Next JS apps?
Various styling options are available for Next.js applications, ranging from CSS modules and CSS-in-JS libraries like styled-components or emotion to the use of global CSS files.
- CSS modules in Next.js enable the creation of modular CSS that exclusively pertains to specific components. This approach aids in steering clear of naming conflicts and maintains a well-organized structure for your CSS.
- CSS-in-JS libraries like styled-components or emotion offer the ability to compose CSS directly within your JavaScript code. This approach simplifies the process of managing styles for a particular component, integrating styling seamlessly with your component’s logic.
- Global CSS files serve as a means to apply styles that affect the entire application.Each approach has its own advantages and disadvantages, and the best choice depends on the specific needs of your application.
When selecting a styling approach for Next.js applications, it is crucial to take into account factors such as performance, maintainability, and the familiarity of developers with the chosen method.
22. How do you work with custom server middleware in Next JS?
In Next JS, incorporating custom server middleware involves creating a Node.js server. The `use` method of the server object allows the addition of middleware. This can be implemented in the `server.js` file situated in the root directory of the Next JS application. Middleware functions are added using the `app.use` method, providing the capability to modify both incoming requests and outgoing responses.
23. Explain the purpose of the _app.js
file in Next JS.
The `_app.js` file serves as the pivotal component for the entire Next JS application. It provides the flexibility to override the default App component supplied by Next JS, enabling customization of the application’s behavior across all pages. Typically utilized for tasks such as incorporating global styles, persisting layout components, or initializing third-party libraries.
24: How would you implement server-side rendering (SSR) for a Next JS page?
Javascript
import React from 'react' ;
const PageAbout =
({ dataFromServer }) => {
return <div>
{dataFromServer}
</div>;
};
export async function getServerSideProps() {
const dataFromServer = 'Server-rendered data for this page' ;
return {
props: {
dataFromServer,
},
};
}
export default PageAbout;
|
25. Explain the concept of “Serverless” deployment in the context of Next JS. How does it work, and what are the advantages?
Deploying your Next.js application serverlessly involves hosting it on platforms such as Vercel or Netlify. In this setup, there’s no need to manage traditional server infrastructure. These platforms handle server-side rendering, routing, and other aspects automatically, providing advantages such as effortless scaling, cost efficiency, and streamlined deployment.
26. What are some best practices for debugging and testing Next JS applications?
Debugging Next.js applications can be done using browser developer tools, the built-in console API, and third-party debugging tools. For testing, you can use libraries like Jest and React Testing Library to write unit and integration tests. Additionally, use linting tools and the built-in TypeScript or ESLint support to catch code issues early.
27. Why use Create Next App?
create-next-app allows you to swiftly initiate a new Next JS application. Officially maintained by the creators of Next JS, it offers several advantages:
- Interactive Setup: Executing `npx create-next-app@latest` (with no arguments) initiates an interactive experience that guides you through the project setup process.
- Dependency-Free: Project initialization is remarkably fast, taking just a second, as Create Next App comes with zero dependencies.
- Offline Capabilities: Create Next App can automatically detect offline status and bootstrap your project using the local package cache.
- Example Support: It can initialize your application with an example from the Next.js examples collection (e.g., `npx create-next-app –example api-routes`).
- Thorough Testing: As part of the Next.js monorepo, this package undergoes testing with the same integration test suite as Next.js itself. This ensures consistent and expected behavior with every release.
28. What is Image Component and Image Optimization in Next JS?
The Next.js Image component, next/image
, represents a modern evolution of the HTML <img>
element with built-in performance enhancements tailored for the contemporary web.
- Enhanced Performance: Ensures the delivery of appropriately sized images for each device, utilizing modern image formats.
- Visual Stability: Automatically mitigates Cumulative Layout Shift issues to enhance visual stability.
- Expedited Page Loads: Images are loaded dynamically when they come into the viewport, and optional blur-up placeholders can be employed for faster page rendering.
- Asset Flexibility: Supports on-demand image resizing, even for images stored on remote servers, providing flexibility in handling assets.
Next.js is equipped with native support for managing environment variables, providing the following capabilities:
- Utilize .env.local for loading environment variables.
- Expose environment variables to the browser by prefixing them with NEXT_PUBLIC_.
