Explain the concept of memoization in pure components.
Last Updated :
20 Feb, 2024
Memoization in React caches results in expensive function calls to save re-computation time. It’s implemented via useMemo and React.memo, which memorize function outputs and component results respectively. This optimizes pure components, updating only when props or state change, boosting performance.
Concept of memoization in pure components:
- Memoization in programming optimizes function execution time by caching results for specific input parameters.
- React’s PureComponent automatically performs shallow comparisons of state and props data for efficient rendering.
- For deeper comparisons, memoization techniques can be employed.
- Memoization contributes to substantial time and resource savings in large-scale applications, enhancing efficiency and speed.
Key Features of memoization in pure components:
- Performance Optimization: Increases performance by reducing unnecessary re-rendering of components.
- Memoization: Memorizes the previous output of a function and reuses it without re-computing.
- Computational Heavy Task Optimization: Helps in managing and optimizing computational heavy tasks in React components.
Steps to Create React Application And Installing Module:
Step 1:Â Create a React application using the following command:
npx create-react-app foldername
Step 2: After creating your project folder i.e. foldername, move to it using the following command:
cd foldername
Step 3: Install the necessary package in your application using the following command.
npm install memoize-one
Example: Let’s take a simple example of a component that calculates the factorial of a number:
In this memoized version, the factorial function gets called only when the this.props.number value changes. If the component re-renders with the same props value, the factorial will not be calculated again; instead, the memoized result will be returned, enhancing the performance.
Javascript
import React from 'react' ;
import memoize from 'memoize-one' ;
class Factorial extends React.Component {
calculateFactorial = memoize(number => {
let fact = 1;
for (let i = 2; i <= number; i++) {
fact = fact * i;
}
return fact;
});
render() {
return (
<div>
{`Factorial of
${ this .props.number} is
${ this .calculateFactorial( this .props.number)}`}
</div>
);
}
}
export default Factorial;
|
Javascript
import React from 'react' ;
import Factorial from './Factorial' ;
class App extends React.Component {
state = { number: 5 };
increaseNumber = () => {
this .setState(prevState => ({
number: prevState.number + 1
}));
};
render() {
return (
<div>
<Factorial number={ this .state.number} />
<button
onClick={ this .increaseNumber}>
Increase Number
</button>
</div>
);
}
}
export default App;
|
Start your application using the following command.
npm start
Output:
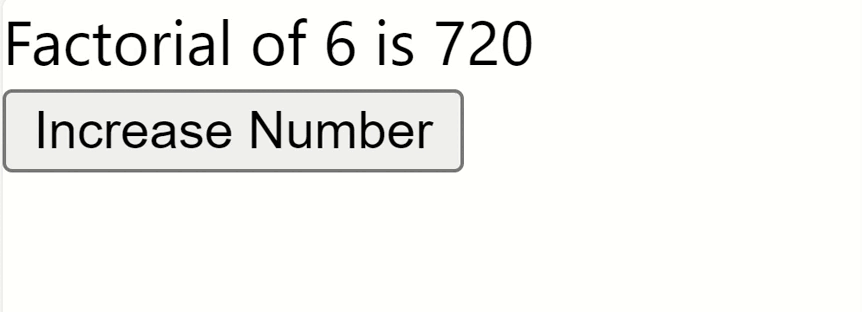
Output
Share your thoughts in the comments
Please Login to comment...