Next.js Styling: Sass
Last Updated :
01 Apr, 2024
NextJS is a React framework that is used to build full-stack web applications. It is used both for front-end as well and back-end. It simplifies React development with powerful features.
In this article, we will learn How to use Sass with next.js
How to use Sass with Next.js ?
Sass stands for Syntactically Awesome Style Sheets. It is a powerful preprocessor scripting language that extends CSS with features like variables, nesting, mixins, and more. It follows a structured approach to writing stylesheets that makes CSS code more maintainable and scalable.
To use Sass in next.js, You have to install it using the following command and create a file with the extension of .scss (ex, global.scss)
npm install --save-dev sass
Syntax:
// Navigation bar styles
.navigation {
background-color: #333;
padding: 1rem;
// List styles
ul {
list-style-type: none;
margin: 0;
padding: 0;
// List item styles
li {
display: inline-block;
margin-right: 1rem;
// Anchor styles
a {
color: white;
text-decoration: none;
padding: 0.5rem 1rem;
&:hover {
text-decoration: underline;
}
}
}
}
}
Steps to Create NextJS Application:
Step 1: Create a Next.js application using the following command.
npx create-next-app@latest gfg
Step 2: It will ask you some questions, so choose as the following.
√ Would you like to use TypeScript? ... No
√ Would you like to use ESLint? ... Yes
√ Would you like to use Tailwind CSS? ... No
√ Would you like to use `src/` directory? ... Yes
√ Would you like to use App Router? (recommended) ... Yes
√ Would you like to customize the default import alias (@/*)? ... Yes
Step 3: After creating your project folder i.e. gfg, move to it using the following command.
cd gfg
Step 4: Install the Sass module.
npm install --save-dev sass
The updated dependencies in package.json will look like this:
"dependencies": {
"next": "14.1.4",
"react": "^18",
"react-dom": "^18",
"sass": "^1.72.0"
}
Project Structure:
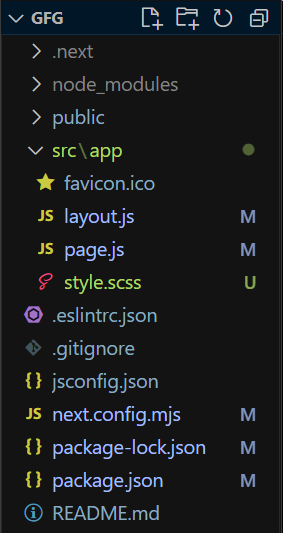
Example: The below example demonstrates the use of Sass in Next.js
CSS
/* File path: src/app/style.scss */
:root {
--primary-color: rgb(6, 6, 92);
}
h1 {
color: green;
}
.container {
display: flex;
align-items: center;
padding: 0px 5px;
gap: 10px;
.box {
height: 150px;
width: 150px;
background-color: var(--primary-color);
}
.btnGroup {
display: flex;
gap: 5px;
button {
padding: 8px 15px;
border: none;
border-radius: 5px;
color: white;
font-weight: bold;
}
button:hover {
background-color: rgba(0, 0, 0, 0.977) !important;
}
#red {
background-color: red;
}
#green {
background-color: green;
}
#blue {
background-color: blue;
}
}
}
JavaScript
//File path: src/app/page.js
'use client';
import { useRef } from "react";
export default function Home() {
const box = useRef(null)
const ChangeColor = (color) => {
box.current.style.backgroundColor = color;
};
return (
<>
<h1>GeeksForGeeks | Sass in Next.js </h1>
<div className="container">
<div className="box" ref={box}></div>
<div className="btnGroup">
<button id="red"
onClick={() => ChangeColor('red')}>
Red
</button>
<button id="green" className="green"
onClick={() => ChangeColor('green')}>
Green
</button>
<button id="blue" className="blue"
onClick={() => ChangeColor('blue')}>
Blue
</button>
</div>
</div>
</>
);
}
To run the Application open the terminal in the project folder and enter the following command:
npm run dev
Output:
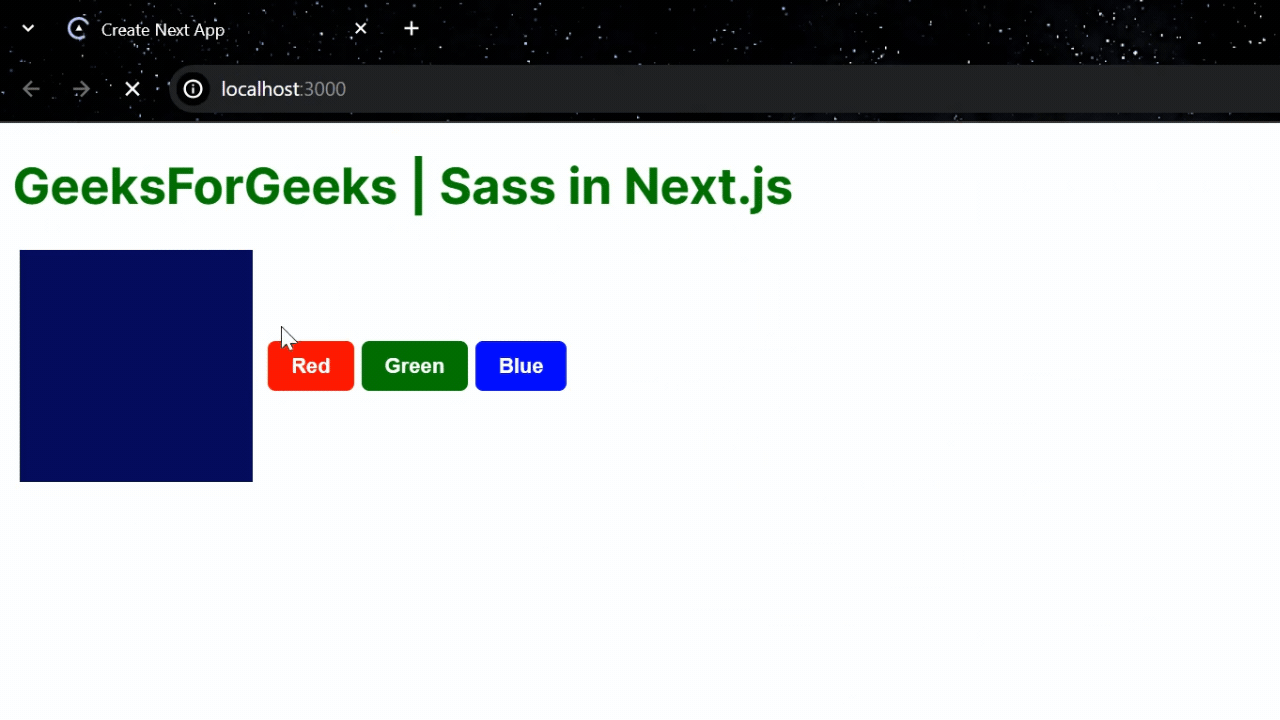
Share your thoughts in the comments
Please Login to comment...