- Default Environment Variables: Typically, only one .env.local file is required. However, there may be instances where you want to establish defaults specifically for the development (next dev) or production (next start) environment.
- Next JS enables you to define defaults in .env (applicable to all environments), .env.development (specific to the development environment), and .env.production (specific to the production environment).
- It’s important to note that .env.local always takes precedence over any defaults that are set.
30. What is Docker Image in Next JS?
Next JS is deployable on hosting providers that offer support for Docker containers. This approach is applicable when deploying to container orchestrators like Kubernetes or HashiCorp Nomad, or when operating within a single node on any cloud provider.
To implement this deployment method:
- Install Docker on your machine.
- Clone the example named with-docker.
- Build your container using the command:
docker build -t nextjs-docker.
- Run your container with the command:
docker run -p 3000:3000 nextjs-docker
31. What is the difference between Next JS and React JS?
The Next JS framework was developed by Vercel.
|
The React front-end library was originated by Facebook.
|
Next JS, an open-source framework based on Node.js and Babel, seamlessly integrates with React to facilitate the development of single-page apps.
|
React, a JavaScript library, empowers the construction of user interfaces through the assembly of components.
|
Next JS, functioning as a framework, leverages React to create both individual UI components and entire web app pages.
|
Within a framework, React JS functions as a library, particularly specializing in the UI components section.
|
Next JS applications exhibit exceptional speed due to static sites and server-side rendering. They are inherently efficient, benefiting from out-of-the-box performance enhancements such as Image Optimization.
|
In the context of React, certain factors make it less suitable for consideration. It inherently supports only client-side rendering by default, a limitation that falls short in constructing a high-performance application.
|
To generate pages for a Next JS project, we include the page in the “pages” folder along with the necessary header component link. This simplification is advantageous as it reduces code volume and enhances project comprehensibility.
|
Initially, we need to craft a component, and subsequently, we incorporate it into the router to formulate a page for a React project.
|
32. How can the data be fetched in Next JS?
We can use multiple methods for fetching data, such as:
- Achieve server-side rendering through the utilization of
getServerSideProps
.
- Implement client-side rendering by employing SWR or utilizing useEffect within React components.
- Utilize
getStaticProps
for static-site rendering, ensuring content generation at build time.
- Enable Incremental Static Regeneration with the use of the ‘revalidate’ prop within
getStaticProps
.
33. Explain the concept of “prefetching” in Next JS and how it impacts performance:
In Next.js, prefetching is a mechanism wherein the framework autonomously initiates the download of JavaScript and assets for linked pages in the background. This proactive approach minimizes navigation time, enhancing the overall user experience with a smoother and faster transition between pages.
34. Can you explain how to internationalize a Next JS application to support multiple languages?
Next.js facilitates internationalization (i18n) through the adoption of libraries such as next-i18next or by developing custom solutions. This process encompasses tasks like translating text and content, managing language-based routing, and implementing a mechanism that allows users to seamlessly switch between languages. Ensuring effective i18n is crucial for ensuring your application is accessible to a diverse global audience.
35. How can you handle cross-origin requests (CORS) in a Next JS application when making API requests to a different domain?
To enable CORS, configure the server or API endpoint receiving your requests. CORS headers may need to be established to permit requests from the domain of your Next.js application. Another option is utilizing serverless functions as proxy endpoints to manage CORS headers effectively.
36. What is serverless architecture, and how does it relate to Next JS?
Serverless architecture is a cloud computing paradigm where the cloud provider takes care of managing the infrastructure, scaling resources automatically based on demand. To leverage serverless architecture with Next.js, one can deploy the application onto serverless platforms such as AWS Lambda or Google Cloud Functions. This approach allows for efficient resource utilization and automatic scaling in response to varying workloads.
37. How do you optimize the performance of a Next JS application?
Optimizing the performance of a Nextjs application involves various strategies such as code splitting, lazy loading, image optimization, server-side caching, and CDN caching. Additionally, leveraging performance monitoring tools like Lighthouse or WebPageTest can help pinpoint areas that require improvement.
38. Explain the purpose of the getServerSideProps
function.
The getServerSideProps
function in Next.js plays a crucial role in achieving server-side rendering (SSR) for dynamic pages. When a user requests a page, this function runs on the server and fetches data dynamically, allowing the page to be pre-rendered with the most up-to-date information.
