ReactJS Pure Components
Last Updated :
14 Mar, 2024
Generally, In ReactJS, we use the shouldComponentUpdate() Lifecycle method to customize the default behavior and implement it when the React component should re-render or update itself.
When working with React pure components, we can utilize their behavior to optimize behavior as pure components automatically handle the shouldComponentUpdate() method and we don’t need to explicitly implement it.
In this article, we will learn what is Pure component in React, and its key points with examples.
Prerequisites
What are Pure Components in React
ReactJS has provided us with a Pure Component. If we extend a class with Pure Component, there is no need for the shouldComponentUpdate() lifecycle method. ReactJS Pure Component Class compares the current state and props with new props and states to decide whether the React component should re-render itself or not.
In simple words, If the previous value of the state or props and the new value of the state or props are the same, the component will not re-render itself. Pure Components restricts the re-rendering when there is no use for re-rendering of the component. Pure Components are Class Components that extend React.PureComponent.
Example
This example demonstrates the creation of Pure Components.
Javascript
import React from "react";
export default class Test extends React.PureComponent {
render() {
return <h1>Welcome to GeeksforGeeks</h1>;
}
}
Output :
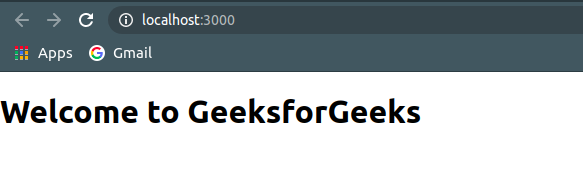
Pure Components Key Points
Some key points to remember about Pure Components are:
Shallow Comparison:
- Pure components perform a shallow comparison of the props and states. If the objects are passed as props or states have the same references, a re-render is prevented.
Performance Optimization:
- Pure components can provide performance optimizations by preventing unnecessary re-renders when the data is the same and hasn’t been modified.
ShouldComponentUpdate:
- Pure components automatically implement the shouldComponentUpdate() method with a shallow prop and state comparison. This method returns false if the props and state haven’t changed.
Pure Components Advantage
Extending React Class Components with Pure Components ensures the higher performance of the Component and ultimately makes your application faster.
While Regular Components will always re-render when the value of state and props changes, in Pure Components the value of State and Props, are Shallow Compared (Shallow Comparison) and it also takes care of “shouldComponentUpdate” Lifecycle method implicitly.
Pure components also simplify the code and provide easy integrations. To convert a regular component to a pure component, change the base class from React.Component to React.PureComponent.
Important Considerations
There is also a possibility that these State and Props objects contain nested data structure then Pure Component’s implemented shouldComponentUpdate will return false and will not update the whole subtree of Children of this Class Component. So in Pure Component, the nested data structure doesn’t work properly.
In this case, State and Props Objects should be simple objects and Child Elements should also be Pure, which means to return the same output for the same input values at any instance.
Also, it is important to use immutable data for props and states to ensure the proper functioning of pure components.
Conclusion
Pure components improve the performance and speed in React by avoiding unnecessary re-renders. They perform shallow comparisons of props and state, resulting in improved performance and simplified code writing.
Share your thoughts in the comments
Please Login to comment...