Purpose of NgModule Decorator in Angular
Last Updated :
28 Mar, 2024
The NgModule decorator in Angular is like a blueprint for organizing and configuring different parts of your application. It’s like a set of instructions that tells Angular how to assemble the various components, directives, pipes, and services into cohesive units called modules. These modules help in managing dependencies, defining routing rules, and configuring providers.
Essentially, the NgModule decorator serves as a central hub for defining and organizing the building blocks of an Angular application, making it easier to develop, maintain, and scale complex web applications.
Angular NgModules are also classes with ‘@NgModule’ and it has metadata that describes how the different parts of the application will work together. Like javascript modules have their own files but NgModule does not have their own file, they only include metadata. it also helps to organize the components and services of the angular application.
Syntax:
import { NgModule } from '@angular/core';
@NgModule({
declarations: [ /* Components, directives, and pipes */],
imports: [ /* Other modules */],
exports: [ /* Exports of this module */],
providers: [ /* Services */],
bootstrap: [ /* Root component */]
})
export class MyNgModule { }
Here, declarations is the array of components, directives, and pipes that belong to this module, imports is the array of other modules that this module depends on, exports is the array of declarations that should be visible and accessible to components in other modules, providers are the array of services available for injection within this module and bootstrap is the array containing the root component(s) to bootstrap when this module is loaded.
Features of NgModules:
- Encapsulation:Â NgModule provides encapsulation by encapsulating components, directives, and pipes within a module, preventing naming conflicts and promoting modularity.
- Dependency Injection:Â NgModule facilitates dependency injection by allowing the registration of providers at the module level, making services available throughout the module and its components.
- Lazy Loading:Â NgModule supports lazy loading, enabling the loading of modules on-demand, thus improving application performance and reducing initial load times.
- Reusability:Â NgModule promotes code reusability by allowing modules to be imported into multiple feature modules, facilitating code organization and sharing across the application.
Purpose of NgModule Decorator
- Modularity: One of the primary purposes of the
NgModule
decorator is to provide modularity within Angular applications. Modules allows to group related components, directives, pipes, and services together, making it easier to manage and maintain code. - Encapsulation:
NgModule
enables encapsulation by defining boundaries between different parts of the application. Each module has its own scope, and components, services, and other elements defined within a module are encapsulated and not visible to other modules by default that prevents naming conflicts and promoting code isolation. - Dependency Injection Configuration:
NgModule
provides a mechanism for configuring dependency injection within Angular applications. By specifying providers at the module level, You can ensure that services are available for injection throughout the module and its components. - Bootstrapping: The
bootstrap
property in NgModule
is used to specify the root component of the application. This tells Angular which component to bootstrap when the application starts, serving as the entry point of the application’s component tree. - Compilation and Optimization: Angular’s compiler uses information provided by
NgModule
decorators to compile templates, optimize performance, and generate efficient code bundles for deployment. NgModule
helps Angular optimize the application’s loading time and runtime performance.
Steps to create app:
Step 1: Create a new Angular project
ng new my-angular-app
Step 2: Navigate to the project directory
cd my-angular-app
Step 3: Serve the application
ng serve
Project Structure:
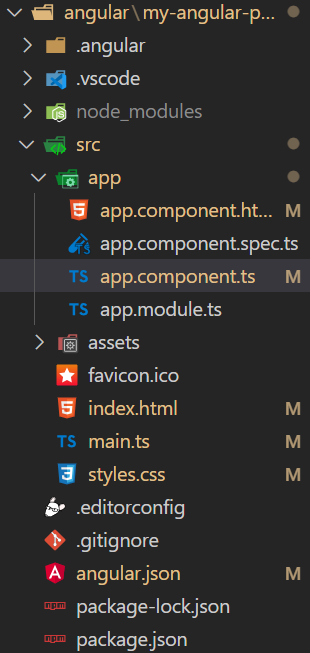
The updated dependencies in package.json file will look like.
