Next.js Reducing JavaScript Size
Last Updated :
06 Jun, 2023
Next.js is a React-based framework that helps build scalable web apps with optimized JavaScript bundles for better performance. Code splitting breaks down large JavaScript files into smaller modules, allowing developers to load only the necessary code for a specific page or feature, which improves performance by reducing the amount of data that needs to be processed by the browser.
Next.js offers built-in code splitting, allowing developers to easily break down and load smaller portions of code on demand. This can enhance app speed and user responsiveness, especially on slower networks and older devices, resulting in a better user experience
Prerequisites:
Â
Steps to Create the Next Application:
Step 1: Create the next application by using this command
npx create-next-app code-splitting
Step 2: After creating your project folder, i.e. code-splitting, use the following command to navigate to it:
cd code-splitting
Project Structure: It will look as follows:
.png)
Â
Example: In this example, We use code splitting and the next/link module’s client-side navigation to load the Hello component dynamically when the user clicks a link without reloading the whole page. Using code splitting to dynamically import the Hello component reduces the JavaScript bundle size and improves app performance.
Step 3: Create index.js and about.js in the pages directory.
Javascript
import Link from "next/link" ;
export default function Index() {
const linkStyle = {
backgroundColor: "green" ,
color: "white" ,
fontSize: "1rem" ,
padding: "0.5rem 1rem" ,
borderRadius: "4px" ,
border: "none" ,
cursor: "pointer" ,
};
return (
<div style={{ margin: "2rem" }}>
{
}
<Link href= "/hello" style={linkStyle}>
Click Me!
</Link>
</div>
);
}
|
Javascript
import Link from "next/link" ;
export default function About() {
const linkStyle = {
backgroundColor: "green" ,
color: "white" ,
fontSize: "1rem" ,
padding: "0.5rem 1rem" ,
borderRadius: "4px" ,
border: "none" ,
cursor: "pointer" ,
};
return (
<div style={{ margin: "2rem" }}>
{
}
<Link href= "/hello" style={linkStyle}>
Click This!
</Link>
</div>
);
}
|
CSS
html,
body {
padding : 0 ;
margin : 0 ;
font-family : -apple-system,
BlinkMacSystemFont, Segoe UI,
Roboto, Oxygen, Ubuntu,
Cantarell, Fira Sans, Droid Sans,
Helvetica Neue, sans-serif ;
}
a {
color : inherit;
text-decoration : none ;
}
* {
box-sizing: border-box;
}
@media (prefers-color-scheme: dark) {
html {
color-scheme: dark;
}
body {
color : white ;
background : black ;
}
}
|
Step 4: Create a “components/Hello.js” module exporting a React component.
Javascript
import React from 'react' ;
export default function Hello({ name }) {
return <div> <h1>Welcome To {name}! </h1> </div>;
}
|
Step 5: Create a “pages/hello.js” page with the dynamic import of the Hello component.
Javascript
import dynamic from "next/dynamic" ;
const Hello = dynamic(
() => import( "../components/Hello" )
.then((mod) => mod. default ), {
ssr: false ,
}
);
export default function HelloPage() {
return <Hello name= "GeeksforGeeks" />;
}
|
Step 6: To run the application, open the Terminal and enter the command listed below. Go to http://localhost:3000/ to see the output.
npm run dev
Output:
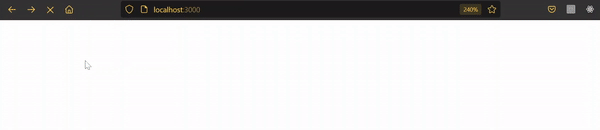
Â
Conclusion: In conclusion, Next.js offers an easy and efficient way to reduce the size of your JavaScript bundle by using code splitting. By implementing this feature, you can improve the performance and loading speed of your web application, resulting in a better user experience.
Share your thoughts in the comments
Please Login to comment...