Interpolation vs. Property Binding in Angular
Last Updated :
20 Mar, 2024
Angular, a powerful front-end framework, offers various techniques to dynamically update and display data in your applications. Two commonly used methods for this purpose are interpolation and property binding.
In this article, we will learn about interpolation and property binding and also see the key differences between them.
Interpolation
Interpolation is a one-way data binding technique in Angular that allows you to embed expressions within double curly braces {{ }} directly in the HTML template. The expression is evaluated and the result is converted to a string, which is then rendered in the view.
Syntax of interpolation:
<p>{{ expression }}</p>
Features of Interpolation:
- Simple syntax for embedding dynamic values.
- Automatic data conversion to string.
- Ideal for displaying data in templates.
Example of Interpolation:
Step 1: Create Angular Project using the following command.
ng new interpolation-example
cd interpolation-example
Step 2: Create a component ‘welcome’ using the following command.
ng generate component welcome
Folder Structure:

Dependencies:
"dependencies": {
"@angular/animations": "^17.3.0",
"@angular/common": "^17.3.0",
"@angular/compiler": "^17.3.0",
"@angular/core": "^17.3.0",
"@angular/forms": "^17.3.0",
"@angular/platform-browser": "^17.3.0",
"@angular/platform-browser-dynamic": "^17.3.0",
"@angular/platform-server": "^17.3.0",
"@angular/router": "^17.3.0",
"@angular/ssr": "^17.3.0",
"express": "^4.18.2",
"rxjs": "~7.8.0",
"tslib": "^2.3.0",
"zone.js": "~0.14.3"
},
"devDependencies": {
"@angular-devkit/build-angular": "^17.3.0",
"@angular/cli": "^17.3.0",
"@angular/compiler-cli": "^17.3.0",
"@types/express": "^4.17.17",
"@types/jasmine": "~5.1.0",
"@types/node": "^18.18.0",
"jasmine-core": "~5.1.0",
"karma": "~6.4.0",
"karma-chrome-launcher": "~3.2.0",
"karma-coverage": "~2.2.0",
"karma-jasmine": "~5.1.0",
"karma-jasmine-html-reporter": "~2.1.0",
"typescript": "~5.4.2"
}
Code Example:
HTML
<!-- welcome.component.html -->
<p>Welcome, {{ username }}!</p>
HTML
<!-- app.component.html -->
<app-welcome></app-welcome>
JavaScript
//welcome.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-welcome',
templateUrl: './welcome.component.html',
styleUrls: ['./welcome.component.css']
})
export class WelcomeComponent {
username: string = 'Sourav Sharma';
}
Run the Application using the following command.
ng serve --open
Output:
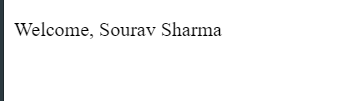
Property Binding
Property binding is another one-way data binding technique in Angular that allows you to bind a property of a DOM element to a component’s property. This binding is achieved using square brackets [] in the HTML template.
Syntax of Property Binding:
<element [property]="expression"></element>
Features of Property Binding:
- Binding component properties to HTML element properties.
- Dynamic updates based on component property changes.
- Ideal for handling properties like disabled, src, href, etc.
Example of Property Binding:
Step 1: Create Angular Project
ng new property-binding-example
cd property-binding-example
Step 2: Create Component
ng generate component button
Folder Structure:
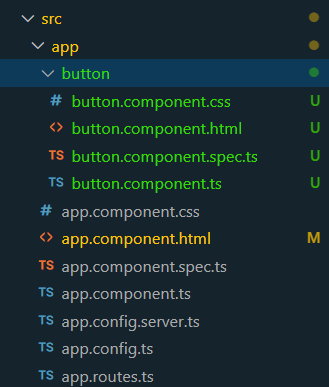
Dependencies:
"dependencies": {
"@angular/animations": "^17.3.0",
"@angular/common": "^17.3.0",
"@angular/compiler": "^17.3.0",
"@angular/core": "^17.3.0",
"@angular/forms": "^17.3.0",
"@angular/platform-browser": "^17.3.0",
"@angular/platform-browser-dynamic": "^17.3.0",
"@angular/platform-server": "^17.3.0",
"@angular/router": "^17.3.0",
"@angular/ssr": "^17.3.0",
"express": "^4.18.2",
"rxjs": "~7.8.0",
"tslib": "^2.3.0",
"zone.js": "~0.14.3"
},
"devDependencies": {
"@angular-devkit/build-angular": "^17.3.0",
"@angular/cli": "^17.3.0",
"@angular/compiler-cli": "^17.3.0",
"@types/express": "^4.17.17",
"@types/jasmine": "~5.1.0",
"@types/node": "^18.18.0",
"jasmine-core": "~5.1.0",
"karma": "~6.4.0",
"karma-chrome-launcher": "~3.2.0",
"karma-coverage": "~2.2.0",
"karma-jasmine": "~5.1.0",
"karma-jasmine-html-reporter": "~2.1.0",
"typescript": "~5.4.2"
}
Code Example:
HTML
<!-- app.component.html -->
<app-button></app-button>
HTML
<!-- button.component.html -->
<button [disabled]="isDisabled"
(click)="toggleDisabled()">Toggle</button>
JavaScript
// button.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-button',
templateUrl: './button.component.html',
styleUrls: ['./button.component.css']
})
export class ButtonComponent {
isDisabled: boolean = true;
toggleDisabled() {
this.isDisabled = !this.isDisabled;
}
}
Run the Application using the following command
ng serve --open
Output:
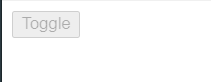
Difference between interpolation and property binding in Angular
In Angular, interpolation and property binding are two ways to bind data and display it in the view. Here’s a tabular comparison between interpolation and property binding:
Feature
| Interpolation
| Property Binding
|
---|
Syntax
| {{ expression }}
| [property]=”expression”
|
Use case
| One-way binding for displaying data
| One-way binding for setting an element’s property
|
Direction of data flow
| Component to view
| Component to view
|
Binding to DOM properties
| Works with element content
| Works with element properties
|
Example
| `<p>{{ message }}</p>`
| `<input [value]=”username”>`
|
Expressions
| Allows simple expressions
| Allows complex expressions and method calls
|
Data types
| Handles strings and simple variables
| Handles any valid JavaScript expressions
|
Dynamic updates
| Automatically updates when data changes
| Automatically updates when data changes
|
Angular Directive Support
| Limited to built-in directives like ngIf, ngFor, etc.
| Supports all directives including custom directives
|
Summary:
Interpolation and property binding are powerful techniques in Angular for achieving one-way data binding. Interpolation is ideal for displaying dynamic data directly in templates, using a simple {{ }} syntax. On the other hand, property binding is more suited for binding component properties to HTML element properties, allowing for dynamic updates based on changes in the component. Understanding the differences between these two techniques will empower you to choose the right approach for different scenarios in your Angular applications.
Share your thoughts in the comments
Please Login to comment...