Purpose of the FormsModule in Angular
Last Updated :
22 Mar, 2024
Forms are widely used in web applications that allow you to provide the data, submit the forms, and interact with the application. In Angular for handling the forms, we use Forms Module which imports several powerful tools for creating, managing, and validating forms. In this article, we’ll cover the purpose of the forms module, syntax, concepts, and examples related to Angular forms.
FormsModule
is a built-in Angular module provided by @angular/forms
package. It enables two-way data binding, form validation, and other form-related functionalities within Angular applications. By importing and including FormsModule
in your Angular application, you gain access to a range of directives, services, and utilities that streamline the process of working with forms.
Syntax: To use Forms module, we first have to import it into the app.module.ts from @angular/forms.
import { FormsModule } from '@angular/forms';
@NgModule({
// Other modules ...
imports: [BrowserModule, FormsModule],
})
export class AppModule {}
1. Template-Driven Forms
Template-driven forms mainly depends on angular directives. It relies on two-way data binding using “[(ngModel)]” directive allowing us to interact with the component directly. Here the form controls are automatically and state of the form is tracked by angular itself.
2. Reactive Forms
Reactive forms provide the direct access to the underlaying form’s object model. Here user have to create instances of FormControl, FormGroup and FormArray explicitly allowing more control and flexibility.
- Two-way Data Binding: One of the primary purposes of
FormsModule
is to provide two-way data binding between form controls and component properties. With two-way data binding, changes made in the template reflect in the component class and vice versa, enabling seamless synchronization of data between the view and the component. - Form Validation: Angular provides powerful form validation capabilities through
FormsModule
. It offers both template-driven and reactive form validation techniques. With FormsModule
, you can easily implement built-in validators such as required, min, max, pattern, etc., as well as create custom validators to enforce specific validation rules. - Form Controls and Directives:
FormsModule
provides a set of directives and form controls that simplify the creation and management of forms in Angular applications. Directives like ngModel
, ngForm
, and ngModelGroup
enable easy binding, grouping, and validation of form controls. Additionally, form controls like input
, select
, and textarea
are enhanced with additional features and functionality when used within FormsModule
. - Handling Form Submission: Another crucial aspect of
FormsModule
is handling form submission. It provides utilities to track the state of form controls, detect form submission events, and retrieve form data. This simplifies the process of submitting form data to backend services and handling responses effectively. - Error Handling and Feedback:
FormsModule
helps in displaying error messages and providing feedback to users during form interaction. It enables the dynamic display of validation errors, allowing users to identify and rectify input errors in real-time, thereby enhancing the overall user experience.
In this example, we’ll see form submission and handling of form data using Template Driven forms.
Step 1: Create a new Angular project by running the below command.
ng new <project_name>
Folder Structure:
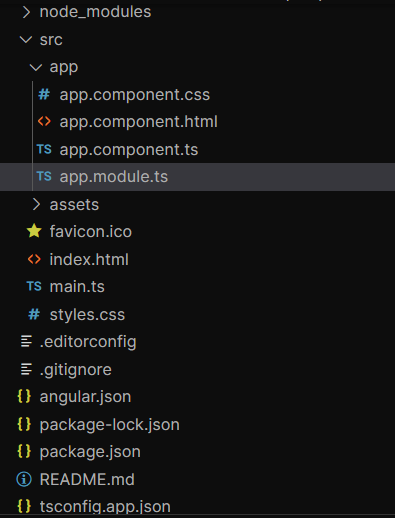
Project Structure
Dependencies:
"dependencies": {
"@angular/animations": "^17.3.0",
"@angular/common": "^17.3.0",
"@angular/compiler": "^17.3.0",
"@angular/core": "^17.3.0",
"@angular/forms": "^17.3.0",
"@angular/platform-browser": "^17.3.0",
"@angular/platform-browser-dynamic": "^17.3.0",
"@angular/platform-server": "^17.3.0",
"@angular/router": "^17.3.0",
"@angular/ssr": "^17.3.0",
"express": "^4.18.2",
"rxjs": "~7.8.0",
"tslib": "^2.3.0",
"zone.js": "~0.14.3"
},
"devDependencies": {
"@angular-devkit/build-angular": "^17.3.0",
"@angular/cli": "^17.3.0",
"@angular/compiler-cli": "^17.3.0",
"@types/express": "^4.17.17",
"@types/jasmine": "~5.1.0",
"@types/node": "^18.18.0",
"jasmine-core": "~5.1.0",
"karma": "~6.4.0",
"karma-chrome-launcher": "~3.2.0",
"karma-coverage": "~2.2.0",
"karma-jasmine": "~5.1.0",
"karma-jasmine-html-reporter": "~2.1.0",
"typescript": "~5.4.2"
}
Code Example: Add the following codes in the required files.
