What is the HostListener Decorator?
Last Updated :
15 Apr, 2024
The HostListener decorator in Angular is a feature that allows us to listen for events on the host element of a component. In simpler terms, it’s like having a watch on the main element of our component, so that we can detect when certain events, such as mouse clicks or keyboard inputs, occur on that element. By using HostListener, we can then trigger specific functions or actions within our Angular component in response to these events.
Syntax:
@HostListener(eventName: string, args?: any[])
The @HostListener decorator in Angular is used to attach event listeners to the host element of a component. It takes two parameters: eventName, which specifies the name of the event to listen for (such as ‘click’ or ‘key down’), and optional args, which can be used to pass additional arguments to the event handler function. When the specified event occurs on the host element, the decorated method is invoked, allowing you to execute custom logic in response to that event.
Features of HostListener Decorator
- Event Binding: Allows binding methods to specific events on the host element.
- Access to Event Data: Provides access to event data such as event properties and event objects.
- Granular Control: Offers granular control over event triggering.
- Dynamic Behavior: Enables dynamic behavior based on component state or input properties.
- Declarative Approach: Promotes a declarative approach to event handling.
Benefits of HostListener Decorator
- Encapsulated Behavior: Encapsulates event-related logic within components or directives.
- Code Reusability: Promotes code reusability by encapsulating event handling logic.
- Improved Readability: Enhances code readability by declaring event handlers within the component class.
- Testing Facilitation: Facilitates unit testing of event-related behavior.
- Dynamic UI Interactions: Enables dynamic user interface interactions based on user actions.
Steps to Create Application:
Step 1: Create a new Angular project
ng new my-angular-app
Step 2: Navigate to the project directory
cd my-angular-app
Step 3: Serve the application
ng serve
Project Structure:
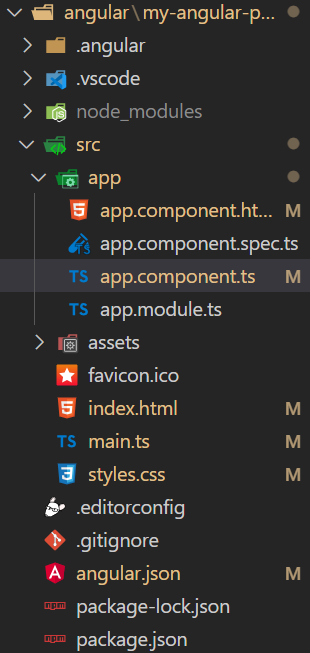
Dependencies:
"dependencies": {
"@angular/animations": "^16.0.0",
"@angular/common": "^16.0.0",
"@angular/compiler": "^16.0.0",
"@angular/core": "^16.0.0",
"@angular/forms": "^16.0.0",
"@angular/platform-browser": "^16.0.0",
"@angular/platform-browser-dynamic": "^16.0.0",
"@angular/router": "^16.0.0",
"rxjs": "~7.8.0",
"tslib": "^2.3.0",
"zone.js": "~0.13.0"
}
Example: In this example on clicking show button or pressing Enter using HostListner the text in input box will be displayed.
HTML
<!-- app.component.html -->
<div style="text-align: center;">
<h1 style="color: green;">GeeksforGeeks</h1>
<input type="text" [(ngModel)]=
"inputText" placeholder="Enter your text">
<button (click)="displayText()">Show</button>
<p *ngIf="displayHi">Hi {{ inputText }}</p>
</div>
JavaScript
//app.module.ts
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { FormsModule } from '@angular/forms';
import { AppComponent } from './app.component';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
FormsModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
JavaScript
//app.component.ts
import { Component, HostListener } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
})
export class AppComponent {
inputText: string = '';
displayHi: boolean = false;
@HostListener('document:keypress', ['$event'])
handleKeyboardEvent(event: KeyboardEvent) {
if (event.key === 'Enter') {
this.displayText();
}
}
displayText() {
this.displayHi = true;
}
}
Output:
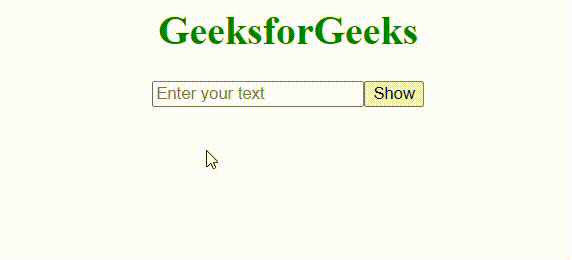
Share your thoughts in the comments
Please Login to comment...