Differece between JavaScript Modules & NgModule
Last Updated :
15 Mar, 2024
Modules play an important role in structuring and organizing the code of Javascript and Angular.js. They help us to use the code efficiently. Majorly NgModule in Angular.js and Modules in Javascript are widely used in different scenarios.
In this article, We will learn about Modules and their importance in Angular.js and Javascript.
Javascript Modules:
Javascript Modules are the files that contain the code of javascript that can be used multiple times by importing it inside the code anywhere. It prevents global clashes and helps us to write clear and clean code with encapsulation, abstraction, reuse, and efficiently manage the dependencies.
Syntax: A JavaScript module is defined using the export
keyword to expose functionality, and the import
keyword to import functionality from other modules.
// myModule.js
export function myFunction() {
// function implementation
}
// app.js
import { myFunction } from './myModule.js';
Features of JavaScript Modules:
- Encapsulation: JavaScript modules offer encapsulation by defining private and public interfaces, allowing developers to control the visibility and accessibility of module members.
- Code Organization: Modules enable developers to organize code into logical units, improving maintainability and readability by reducing complexity and separating concerns.
- Dependency Management: Modules facilitate dependency management by specifying explicit dependencies between modules, making it easier to understand and reason about code dependencies.
- Browser Compatibility: JavaScript modules are supported by modern browsers and are compatible with tools like Webpack, Rollup, and Babel, making them suitable for building modular web applications.
Example of JavaScript Modules:
Step 1: Initialize the application using the following command.
npm init -y
Step 2: In Package.json, Put a line ( “type”: “module” ).
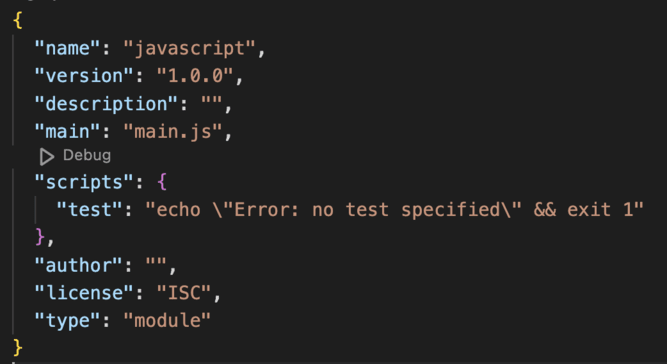
Folder Structure:
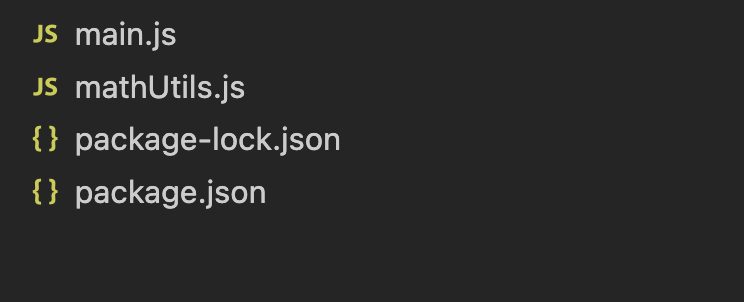
Code Example: Create the following files and add the following codes.
Javascript
// mathUtils.js
export function cube(x) {
return x * x * x;
}
JavaScript
// main.js
import { cube } from './mathUtils.js';
const result = cube(10);
console.log(`The square of 10 is: ${result}`);
Output:
NgModules
Angular NgModules are also classes with ‘@NgModule’ and it has metadata that describe how the different parts of the application will work together. Like javascript modules have their own files but NgModule does not have their own file, they only include metadata. it also helps to organise that component and services of the angular application.
Syntax: An NgModule is defined using the @NgModule decorator, which accepts a metadata object containing various configuration options such as declarations, imports, providers, and exports.
import { NgModule } from '@angular/core';
@NgModule({
declarations: [ /* components, directives, and pipes */ ],
imports: [ /* imported modules */ ],
providers: [ /* services */ ],
exports: [ /* exported components, directives, and pipes */ ]
})
export class MyModule { }
Features of NgModules:
- Encapsulation: NgModule provides encapsulation by encapsulating components, directives, and pipes within a module, preventing naming conflicts and promoting modularity.
- Dependency Injection: NgModule facilitates dependency injection by allowing the registration of providers at the module level, making services available throughout the module and its components.
- Lazy Loading: NgModule supports lazy loading, enabling the loading of modules on-demand, thus improving application performance and reducing initial load times.
- Reusability: NgModule promotes code reusability by allowing modules to be imported into multiple feature modules, facilitating code organization and sharing across the application.
