React onFocus event
Last Updated :
04 Dec, 2023
The onFocus
event in React is triggered when an element receives focus, meaning it becomes the active element that can accept user input. This event is commonly used to execute functions or perform actions when an element gains focus.
It is similar to the HTML DOM onfocus event but uses the camelCase convention in React.
Syntax:
onFocus={handleOnFocus}
Parameter: The function handleOnFocus which is invoked when onFocus is triggered
Return Type:
It is an event object containing information about the methods and triggered on method calls.
Example 1:
The React component tracks the focus state of an input field, dynamically changing its background color and adding a border radius when focused, and resets styles when blurred.
Javascript
import React, { useState } from 'react' ;
function FocusExample() {
const [isFocused, setIsFocused] = useState( false );
const handleOnFocus = () => {
setIsFocused( true );
};
const handleBlur = () => {
setIsFocused( false );
};
return (
<div>
<label htmlFor= "myInput" >Write something: </label>
<input
type= "text"
id= "myInput"
onFocus={handleOnFocus}
onBlur={handleBlur}
style=
{
{
backgroundColor: isFocused ?
'antiquewhite' : 'white' ,
borderRadius: "5px"
}
}
/>
</div>
);
}
export default FocusExample;
|
Output:
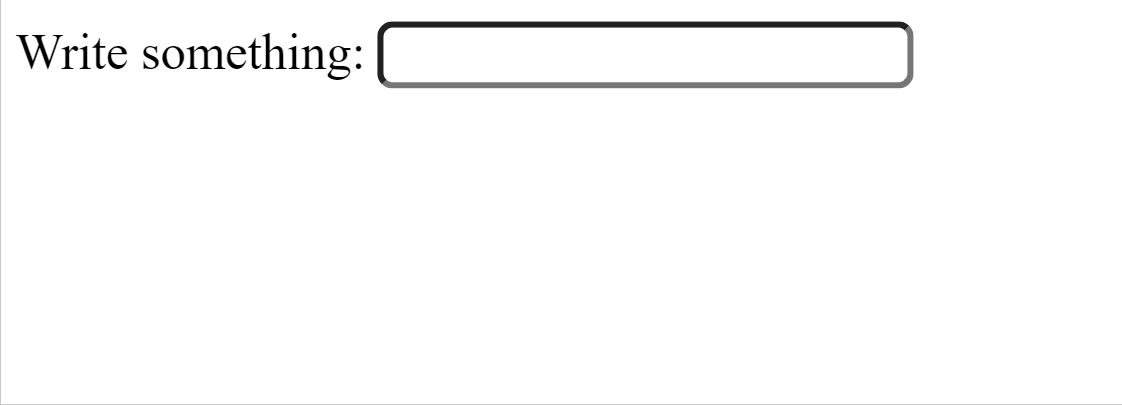
Output
Example 2: The React component tracks the focus state of an input field, displaying “onFocus Active” in red below the input when it gains focus and hiding the message when out of focus.
Javascript
import React, { useState } from 'react' ;
function App() {
const [isFocused, setIsFocused] = useState( false );
const handleOnFocus = () => {
setIsFocused( true );
};
const handleBlur = () => {
setIsFocused( false );
};
return (
<div>
<label htmlFor= "myInput" >
Enter your details:
</label>
<input
type= "text"
id= "myInput"
onFocus={handleOnFocus}
onBlur={handleBlur}
/>
{isFocused ? (
<div style=
{
{
marginTop: '7px' ,
color: 'red'
}
}>
onFocus Active
</div>
) : "" }
</div>
);
}
export default App;
|
Output:
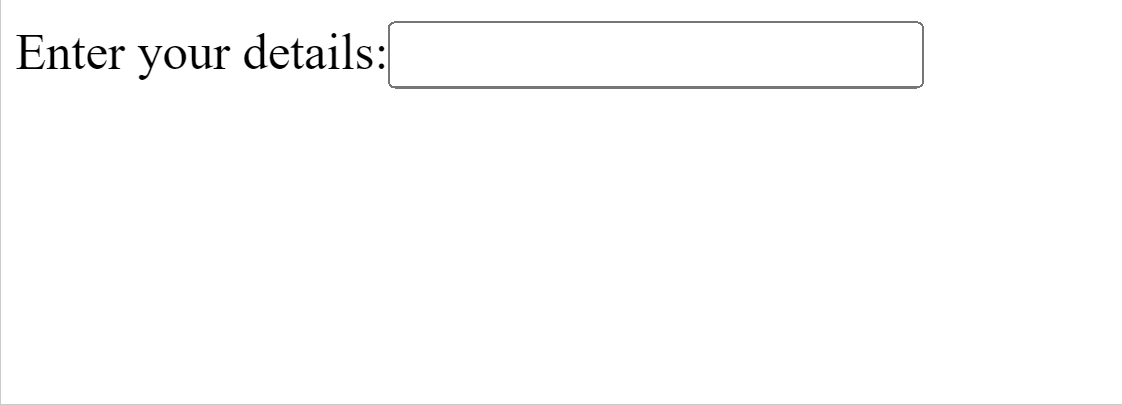
Output
Share your thoughts in the comments
Please Login to comment...