This function is particularly useful for content that frequently changes or relies on data from external sources. By fetching data on the server side during each request, getServerSideProps
ensures that the content is always fresh, providing a real-time experience to users.
39. What is the purpose of the next.config.js excludes property?
The excludes
property in the next.config.js
file is used to specify patterns for files and directories that should be excluded from the automatic code splitting and bundling performed by Next.js. By defining exclusion patterns, developers can control which files are not subject to the default behavior of code splitting.
Here’s an example of how the excludes
property can be used in next.config.js
:
Javascript
module.exports = {
excludes: [ '/path/to/excluded/file.js' , /\/node_modules\
}
|
40. Explain the purpose of the next.config.js headers property.
The headers
property in the next.config.js
file is used to define custom HTTP headers that should be included in the responses served by your Next.js application. This property allows developers to set various HTTP headers, such as caching policies, security-related headers, and other custom headers, to control how browsers and clients interact with the application.
Here’s an example of how the headers
property can be used:
Javascript
module.exports = {
async headers() {
return [
{
source: '/path/:slug' ,
headers: [
{
key: 'Custom-Header' ,
value: 'Custom-Header-Value' ,
},
{
key: 'Cache-Control' ,
value: 'public, max-age=3600' ,
},
],
},
]
},
}
|
41. What is the purpose of the next.config.js experimental property?
The experimental
property in next.config.js
serves two main purposes in Next.js:
1. Accessing and enabling pre-release features:
- Next.js constantly innovates and introduces new features before being officially released. The
experimental
property provides a safe space to access and experiment with these features before they become stable and widely available.
- You can configure specific flags or options under the
experimental
property to activate these pre-release features in your app. This allows you to test them out, provide feedback, and shape their development before public release.
2. Fine-tuning advanced capabilities:
- Beyond pre-release features, the
experimental
property also offers access to specific configurations aimed at experienced developers who want to further customize and optimize their Next.js applications.
- These configurations might involve low-level optimizations, alternative build mechanisms, or deeper control over internal Next.js behavior. While powerful, they demand a thorough understanding of their impact and potential caveats.
42. What is the purpose of the next.config.js redirects property?
The redirects
property empowers you to establish server-side redirects for incoming requests within your Next.js application. This means you can seamlessly guide users and search engines to different URLs without relying on client-side routing or additional server-side logic.
Key Features:
- Configuration: It’s configured as an asynchronous function that returns an array of redirect objects, each defining a specific redirection rule.
- Server-Side Implementation: Redirects are executed on the server, ensuring a consistent experience across browsers and devices, even with JavaScript disabled.
- Status Codes: You can choose between temporary (307) and permanent (308) redirects based on the intended behavior.
- Conditional Logic: Advanced conditional redirects can be configured based on headers, cookies, query parameters, or other factors, offering granular control over redirection logic.
Javascript
module.exports = {
async redirects() {
return [
{
source: '/old-page' ,
destination: '/new-page' ,
permanent: true ,
},
];
},
};
|
43. What is the purpose of the next.config.js rewrites property?
The rewrites
property offers a powerful mechanism for rewriting incoming request paths to different destination paths within your Next.js application.
Here’s an explanation of the rewrites
property in next.config.js
:
Purpose:
- The
rewrites
property offers a powerful mechanism for rewriting incoming request paths to different destination paths within your Next.js application.
Key Features:
- Configuration: It’s configured as an asynchronous function that returns an array (or object of arrays) of rewrite objects, each defining a specific rewrite rule.
- Server-Side Rewriting: Rewrites occur on the server before the request reaches the client, ensuring full control over routing and content delivery.
- Transparent Redirection: Unlike redirects, rewrites mask the destination path, making it appear as if the user remains on the original URL. This maintains a seamless user experience without visible URL changes.
- Parameter Handling: Query parameters from the original URL can be passed to the destination path, enabling dynamic content fetching and routing.
Javascript
module.exports = {
async rewrites() {
return [
{
source: '/blog/:slug' ,
destination: '/posts/:slug' ,
},
];
},
};
|
44. How can you achieve dynamic route-based code splitting without using getServerSideProps
in Next.js?
Here are two effective ways to achieve dynamic route-based code splitting in Next.js without relying on getServerSideProps
:
1. Dynamic Imports with next/dynamic
:
- Utilize
next/dynamic
to wrap components that you want to load lazily based on route parameters or other conditions.