"dependencies": {
"@angular/animations": "^16.0.0",
"@angular/common": "^16.0.0",
"@angular/compiler": "^16.0.0",
"@angular/core": "^16.0.0",
"@angular/forms": "^16.0.0",
"@angular/platform-browser": "^16.0.0",
"@angular/platform-browser-dynamic": "^16.0.0",
"@angular/router": "^16.0.0",
"rxjs": "~7.8.0",
"tslib": "^2.3.0",
"zone.js": "~0.13.0"
}
Example:
HTML
<!-- app.component.html -->
<div>
<h1 style="text-align: center; color: green">GeeksforGeeks</h1>
<div style="margin-left: 20px">
<h2>About Us</h2>
<p>
<strong>1. Company Profile and Brand:</strong><br />
GeeksforGeeks is a leading platform that provides
computer science resources and coding challenges for
programmers and technology enthusiasts, along with
interview and exam preparations for upcoming aspirants.
With a strong emphasis on enhancing coding skills and
knowledge, it has become a trusted destination for over
12 million plus registered users worldwide. The platform
offers a vast collection of tutorials, practice problems,
interview tutorials, articles, and courses,
covering various domains of computer science.
<br /><br />
Our exceptional mentors hailing from top colleges
& organizations have the ability to guide you on a
journey from the humble beginnings of coding to the
pinnacle of expertise. Under their guidance watch your
skills flourish as we lay the foundation and help
you conquer the world of coding.
</p>
<p>
<strong>2. Company Founders/Directors:</strong><br />
Our founder Sandeep Jain is a visionary entrepreneur and
esteemed computer science expert. Fueled by his
unwavering passion for coding and education,
laid the very bedrock upon which GeeksforGeeks stands
today, and his indomitable spirit has been instrumental
in its remarkable growth and resounding success. As
the steadfast driving force behind the company,
Sandeep remains a beacon of guidance and inspiration,
propelling the teamto constantly challenging
limits and craft transformative learning
experiences.
</p>
</div>
</div>
JavaScript
//app.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
})
export class AppComponent {
}
JavaScript
//app.module.ts
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppComponent } from './app.component';
@NgModule({
declarations: [AppComponent],
imports: [BrowserModule],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Output:
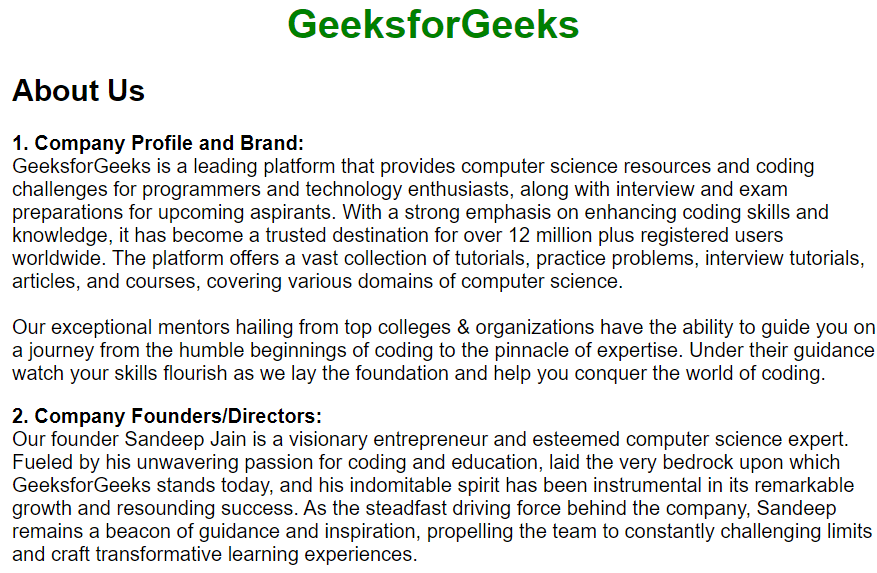
Share your thoughts in the comments
Please Login to comment...