JavaScript onclick Event
Last Updated :
02 Feb, 2024
The onclick function in JavaScript is an event handler that is used to respond to or handle the mouse click on the web page. It takes a callback function which will be invoked later at the time when the event is triggered.
In JavaScript, we can use the onclick function in two ways as listed below:
Applying onclick event as an attribute
The onclick event can be passed as an attribute to an HTML element which makes it trigger a specified functionality on the web page when the user clicks on it.
Syntax:
<HTMLElement onclick = "callbackFunction">
<!-- Content of HTML element -->
</HTMLElement>
Example: The below code example implements the onclick function when it is passed as an attribute to an element.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content=" width = device -width,
initial-scale = 1 .0">
< title >
JavaScript onclick Function
</ title >
< style >
#container{
text-align: center;
}
#toggler{
display: none;
}
</ style >
</ head >
< body >
< div id = "container" >
< h1 style = "color: green;" >
GeeksforGeeks
</ h1 >
< h2 >
The below button uses the onclick function
to perform some functionality on click to it.
</ h2 >
< button onclick = "clickHandler()" >
Click me to see changes
</ button >
< h3 id = "toggler" >
Hey Geek, < br />
Welcome to GeeksforGeeks
</ h3 >
</ div >
< script >
function clickHandler(){
const toggler = document.getElementById('toggler');
console.log(toggler.style.display)
if(toggler.style.display === "block"){
toggler.style.display = "none";
}
else{
toggler.style.display = "block";
}
}
</ script >
</ body >
</ html >
|
Output:
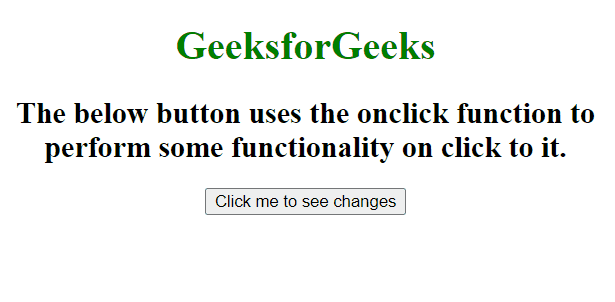
Applying onclick using addEventListener() method
There is a in-bulit method addEventListener() provided by JavaScript to attach events with the HTML elements. It takes the name of the event and the callback function as arguements to attach them.
Syntax:
selectedHTMLElement.addEvenetListener('eventName', callbackFunction);
Example: The below code implements the addEventListener() method to applying the onclick function.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content=" width = device -width,
initial-scale = 1 .0">
< title >
JavaScript onclick Function
</ title >
< style >
#container{
text-align: center;
}
#toggler{
display: none;
}
</ style >
</ head >
< body >
< div id = "container" >
< h1 style = "color: green;" >
GeeksforGeeks
</ h1 >
< h2 >
The below button uses the onclick function
to perform some functionality on click to it.
</ h2 >
< button id = "myBtn" >
Click me to see changes
</ button >
< h3 id = "toggler" >
Hey Geek, < br />
Welcome to GeeksforGeeks
</ h3 >
</ div >
< script >
const myBtn = document.getElementById('myBtn');
function clickHandler(){
const toggler = document.getElementById('toggler');
console.log(toggler.style.display)
if(toggler.style.display === "block"){
toggler.style.display = "none";
}
else{
toggler.style.display = "block";
}
}
myBtn.addEventListener('click', clickHandler);
</ script >
</ body >
</ html >
|
Output:
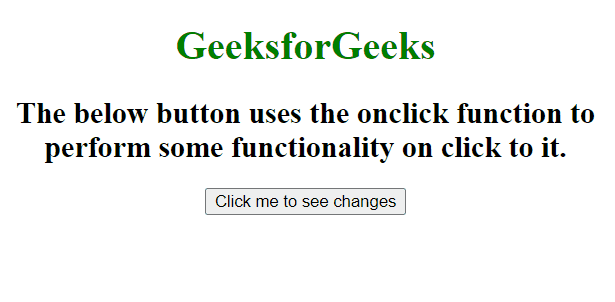
Share your thoughts in the comments
Please Login to comment...