React onClick Event
Last Updated :
28 Feb, 2024
React onClick is an event handler utilized to capture and respond to user clicks on specific elements, typically interactive ones like buttons or links.
It is similar to the HTML DOM onclick event but uses the camelCase convention in React. React onClick captures the user’s click responses and executes the handler functions.
React onClick Syntax:
onClick={handleClick}
React onClick Parameter:
- The callback function handleClick which is invoked when onClick event is triggered
React onClick Return Type:
- It is an event object containing information about the methods and is triggered when the mouse is clicked.
React onClick Event Examples:
Let’s see some examples of using the onClick event in react application.
Example 1: React onClick Event to change the Display Text
In this React functional component, two state variables (`num` and `btn`) are initialized using the `useState` hook, and the `handleClick` function toggles their boolean values, updating the displayed text and button label based on the click event.
Javascript
import React, { useState } from 'react' ;
import './App.css'
const App = () => {
const [num, setNum] = useState( false );
const [btn, setBtn] = useState( false );
const handleClick = () => {
setNum(!num);
setBtn(!btn);
};
return (
<div className= "App" >
<h2> {
num ?
"onClick event performed" :
"onClick event not performed"
}
</h2>
<button onClick={handleClick}>
{btn ? "clicked" : "onClick" }
</button>
</div>
);
};
export default App;
|
CSS
.App {
display : flex;
flex- direction : column;
justify- content : center ;
align-items: center ;
}
body {
background-color : antiquewhite;
}
.App>h 2 {
text-align : center ;
}
.App>button {
width : 8 rem;
font-size : larger ;
padding : 2 vmax auto ;
height : 1.8 rem;
color : white ;
background-color : rgb ( 34 , 34 , 33 );
border-radius: 10px ;
}
button:hover {
background-color : rgb ( 80 , 80 , 78 );
}
|
Output:
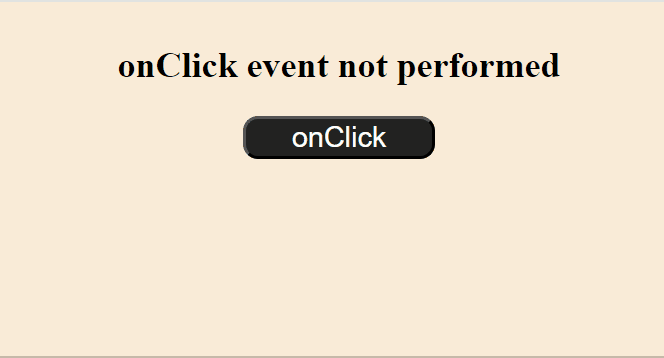
React onClick Event
Example 2: React onClick Event to Create Counter
In this React functional component, a state variable `num` is initialized using the `useState` hook, and the `handleClick` function updates the state by incrementing `num` by 1 when the button is clicked, displaying the updated value.
Javascript
import React, { useState } from 'react' ;
import './App.css'
const App = () => {
const [num, setNum] = useState(0);
const handleClick = () => {
setNum(num + 1);
};
return (
<div className= "App" >
<h2> {num}</h2>
<button onClick={handleClick}>
Add one
</button>
</div>
);
};
export default App;
|
CSS
.App {
display : flex;
flex- direction : column;
justify- content : center ;
align-items: center ;
}
body {
background-color : antiquewhite;
}
.App>h 2 {
text-align : center ;
}
.App>button {
width : 8 rem;
font-size : larger ;
padding : 2 vmax auto ;
height : 1.8 rem;
color : white ;
background-color : rgb ( 34 , 34 , 33 );
border-radius: 10px ;
}
button:hover {
background-color : rgb ( 80 , 80 , 78 );
}
|
Output:
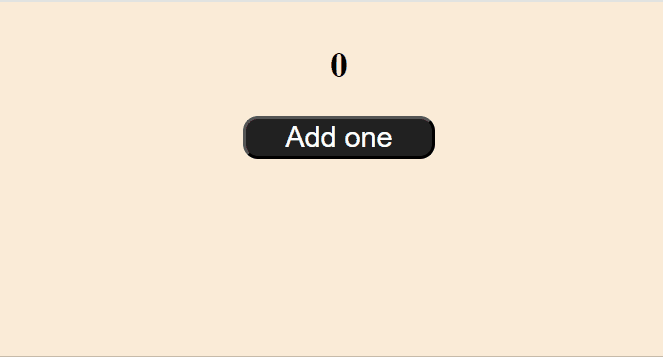
React onClick Event to Create Counter
Share your thoughts in the comments
Please Login to comment...