Example of NgModule :
Step 1: Install the angular cli using the following command.
npm install -g @angular/cli
Step 2: Create a new angular App
ng new my-angular-project
Folder Structure:
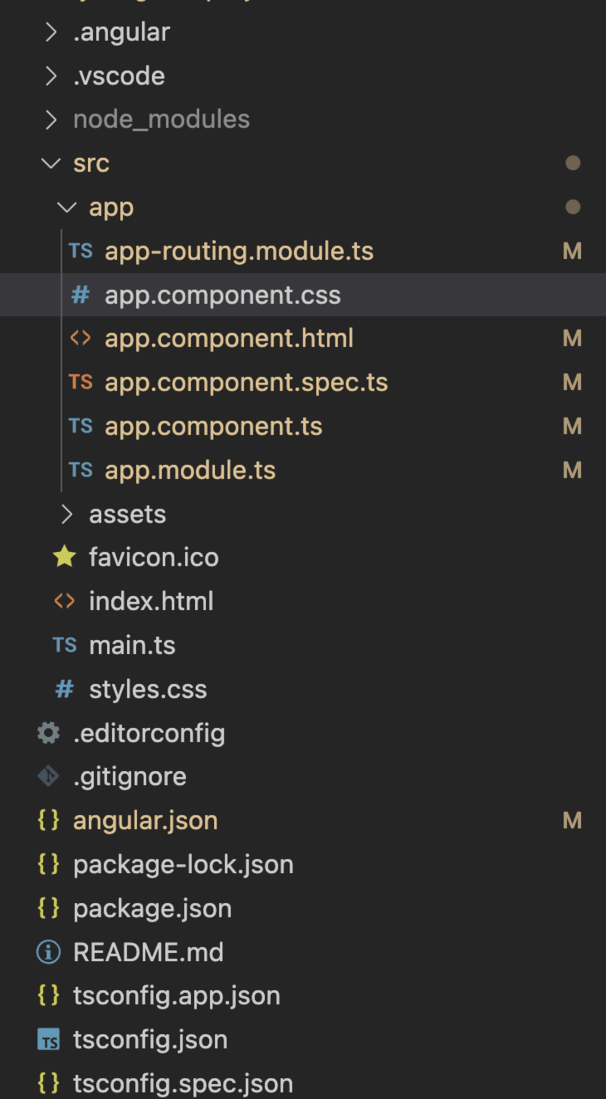
The updated Dependencies in package.json file will look like:
"dependencies": {
"@angular/animations": "^17.3.0",
"@angular/common": "^17.3.0",
"@angular/compiler": "^17.3.0",
"@angular/core": "^17.3.0",
"@angular/forms": "^17.3.0",
"@angular/platform-browser": "^17.3.0",
"@angular/platform-browser-dynamic": "^17.3.0",
"@angular/router": "^17.3.0",
"rxjs": "~7.8.0",
"tslib": "^2.3.0",
"zone.js": "~0.14.3"
}
Code Example: Ctreate the required files and add the following codes.
HTML
// src/app/app.component.html
<h1>Hello {{Name}}</h1>
Javascript
//src/app/app.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'my-angular-project';
}
JavaScript
// app.module.ts
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
@NgModule({
declarations: [
AppComponent,
],
imports: [
BrowserModule,
AppRoutingModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule {
}
To start the application run the following command.
ng serve
Output:
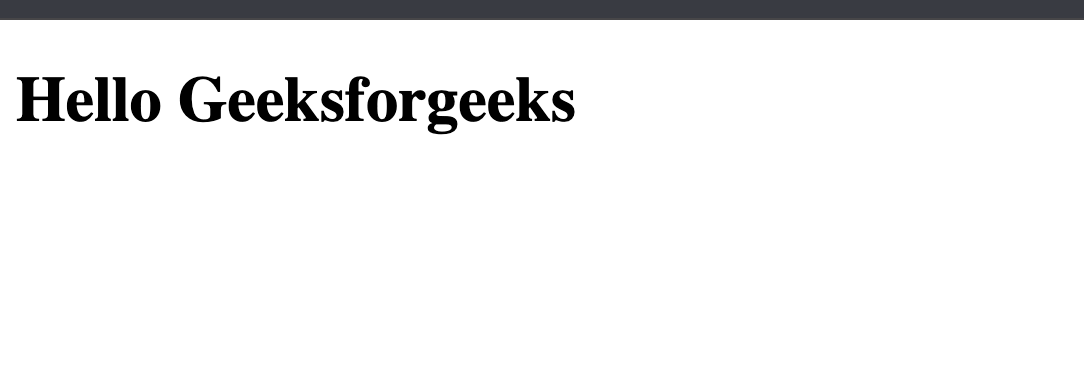
Difference between NgModule and JavaScript Modules
Javascript Modules
| NgModules
|
---|
Contains Individual File of javascript code.
| Contains classes with metadata marked by @Ngmodule decorator.
|
export statement is used to exporting the code.
| @Ngmodule is used with metadata.
|
Across files, it organise and manage the code.
| it structures and configure the angular components.
|
metadata is not present in it.
| Metadata is needed for angular compilation.
|
Organise file in separated files
| Kept the code in separate file but not necessity.
|
Can be used in Angular application but lacks of Angular-specific features.
| Essential for structuring angular application.
|
Conclusion:
Javascript modules and NgModules are used for a common purpose that is for organising the code but their implementation is different from each other. Javascript module focus on file-based code organisation but NgModule is specially for Angular Applications.
Share your thoughts in the comments
Please Login to comment...