For Loops in Python
Last Updated :
10 Apr, 2024
For Loops in Python are a special type of loop statement that is used for sequential traversal. Python For loop is used for iterating over an iterable like a String, Tuple, List, Set, or Dictionary.Â
In Python, there is no C style for loop, i.e., for (i=0; I <n; i++). The For Loops in Python is similar to each loop in other languages, used for sequential traversals.
Note: In Python, for loops only implement the collection-based iteration.
Python For Loop Syntax
for var in iterable:
# statements
Flowchart of Python For Loop
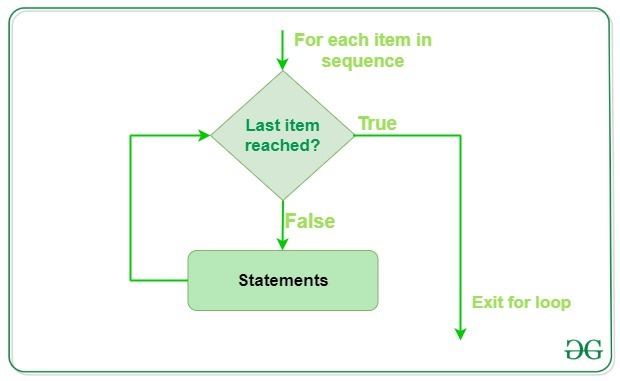
For Loop flowchart
Here the iterable is a collection of objects like lists, and tuples. The indented statements inside the for loops are executed once for each item in an iterable. The variable var takes the value of the next item of the iterable each time through the loop.
Examples of Python For Loop
Python For Loop with List
This code uses a for loop to iterate over a list of strings, printing each item in the list on a new line. The loop assigns each item to the variable I and continues until all items in the list have been processed.
Python
# Python program to illustrate
# Iterating over a list
l = ["geeks", "for", "geeks"]
for i in l:
print(i)
Output :
geeks
for
geeks
Python For Loop with Dictionary
This code uses a for loop to iterate over a dictionary and print each key-value pair on a new line. The loop assigns each key to the variable i and uses string formatting to print the key and its corresponding value.
Python
# Iterating over dictionary
print("Dictionary Iteration")
d = dict()
d['xyz'] = 123
d['abc'] = 345
for i in d:
print("% s % d" % (i, d[i]))
Output:
Dictionary Iteration
xyz 123
abc 345
Python For Loop with String
This code uses a for loop to iterate over a string and print each character on a new line. The loop assigns each character to the variable i and continues until all characters in the string have been processed.
Python
# Iterating over a String
print("String Iteration")
s = "Geeks"
for i in s:
print(i)
Output:
String Iteration
G
e
e
k
s
Python For Loop with a step size
This code uses a for loop in conjunction with the range() function to generate a sequence of numbers starting from 0, up to (but not including) 10, and with a step size of 2. For each number in the sequence, the loop prints its value using the print() function. The output will show the numbers 0, 2, 4, 6, and 8.
Python
for i in range(0, 10, 2):
print(i)
Output :
0
2
4
6
8
For Loops in Python inside a For Loop
This code uses nested for loops to iterate over two ranges of numbers (1 to 3 inclusive) and prints the value of i and j for each combination of the two loops. The inner loop is executed for each value of i in the outer loop. The output of this code will print the numbers from 1 to 3 three times, as each value of i is combined with each value of j.
Python
for i in range(1, 4):
for j in range(1, 4):
print(i, j)
Output :
1 1
1 2
1 3
2 1
2 2
2 3
3 1
3 2
3 3
Python For Loop with Zip()
This code uses the zip() function to iterate over two lists (fruits and colors) in parallel. The for loop assigns the corresponding elements of both lists to the variables fruit and color in each iteration. Inside the loop, the print() function is used to display the message “is” between the fruit and color values. The output will display each fruit from the list of fruits along with its corresponding color from the colours list.