- When the wrapped component is required for rendering, Next.js automatically fetches its code and dependencies in a separate chunk, reducing initial load time.
Javascript
import dynamic from 'next/dynamic' ;
const BlogPost = dynamic(() => import( '../components/BlogPost' ), {
loading: () => <p>Loading post...</p>,
});
function BlogPage({ postId }) {
return <BlogPost post={postData} />;
}
export default BlogPage;
|
2. Client-Side Rendering (CSR) with Router:
- Employ Next.js’s
router
object within a client-side rendered component to handle navigation and dynamic route loading.
- When a user navigates to a route that hasn’t been loaded yet, the JavaScript code for that route is fetched and executed on the client-side.
Javascript
import { useRouter } from 'next/router' ;
function BlogPage() {
const router = useRouter();
const { postId } = router.query;
return <div>...</div>;
}
export default BlogPage;
|
45. Describe scenarios where you would choose to use getStaticProps
over getServerSideProps
, and vice versa.
Choosing between getStaticProps
and getServerSideProps
depends on several factors in your Next.js application. Here’s a breakdown of scenarios where each method shines:
Choose getStaticProps
when:
- Content is static and rarely changes: Pre-rendering pages at build time with
getStaticProps
delivers lightning-fast performance and optimal SEO, as search engines can readily crawl and index the content.
- Scalability and cost-effectiveness: Since page generation happens at build time, no server requests are needed on every visit, improving server performance and reducing costs.
- Improved user experience: Pages load instantly as they’re already pre-rendered, leading to a smooth and responsive user experience, especially for initial visits.
Choose getServerSideProps
when:
- Dynamic content that frequently updates: Use
getServerSideProps
to fetch data and pre-render pages at request time when content changes frequently, like news articles, stock prices, or personalized user data.
- User authentication and personalization: Access user-specific data like shopping carts or logged-in states, personalize UI elements, and implement authentication logic dynamically based on user requests.
- API data integration: For real-time data from external APIs or databases,
getServerSideProps
allows fetching and integrating data directly into page responses during server-side rendering.
46. Explain the purpose of the next export
command. When would you use it, and what are its limitations?
As of Next.js version 12.2, the next export
command has been deprecated and removed in favour of configuring static exports within the next.config.js
file. However, understanding its previous purpose and limitations can still be relevant for older projects or migrating to the new approach.
Purpose:
next export
used to generate a static version of your Next.js application, meaning all pages and their corresponding HTML, CSS, and JavaScript files were pre-rendered and saved to a static folder (/out
).
- This static directory could then be hosted on any web server that serves static assets, eliminating the need for a Node.js server to run Next.js at runtime.
Use cases:
- Offering faster deployment times and lower server costs compared to server-side rendering.
- Improved search engine optimization (SEO)
- Faster initial page load
Limitations:
- Dynamic content limitations
- Higher build times
- Limited flexibility
Current approach:
With the next export
command gone, static site generation is now configured within the next.config.js
file through the output: export
option. This option offers more flexibility and control over static exports, allowing you to fine-tune which pages or routes to pre-render and define custom configurations.
Remember, static exports are ideal for primarily static websites where performance and SEO are crucial. But for applications with significant dynamic content or server-side logic, server-side rendering might be a better choice. Weighing your specific needs and priorities will help you determine the best approach for your Next.js application.
47. What is the significance of the _error.js
and 404.js
files in the pages
directory, and how can they be customized for error handling in Next.js?
Here’s an explanation of the _error.js
and 404.js
files in Next.js, along with how to customize them for effective error handling:
_error.js:
Purpose:
- Serves as a catch-all mechanism for handling unhandled errors that occur during rendering or runtime in your Next.js application.
- It’s a special file within the
pages
directory that Next.js automatically invokes when errors arise.
Customization:
- Create a custom
_error.js
file to render a user-friendly error page instead of the default stack trace.
- Access and display relevant error information within the component:
statusCode
: The HTTP status code of the error.
error
: The error object itself.
Example: Below is the code example of the _error.js.
Javascript
import React from 'react' ;
export default
function Error({ statusCode }) {
return (
<div>
<h1>Something went wrong!</h1>
<p>
We're working on it.
Please try again later.
