React lifecycle starts from its initialization and ends when it is unmounted from the DOM. There are several methods defined for different phases of the lifecycle of React Components.
React Lifecycle
React Lifecycle is defined as the series of methods that are invoked in different stages of the component’s existence. The definition is pretty straightforward but what do we mean by different stages? A React Component can go through four stages of its life as follows.
Lifecycle of React Components:
Each React Component go though the given Phases.
1. Initialization phase
This is the stage where the component is constructed with the given Props and default state. This is done in the constructor of a Component Class.
2. Mounting Phase
Mounting is the stage of rendering the JSX returned by the render method itself.
3. Updating
Updating is the stage when the state of a component is updated and the application is repainted.
4. Unmounting
As the name suggests Unmounting is the final step of the component lifecycle where the component is removed from the page.
React provides the developers with a set of predefined functions that if present are invoked around specific events in the lifetime of the component. Developers are supposed to override the functions with the desired logic to execute accordingly. We have illustrated the gist in the following diagram.
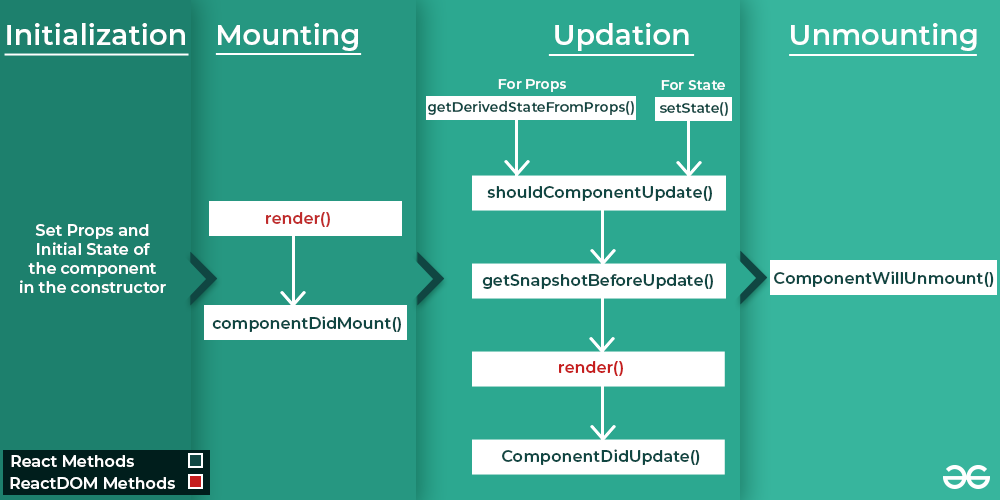
Now let us describe each phase and its corresponding functions.
Phases of Lifecycle in React Components
1. Initialization
In this phase, the developer has to define the props and initial state of the component this is generally done in the constructor of the component. The following code snippet describes the initialization process.
class Clock extends React.Component {
constructor(props)
{
// Calling the constructor of
// Parent Class React.Component
super(props);
// Setting the initial state
this.state = { date : new Date() };
}
}
2. Mounting
Mounting is the phase of the component lifecycle when the initialization of the component is completed and the component is mounted on the DOM and rendered for the first time on the webpage. Now React follows a default procedure in the Naming Conventions of these predefined functions where the functions containing “Will” represents before some specific phase and “Did” represents after the completion of that phase. The mounting phase consists of two such predefined functions as described below.
- constructor
- static getDerivedStateProps
- render()
- componentDidMount()
Method to initialize state and bind methods. Executed before the component is mounted.
constructor example:
Javascript
import React from "react" ;
import ReactDOM from "react-dom/client" ;
class Test extends React.Component {
constructor(props) {
super (props);
this .state = { hello: "World!" };
}
render() {
return (
<div>
<h1>
GeeksForGeeks.org, Hello
{ this .state.hello}
</h1>
</div>
);
}
}
const root = ReactDOM.createRoot(
document.getElementById( "root" )
);
root.render(<Test />);
|
Used for updating the state based on props. Executed before every render.
Example:
Javascript
import React from "react" ;
import ReactDOM from "react-dom/client" ;
class Test extends React.Component {
constructor(props) {
super (props);
this .state = { hello: "World!" };
}
static getDerivedStateFromProps(props, state) {
return { hello: props.greet };
}
render() {
return (
<div>
<h1>
GeeksForGeeks.org, Hello
{ this .state.hello}
</h1>
</div>
);
}
}
const root = ReactDOM.createRoot(
document.getElementById( "root" )
);
root.render(<Test greet= "Geeks!" />);
|
Responsible for rendering JSX and updating the DOM.
render() Example:
Javascript
import React from "react" ;
import ReactDOM from "react-dom/client" ;
class Test extends React.Component {
render() {
return (
<div>
<h1>
GeeksforGeeks
</h1>
</div>
);
}
}
const root = ReactDOM.createRoot(
document.getElementById( "root" )
);
root.render(<Test />);
|
This function is invoked right after the component is mounted on the DOM i.e. this function gets invoked once after the render() function is executed for the first time
componentDidMount() Example:
Javascript
import React from "react" ;
import ReactDOM from "react-dom/client" ;
class Test extends React.Component {
constructor(props) {
super (props);
this .state = { hello: "World!" };
}
componentDidMount() {
this .setState({hello: "Geeks!" })
}
render() {
return (
<div>
<h1>
GeeksForGeeks.org, Hello
{ this .state.hello}
</h1>
</div>
);
}
}
const root = ReactDOM.createRoot(
document.getElementById( "root" )
);
root.render(<Test />);
|
3. Updation
React is a JS library that helps create Active web pages easily. Now active web pages are specific pages that behave according to their user. For example, let’s take the GeeksforGeeks {IDE} webpage, the webpage acts differently with each user. User A might write some code in C in the Light Theme while another User may write Python code in the Dark Theme all at the same time. This dynamic behavior that partially depends upon the user itself makes the webpage an Active webpage. Now how can this be related to Updation? Updation is the phase where the states and props of a component are updated followed by some user events such as clicking, pressing a key on the keyboard, etc. The following are the descriptions of functions that are invoked at different points of the Updation phase.
