Python 3 basics
Last Updated :
31 Jul, 2023
Python 3 is a popular high-level programming language used for a wide variety of applications. Here are some basics of Python 3 that you should know:
- Variables: In Python 3, variables are created by assigning a value to a name. For example, x = 5 creates a variable called x and assigns the value 5 to it.
- Data types: Python 3 supports several built-in data types, including integers, floats, strings, booleans, lists, tuples, and dictionaries.
- Operators: Python 3 supports a variety of operators, including arithmetic operators (+, -, *, /), comparison operators (>, <, ==, !=), and logical operators (and, or, not).
- Control flow statements: Python 3 supports several control flow statements, including if-else statements, for loops, and while loops. These statements allow you to control the flow of execution in your code.
- Functions: In Python 3, functions are created using the def keyword. For example, def my_function(x): creates a function called my_function that takes one argument called x.
Input and output: In Python 3, you can use the input() function to get user input, and the print() function to output text to the console.
Modules: Python 3 supports modules, which are collections of functions and variables that can be imported and used in other Python code. You can import modules using the import keyword.
We also have a complete Python 3 Tutorial designed to learn Python 3 at all levels, from beginners to advanced. This comprehensive tutorial takes you through the fundamental concepts of Python and gradually progresses to more advanced topics.
Advantages of Python 3:
- Python 3 has a simple syntax that is easy to learn and read, making it a good choice for beginners.
- Python 3 is a high-level language that has a large standard library and many third-party libraries available, making it a versatile language that can be used for a wide variety of applications.
- Python 3 supports multiple programming paradigms, including object-oriented, functional, and procedural programming.
- Python 3 is an interpreted language, meaning that it does not need to be compiled before running, making it easy to write and test code quickly.
- Python 3 has good support for data analysis and scientific computing, with libraries such as NumPy and Pandas.
Disadvantages of Python 3:
- Python 3 can be slower than compiled languages such as C++ or Java, which may be a concern for applications that require high performance.
- Python 3 has a global interpreter lock (GIL), which can limit its ability to take advantage of multiple CPU cores.
- Python 3 may not be the best choice for low-level systems programming, as it does not offer the same level of control over hardware as other languages.
- Python 3 is not as popular in some fields as other languages, such as R for data analysis or C++ for game development, so it may not always be the best choice for specific applications.
Python was developed by Guido van Rossum in the early 1990s and its latest version is 3.11.0, we can simply call it Python3. Python 3.0 was released in 2008. and is interpreted language i.e it’s not compiled and the interpreter will check the code line by line. This article can be used to learn the very basics of Python programming language. So before moving on further.. let’s do the most popular ‘HelloWorld’ tradition 😛 and hence compare Python’s Syntax with C, C++, and Java ( I have taken these 3 because they are the most famous and mostly used languages).
Note: Please note that Python for its scope doesn’t depend on the braces ( { } ), instead it uses indentation for its scope. Let us start with our basics of Python where we will be covering the basics in some small sections. Just go through them and trust me you’ll learn the basics of Python very easily.
- If you are on Windows OS download Python by Clicking here and now install from the setup and in the start menu type IDLE.IDLE, you can think it as a Python’s IDE to run the Python Scripts. It will look somehow this :