</p>
{statusCode !== 404 && (
<p>Status Code: {statusCode}</p>
)}
</div>
);
}
|
404.js:
Purpose:
- Handles 404 Not Found errors specifically, providing a tailored experience when users try to access non-existent pages.
Customization:
- Create a custom
404.js
file to render a more informative or visually appealing 404 page.
- Optionally, implement custom logic to redirect users to relevant pages or handle 404 errors differently.
Example: Below is the code example of the 404.js:
Javascript
import React from 'react' ;
export default
function NotFound() {
return (
<div>
<h1>Page Not Found</h1>
<p>
Sorry, the page you 're
looking for doesn' t exist.
</p>
<p>
Try searching for what you need,
or go back to the
<a href= "/" >homepage</a>.
</p>
</div>
);
}
|
48. How can you implement conditional redirects in Next.js based on certain criteria, such as user authentication status or role?
Here are several methods to implement conditional redirects in Next.js based on criteria like authentication status or user roles:
Here are several methods to implement conditional redirects in Next.js based on criteria like authentication status or user roles:
1. Redirects in getServerSideProps
or getStaticProps
:
- Check conditions within these functions and initiate redirects using
res.writeHead()
and res.end()
:
Javascript
export async function getServerSideProps(context) {
const isAuthenticated =
await checkAuth(context.req);
if (
!isAuthenticated &&
context.resolvedUrl !== '/login'
){
context.res
.writeHead(302, { Location: '/login' });
context.res.end();
return { props: {} };
}
}
|
2. Client-Side Redirects with useEffect
and router.push
:
- Execute redirects on the client-side after component rendering:
Javascript
import { useEffect } from 'react' ;
import { useRouter } from 'next/router' ;
function MyPage() {
const router = useRouter();
useEffect(
() => {
const isAuthenticated = checkAuth();
if (!isAuthenticated) {
router.push( '/login' );
}
}, []);
}
|
49. Explain the purpose of the publicRuntimeConfig
and serverRuntimeConfig
options in Next.js. How do they differ from regular environment variables?
Next.js provides two distinct options for configuring your application: publicRuntimeConfig
and serverRuntimeConfig
. They differ from regular environment variables in terms of accessibility and security. Let’s explore each option:
1. publicRuntimeConfig:
- Purpose: Stores configuration values accessible both on the client and server-side. Ideal for settings like API endpoints, base URLs, or theme information.
- Accessibility: Values are serialized into the built JavaScript bundle during server-side rendering. This means they are easily accessible within any component on the client-side.
2. serverRuntimeConfig:
- Purpose: Stores configuration values accessible only on the server-side.
- Security: Ideal for storing sensitive information as it is never exposed to the client.
3. Differences from Environment Variables:
- Environment variables: Set at the system or deployment level and accessible at runtime in both server and client-side processes.
publicRuntimeConfig
: Offers controlled client-side access without needing environment variables.
50. How can you implement custom error boundaries in a Next.js project to gracefully handle errors and prevent the entire application from crashing?
Here’s how to implement custom error boundaries in Next.js to gracefully handle errors and enhance application resilience:
1. Create a Custom Error Boundary Component:
- Define a class component that extends React’s
React.Component
class.
- Implement the
static getDerivedStateFromError(error)
lifecycle method to capture errors and update the component’s state.
- Render a fallback UI in the
render()
method when errors occur, preventing the entire application from crashing.
Javascript
import React from 'react' ;
class ErrorBoundary extends React.Component {
constructor(props) {
super (props);
this .state = { hasError: false };
}
static getDerivedStateFromError(error) {
return { hasError: true };
}
componentDidCatch(error, errorInfo) {
}
render() {
if ( this .state.hasError) {
return <h1>Something went wrong.</h1>;
}
return this .props.children;
}
}
|
2. Wrap Components with the Error Boundary:
- Use the
<ErrorBoundary>
component as a wrapper around any components or sections of your application you want to protect.
Javascript
<ErrorBoundary>
<MyComponent />
</ErrorBoundary>
|
Key Points:
- Error Handling: Error boundaries catch both JavaScript errors (e.g., syntax errors, runtime exceptions) and React errors (e.g., errors thrown during rendering).
- Error Propagation: Unhandled errors within the error boundary itself propagate up to the nearest parent error boundary, creating a hierarchy for error handling.
Share your thoughts in the comments
Please Login to comment...