How to Add & Remove Value Onclick Event in HTML ?
Last Updated :
18 Apr, 2024
To add a value to an element’s onclick event in HTML, you can set the event attribute to a JavaScript function or expression, like onclick=”myFunction()”. To remove it, you can set the attribute to an empty string, like onclick=’ ‘. This allows for dynamic manipulation of event behavior based on user interactions.
Using addEventListener method
This approach uses the addEventListener method to efficiently manage value addition and removal, enhancing user interaction.
- Here we utilize Flexbox for a centered layout, enhancing visual organization.
- On button click, JavaScript dynamically creates list items labeled as “Item 1”, “Item 2”, etc.
- List items exhibit hover effects for improved user interaction, enhancing visual feedback.
- Utilizes event delegation for removing list items, optimizing performance, and code simplicity.
Example: Implementation to Add and Remove Value Onclick Event in HTML.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>
Add and Remove
Value Onclick
</title>
<style>
body {
display: flex;
height: 100vh;
justify-content: center;
align-items: center;
}
#valueContainer {
list-style-type: none;
padding: 0;
}
li {
margin-bottom: 5px;
padding: 5px 10px;
background-color: #f0f0f0;
border-radius: 5px;
cursor: pointer;
}
li:hover {
background-color: #e0e0e0;
}
</style>
</head>
<body>
<button id="addButton">
Add Value
</button>
<ul id="valueContainer"></ul>
<script>
let itemCount = 1;
// Function to add a new value
function addValue() {
const container =
document.getElementById('valueContainer');
const newValueElement =
document.createElement('li');
newValueElement.textContent = 'Item ' + itemCount++;
container.appendChild(newValueElement);
}
// Function to remove a value
function removeValue(target) {
if (target.tagName === 'LI') {
target.remove();
}
}
// Event listener for adding values
document.getElementById(
'addButton').addEventListener(
'click', addValue);
// Event delegation for removing values
document.getElementById(
'valueContainer').addEventListener(
'click', function (event) {
removeValue(event.target);
});
</script>
</body>
</html>
Output:
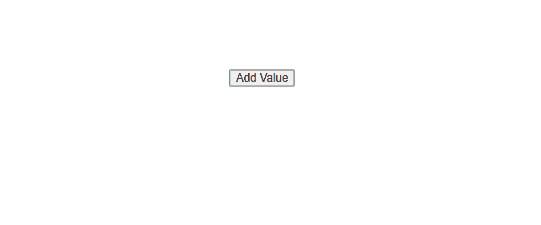
Example 2: Implementation to Add and Remove Value Onclick Event in HTML with another example.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>
Add and Remove Onclick Event
</title>
</head>
<body>
<button id="myButton">Click me!</button>
<script>
function handleClick() {
alert("Button clicked!");
}
document.getElementById(
"myButton").addEventListener(
"click", handleClick);
// Function to remove event
// listener from the button
function removeClickHandler() {
document.getElementById(
"myButton").removeEventListener(
"click", handleClick);
alert("Onclick event removed!");
}
// Adding a new button to
// remove the event handler
let removeButton = document.createElement("button");
removeButton.textContent = "Remove Onclick Event";
removeButton.addEventListener("click", removeClickHandler);
document.body.appendChild(removeButton);
</script>
</body>
</html>
Output:
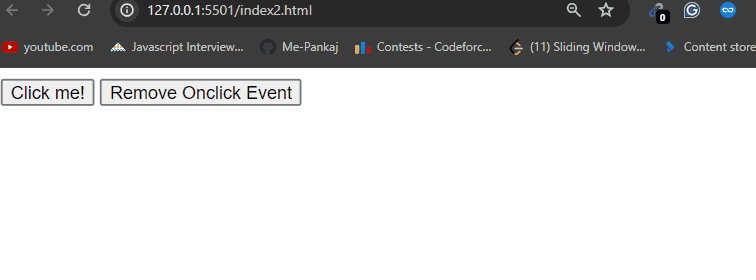
Share your thoughts in the comments
Please Login to comment...