ReactJS Keys
Last Updated :
24 Nov, 2023
React JS keys are a way of providing a unique identity to each item while creating the React JS Lists so that React can identify the element to be processed.
What is a key in React ?
A “key” is a special string attribute you need to include when creating lists of elements in React. Keys are used in React to identify which items in the list are changed, updated, or deleted.
Keys are used to give an identity to the elements in the lists. It is recommended to use a string as a key that uniquely identifies the items in the list.
Assigning keys to the list
You can assign the array indexes as keys to the list items. The below example assigns array indexes as keys to the elements.
Syntax:
const numbers = [1, 2, 3, 4, 5];
const updatedNums = numbers.map((number, index) =>
<li key={index}>
{number}
</li>
);
Issue with index as keys
Assigning indexes as keys is highly discouraged because if the elements of the arrays get reordered in the future then it will get confusing for the developer as the keys for the elements will also change.
Difference between keys and props in React
Keys are not the same as props, only the method of assigning “key” to a component is the same as that of props. Keys are internal to React and can not be accessed from inside of the component like props. Therefore, we can use the same value we have assigned to the Key for any other prop we are passing to the Component.
Using Keys with Components :
Consider a situation where you have created a separate component for list items and you are extracting list items from that component. In that case, you will have to assign keys to the component you are returning from the iterator and not to the list items. That is you should assign keys to <Component /> and not to <li> A good practice to avoid mistakes is to keep in mind that anything you are returning from inside of the map() function is needed to be assigned a key.
Incorrect usage of keys:
Example 1: The below code shows the incorrect usage of keys:
Javascript
import React from "react" ;
import ReactDOM from "react-dom/client" ;
function MenuItems(props) {
const item = props.item;
return <li key={item.toString()}>{item}</li>;
}
function Navmenu(props) {
const list = props.menuitems;
const updatedList = list.map((listItems) => {
return <MenuItems item={listItems} />;
});
return <ul>{updatedList}</ul>;
}
const menuItems = [1, 2, 3, 4, 5];
const root = ReactDOM.createRoot(document.getElementById( 'root' ));
root.render(
<React.StrictMode>
<Navmenu menuitems={menuItems} />
</React.StrictMode>
);
|
Output: You can see in the below output that the list is rendered successfully but a warning is thrown to the console that the elements inside the iterator are not assigned keys. This is because we had not assigned the key to the elements we are returning to the map() iterator.

Correct usage of keys:
Example 2: The below example shows the correct usage of keys.
Javascript
import React from "react" ;
import ReactDOM from "react-dom" ;
function MenuItems(props) {
const item = props.item;
return <li>{item}</li>;
}
function Navmenu(props) {
const list = props.menuitems;
const updatedList = list.map((listItems) => {
return <MenuItems key={listItems.toString()} item={listItems} />;
});
return <ul>{updatedList}</ul>;
}
const menuItems = [1, 2, 3, 4, 5];
ReactDOM.render(
<Navmenu menuitems={menuItems} />,
document.getElementById( "root" )
);
|
Output: In the below-given output you can clearly see that this time the output is rendered but without any type of warning in the console, it is the correct way to use React Keys.
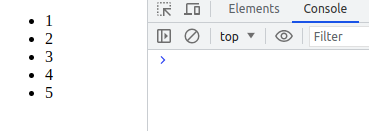
Uniqueness of Keys:
We have told many times while discussing keys that keys assigned to the array elements must be unique. By this, we did not mean that the keys should be globally unique. All the elements in a particular array should have unique keys. That is, two different arrays can have the same set of keys.
Example 3: In the below code, we have created two different arrays menuItems1 and menuItems2. You can see in the below code that the keys for the first 5 items for both arrays are the same still the code runs successfully without any warning.
Javascript
import React from "react" ;
import ReactDOM from "react-dom" ;
function MenuItems(props) {
const item = props.item;
return <li>{item}</li>;
}
function Navmenu(props) {
const list = props.menuitems;
const updatedList = list.map((listItems) => {
return <MenuItems key={listItems.toString()} item={listItems} />;
});
return <ul>{updatedList}</ul>;
}
const menuItems1 = [1, 2, 3, 4, 5];
const menuItems2 = [1, 2, 3, 4, 5, 6];
ReactDOM.render(
<div>
<Navmenu menuitems={menuItems1} />
<Navmenu menuitems={menuItems2} />
</div>,
document.getElementById( "root" )
);
|
Output: This will be the output of the above code
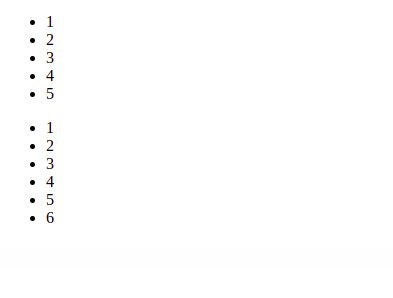
Share your thoughts in the comments
Please Login to comment...