Python
fruits = ["apple", "banana", "cherry"]
colors = ["red", "yellow", "green"]
for fruit, color in zip(fruits, colors):
print(fruit, "is", color)
Output :
apple is red
banana is yellow
cherry is green
Python For Loop with Tuple
This code iterates over a tuple of tuples using a for loop with tuple unpacking. In each iteration, the values from the inner tuple are assigned to variables a and b, respectively, and then printed to the console using the print() function. The output will show each pair of values from the inner tuples.
Python
t = ((1, 2), (3, 4), (5, 6))
for a, b in t:
print(a, b)
Output :
1 2
3 4
5 6
Control Statements that can be used with For Loop in Python
Loop control statements change execution from their normal sequence. When execution leaves a scope, all automatic objects that were created in that scope are destroyed. Python supports the following control statements.
For Loop in Python with Continue Statement
Python continue Statement returns the control to the beginning of the loop.
Python
# Prints all letters except 'e' and 's'
for letter in 'geeksforgeeks':
if letter == 'e' or letter == 's':
continue
print('Current Letter :', letter)
Output:Â
Current Letter : g
Current Letter : k
Current Letter : f
Current Letter : o
Current Letter : r
Current Letter : g
Current Letter : k
For Loop in Python with Break Statement
Python break statement brings control out of the loop.
Python
for letter in 'geeksforgeeks':
# break the loop as soon it sees 'e'
# or 's'
if letter == 'e' or letter == 's':
break
print('Current Letter :', letter)
Output:Â
Current Letter : e
For Loop in Python with Pass Statement
The pass statement to write empty loops. Pass is also used for empty control statements, functions, and classes.
Python
# An empty loop
for letter in 'geeksforgeeks':
pass
print('Last Letter :', letter)
Output:Â
Last Letter : s
For Loops in Python with Range Statement
The Python range() function is used to generate a sequence of numbers. Depending on how many arguments the user is passing to the function, the user can decide where that series of numbers will begin and end as well as how big the difference will be between one number and the next.range() takes mainly three arguments.Â
- start: integer starting from which the sequence of integers is to be returned
- stop: integer before which the sequence of integers is to be returned.Â
The range of integers ends at a stop – 1. - step: integer value which determines the increment between each integer in the sequence.
Python
# Python Program to
# show range() basics
# printing a number
for i in range(10):
print(i, end=" ")
# performing sum of first 10 numbers
sum = 0
for i in range(1, 10):
sum = sum + i
print("\nSum of first 10 numbers :", sum)
Output:
0 1 2 3 4 5 6 7 8 9
Sum of first 10 numbers : 45
For Loops in Python with Else Statement
Python also allows us to use the else condition for loops. The else block just after for/while is executed only when the loop is NOT terminated by a break statement.
Python
# Python program to demonstrate
# for-else loop
for i in range(1, 4):
print(i)
else: # Executed because no break in for
print("No Break\n")
Output:Â
1
2
3
No Break
Python For Loop Exercise Questions
Below are two Exercise Questions on Python for-loops. We have covered continue statement and range() function in these exercise questions.
Q1. Code to implement Continue statement in for-loop
Python
clothes = ["shirt", "sock", "pants", "sock", "towel"]
paired_socks = []
for item in clothes:
if item == "sock":
continue
else:
print(f"Washing {item}")
paired_socks.append("socks")
print(f"Washing {paired_socks}")
Output
Washing shirt
Washing pants
Washing towel
Washing ['socks']
Q2. Code to implement range function in for-loop
Python
for day in range(1, 8):
distance = 3 + (day - 1) * 0.5
print(f"Day {day}: Run {distance:.1f} miles")
Output
Day 1: Run 3.0 miles
Day 2: Run 3.5 miles
Day 3: Run 4.0 miles
Day 4: Run 4.5 miles
Day 5: Run 5.0 miles
Day 6: Run 5.5 miles
Day 7: Run 6.0 miles
Share your thoughts in the comments
Please Login to comment...