How to create a Popup Form using Vue.js & Tailwind CSS ?
Last Updated :
24 Apr, 2024
We’ll create a simple popup form using Vue.js and Tailwind CSS. The form will allow users to input their email address and password. A button will open the form in the popup modal and users can submit the form or close it.
Prerequisites
Approach
We’ll create a popup form using Vue.js. The form will include fields for the email address and password and users can open and close the form using the button.
- Create a button to open the popup form.
- Implement a popup modal with the fields for the email address and password.
- Use Vue.js to manage the form’s visibility.
- Allow users to submit the form or close it.
- Style the form using the Tailwind CSS for a modern and responsive design.
Example: This example shows the implementation of the above-explained approach.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width,
initial-scale=1.0">
<title>Popup Form</title>
<link href=
"https://cdn.jsdelivr.net/npm/tailwindcss@2.2.19/dist/tailwind.min.css"
rel="stylesheet">
</head>
<body class="bg-blue-200 h-screen flex
justify-center items-center">
<div id="app">
<button @click="openPopup"
class="bg-blue-800 hover:bg-blue-600
text-white font-bold py-2 px-4
rounded transition duration-300
ease-in-out">Open
Form</button>
<div v-if="showPopup"
class="fixed inset-0 flex items-center
justify-center bg-gray-900
bg-opacity-50 transition-opacity
duration-300">
<div class="bg-white p-8 rounded shadow-md
max-w-md transform
transition-transform duration-300">
<h1 class="text-2xl font-semibold mb-4">
Popup Form</h1>
<form>
<div class="mb-4">
<label for="email"
class="block text-gray-700
font-bold mb-2">Email Address:</label>
<input type="email" id="email"
name="email"
class="w-full border border-gray-300
rounded px-3 py-2">
</div>
<div class="mb-4">
<label for="password"
class="block text-gray-700
font-bold mb-2">Password:</label>
<input type="password" id="password"
name="password"
class="w-full border border-gray-300
rounded px-3 py-2">
</div>
<button type="submit"
class="bg-green-500 hover:bg-green-600
text-white font-bold py-2 px-4
rounded transition duration-300
ease-in-out">Submit</button>
<button type="button" @click="closePopup"
class="bg-red-500 hover:bg-red-600
text-white font-bold py-2 px-4
rounded transition duration-300
ease-in-out ml-4">Close</button>
</form>
</div>
</div>
</div>
<script src=
"https://cdn.jsdelivr.net/npm/vue@2.6.14/dist/vue.js"></script>
<script>
new Vue({
el: '#app',
data: {
showPopup: false
},
methods: {
openPopup() {
this.showPopup = true;
},
closePopup() {
this.showPopup = false;
}
}
});
</script>
</body>
</html>
Output:
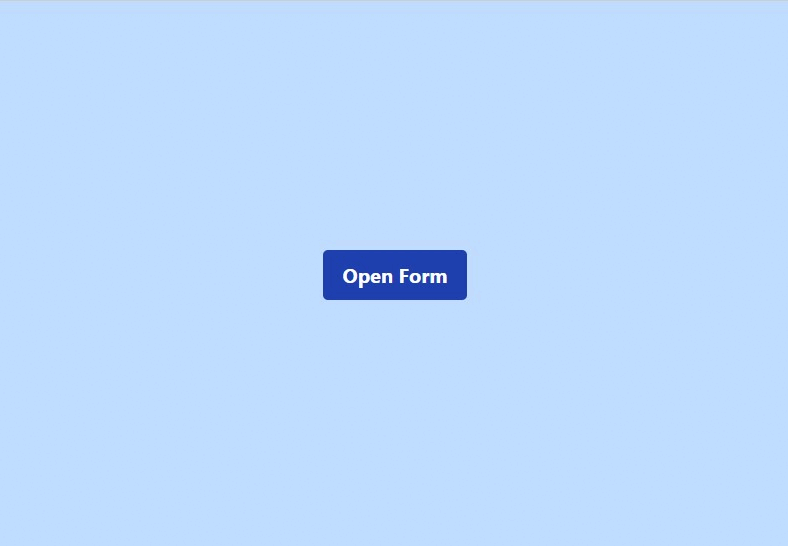
Share your thoughts in the comments
Please Login to comment...