- getDerivedStateFromProps
- setState() Function
- shouldComponentUpdate()
- getSnapshotBeforeUpdate() Method
- componentDidUpdate()
getDerivedStateFromProps(props, state) is a static method that is called just before render() method in both mounting and updating phase in React. It takes updated props and the current state as arguments.
static getDerivedStateFromProps(props, state) {
if(props.name !== state.name){
//Change in props
return{
name: props.name
};
}
return null; // No change to state
}
This is not particularly a Lifecycle function and can be invoked explicitly at any instant. This function is used to update the state of a component. You may refer to this article for detailed information.
this.setState((prevState, props) => ({
counter: prevState.count + props.diff
}));
setState Example:
Javascript
import React from "react" ;
import ReactDOM from "react-dom/client" ;
class App extends React.Component {
constructor(props) {
super (props);
this .state = {
count: 0,
};
}
increment = () => {
this .setState((prevState) => ({
count: prevState.count + 1,
}));
};
decrement = () => {
this .setState((prevState) => ({
count: prevState.count - 1,
}));
};
render() {
return (
<div>
<h1>
The current count is :{ " " }
{ this .state.count}
</h1>
<button onClick={ this .increment}>
Increase
</button>
<button onClick={ this .decrement}>
Decrease
</button>
</div>
);
}
}
const root = ReactDOM.createRoot(
document.getElementById( "root" )
);
root.render(
<React.StrictMode>
<App />
</React.StrictMode>
);
|
By default, every state or props update re-renders the page but this may not always be the desired outcome, sometimes it is desired that updating the page will not be repainted. The shouldComponentUpdate() Function fulfills the requirement by letting React know whether the component’s output will be affected by the update or not. shouldComponentUpdate() is invoked before rendering an already mounted component when new props or states are being received. If returned false then the subsequent steps of rendering will not be carried out. This function can’t be used in the case of forceUpdate(). The Function takes the new Props and new State as the arguments and returns whether to re-render or not.
shouldComponentUpdate(nextProps, nextState)
It returns true or false, if false then render(), componentWillUpdate() and componentDidUpdate() method does not gets invoked.
The getSnapshotBeforeUpdate() method is invoked just before the DOM is being rendered. It is used to store the previous values of the state after the DOM is updated.
getSnapshotBeforeUpdate(prevProps, prevState)
Similarly this function is invoked after the component is rerendered i.e. this function gets invoked once after the render() function is executed after the updation of State or Props.
componentDidUpdate(prevProps, prevState, snapshot)
4. Unmounting
This is the final phase of the lifecycle of the component which is the phase of unmounting the component from the DOM. The following function is the sole member of this phase.
This function is invoked before the component is finally unmounted from the DOM i.e. this function gets invoked once before the component is removed from the page and this denotes the end of the lifecycle.
componentWillUnmount() Example:
Javascript
import React from "react" ;
class ComponentOne extends React.Component {
componentWillUnmount() {
alert( "The component is going to be unmounted" );
}
render() {
return <h1>Hello Geeks!</h1>;
}
}
class App extends React.Component {
state = { display: true };
delete = () => {
this .setState({ display: false });
};
render() {
let comp;
if ( this .state.display) {
comp = <ComponentOne />;
}
return (
<div>
{comp}
<button onClick={ this . delete }>
Delete the component
</button>
</div>
);
}
}
export default App;
|
We have so far discussed every predefined function there was in the lifecycle of the component, and we have also specified the order of execution of the function.
Implementing the Component Lifecycle methods
Let us now see one final example to finish the article while revising what’s discussed above.
First, create a react app and edit your index.js file from the src folder.
Javascript
import React from "react" ;
import ReactDOM from "react-dom/client" ;
class Test extends React.Component {
constructor(props) {
super (props);
this .state = { hello: "World!" };
}
componentDidMount() {
console.log( "componentDidMount()" );
}
changeState() {
this .setState({ hello: "Geek!" });
}
render() {
return (
<div>
<h1>
GeeksForGeeks.org, Hello
{ this .state.hello}
</h1>
<h2>
<a
onClick={ this .changeState.bind(
this
)}
>
Press Here!
</a>
</h2>
</div>
);
}
shouldComponentUpdate(nextProps, nextState) {
console.log( "shouldComponentUpdate()" );
return true ;
}
componentDidUpdate() {
console.log( "componentDidUpdate()" );
}
}
const root = ReactDOM.createRoot(
document.getElementById( "root" )
);
root.render(<Test />);
|
Output: This output will be visible on the http://localhost:3000 on the browser window.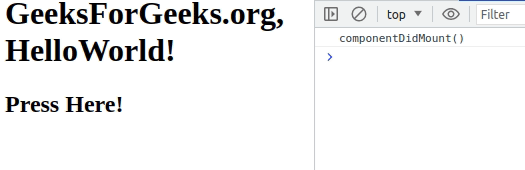
Share your thoughts in the comments
Please Login to comment...