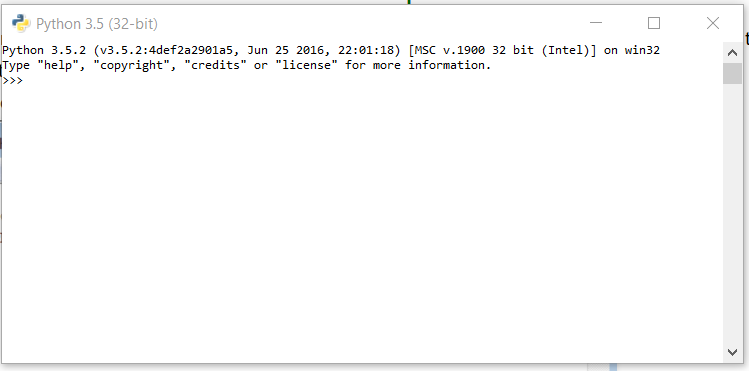
- If you are on Linux/Unix-like just open the terminal and on 99% linux OS Python comes preinstalled with the OS.Just type ‘python3’ in terminal and you are ready to go. It will look like this :
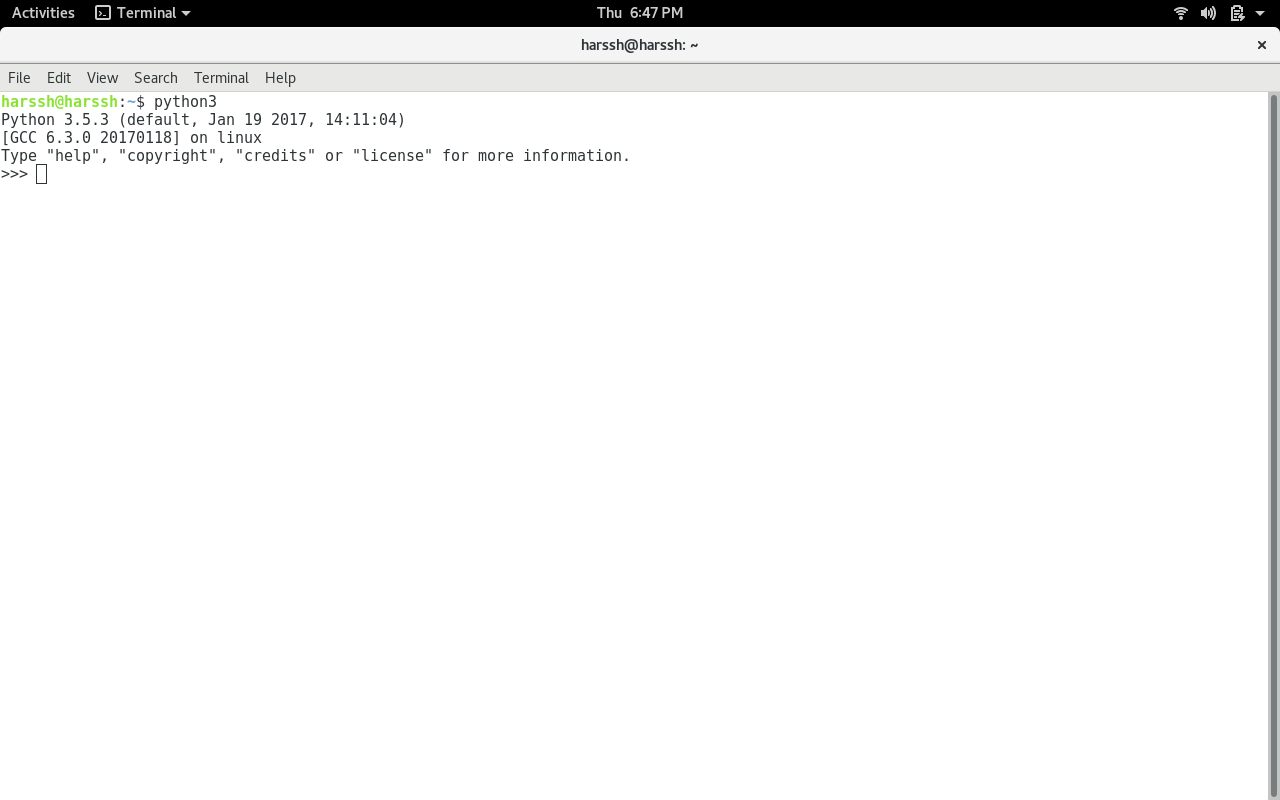
The ” >>> ” represents the python shell and its ready to take python commands and code.
In other programming languages like C, C++, and Java, you will need to declare the type of variables but in Python you don’t need to do that. Just type in the variable and when values will be given to it, then it will automatically know whether the value given would be an int, float, or char or even a String.
Python3
for step in range ( 5 ):
print (step)
|
See, how simple is it, just create a variable and assign it any value you want and then use the print function to print it. Python have 4 types of built-in Data Structures namely List, Dictionary, Tuple, and Set.
List is the most basic Data Structure in python. List is a mutable data structure i.e items can be added to list later after the list creation. It’s like you are going to shop at the local market and made a list of some items and later on you can add more and more items to the list.
append() function is used to add data to the list.
Python3
step = 0
while (step < 5 ):
print (step)
step = step + 1
|
Output
[21, 40.5, 'String']
Python3
import math
def Main():
num = - 85
num = math.fabs(num)
print (num)
if __name__ = = "__main__" :
Main()
|
Output
{1: 'Geeks', 2: 'For', 3: 'Geeks'}
Output
('Geeks', 'For', 'Geeks')
Python3
myset = set ([ "Geeks" , "For" , "Geeks" ])
print (myset)
|
Comments:
# is used for single line comment in Python
""" this is a comment """ is used for multi line comments
In this section, we will learn how to take input from the user and hence manipulate it or simply display it. input() function is used to take input from the user.
Python3
name = input ( "Enter your name: " )
print ( "hello" , name)
|
Output:
hello harssh
Python3
num1 = int ( input ( "Enter num1: " ))
num2 = int ( input ( "Enter num2: " ))
num3 = num1 * num2
print ( "Product is: " , num3)
|
Output:
Enter num1: 8 Enter num2: 6 ('Product is: ', 48)
Selection
Selection in Python is made using the two keywords ‘if’ and ‘elif’(elseif) and else
Python3
num1 = 34
if (num1> 12 ):
print ( "Num1 is good" )
elif (num1> 35 ):
print ( "Num2 is not gooooo...." )
else :
print ( "Num2 is great" )
|
Functions
You can think of functions like a bunch of code that is intended to do a particular task in the whole Python script. Python used the keyword ‘def’ to define a function.
Syntax:
def function-name(arguments):
#function body
Python3
def hello():
print ( "hello" )
print ( "hello again" )
hello()
hello()
|
Output
hello
hello again
hello
hello again
Now as we know any program starts from a ‘main’ function…lets create a main function like in many other programming languages.
Python3
def getInteger():
result = int ( input ( "Enter integer: " ))
return result
def Main():
print ( "Started" )
output = getInteger()
print (output)
if __name__ = = "__main__" :
Main()
|
As the name suggests it calls repeating things again and again. We will use the most popular ‘for and while’ loop here.
Python3
for step in range ( 5 ):
print (step)
|
Python3
step = 0
while (step < 5 ):
print (step)
step = step + 1
|
Python has a very rich module library that has several functions to do many tasks. You can read more about Python’s standard library by Clicking here
‘import’ keyword is used to import a particular module into your python code. For instance consider the following program.
Python3
import math
def Main():
num = - 85
num = math.fabs(num)
print (num)
if __name__ = = "__main__" :
Main()
|
These are some of the basics of the Python programming language and I will be covering both the intermediate and advanced level Python topics in my upcoming articles.
Your article will be reviewed first by the Geeks for Geeks team before publishing.
Share your thoughts in the comments
Please Login to comment...