HTML
<!-- app.component.html -->
<form #userForm="ngForm" (ngSubmit)="onSubmit()">
<label for="name">Name:</label>
<input type="text" id="name" name="name" [(ngModel)]="user.name" required>
<label for="email">Email:</label>
<input type="email" id="email" name="email" [(ngModel)]="user.email" required>
<button type="submit">Submit</button>
</form>
<h3>User Details</h3>
<p>{{userDetails}}</p>
Javascript
// app.module.ts
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppComponent } from './app.component';
import { FormsModule } from '@angular/forms';
@NgModule({
declarations: [AppComponent],
imports: [BrowserModule, FormsModule],
providers: [],
bootstrap: [AppComponent],
})
export class AppModule { }
JavaScript
// app.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
})
export class AppComponent {
user = { name: '', email: '' };
userDetails = 'Loading...';
onSubmit() {
this.userDetails =
'User name is ' + this.user.name + ' and email is ' + this.user.email;
}
}
Output:
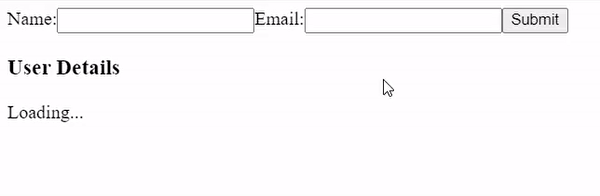
Output for example 1
Example 2: Form Handling using Reactive Forms.
In this example, we’ll see form submission and handling of form data using Reactive forms.
Code Example: Add the following codes in the respective files.
HTML
<!-- app.component.html -->
<form [formGroup]="myForm" (ngSubmit)="onSubmit()">
<label for="name">Name:</label>
<input type="text" id="name" formControlName="name">
<label for="email">Email:</label>
<input type="email" id="email" formControlName="email">
<button type="submit">Submit</button>
</form>
<h3>Form Details</h3>
<p>{{formDetails}}</p>
Javascript
// app.module.ts
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppComponent } from './app.component';
import { FormsModule, ReactiveFormsModule } from '@angular/forms';
@NgModule({
declarations: [AppComponent],
imports: [BrowserModule, FormsModule, ReactiveFormsModule],
providers: [],
bootstrap: [AppComponent],
})
export class AppModule { }
JavaScript
//app.component.ts
import { Component } from '@angular/core';
import { FormBuilder, FormGroup, Validators } from '@angular/forms';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
})
export class AppComponent {
myForm: FormGroup = {} as FormGroup;
formDetails: string = 'loading...';
constructor(private fb: FormBuilder) { }
ngOnInit() {
this.myForm = this.fb.group({
name: ['', [Validators.required]],
email: ['', [Validators.required, Validators.email]],
});
}
onSubmit() {
this.formDetails =
'Name: ' + this.myForm.value.name + ' Email:' + this.myForm.value.email;
}
}
Output:
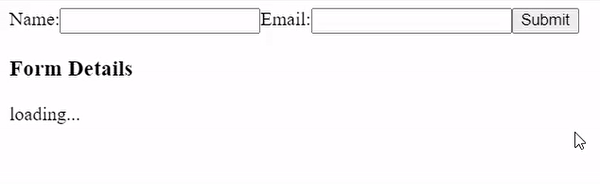
Output for example 2
Share your thoughts in the comments
Please Login to comment...