What is the Purpose of Exports Array in NgModule?
Last Updated :
05 Apr, 2024
NgModule is a class marked by the @NgModule decorator. It identifies the module’s own components, directives, and pipes, making some of them public, through the exports property, so that external components can use them. The @NgModule decorator takes a metadata object as an argument. This object configures various aspects of the module, such as declarations, exports, imports, providers, and bootstrap.
Prerequisites
What is the Exports Array?
In Angular, a NgModule is a class with the @NgModule decorator. It acts as a container for organizing related components, directives, and pipes into functional units. The exports array within a NgModule is a key configuration property that defines which of its components, directives, or pipes can be accessed and used by other NgModules within the same application.
Purpose of the Exports Array:
- Sharing Components, Directives, and Pipes: Angular applications often have multiple NgModules, each containing a set of related functionalities. By specifying certain components, directives, or pipes in the exports array, you can make them available for use in other NgModules. This helps in code reusability and avoids duplication of code across the application.
- Creating Reusable Libraries: The exports array plays a crucial role in creating reusable libraries or modules in Angular. You can package a set of components, directives, or pipes into a separate NgModule and expose them via the exports array.
- Encapsulation and Abstraction: While Angular allows modularity and encapsulation, it also allows selective exposure of functionalities to other parts of the application.
- Promoting Consistency and Best Practices: By declaring the exported components, directives, or pipes in an NgModule, you can establish a clear contract for consuming these functionalities in other parts of the application.
Example
Step 1: Install the Angular CLI using the following command
npm install -g @angular/cli
Step 2: Create a new Angular Project.
ng new new-project
cd new-project
Step 3: To start the application run the following command.
ng serve
Step 4: Let us create a new module
cd src
ng g module sample
So here, we are creating a new module named sample .
Step 5 : Let us create a new component inside the module
ng g c loader
The above command creates a new component called loader inside the sample module.
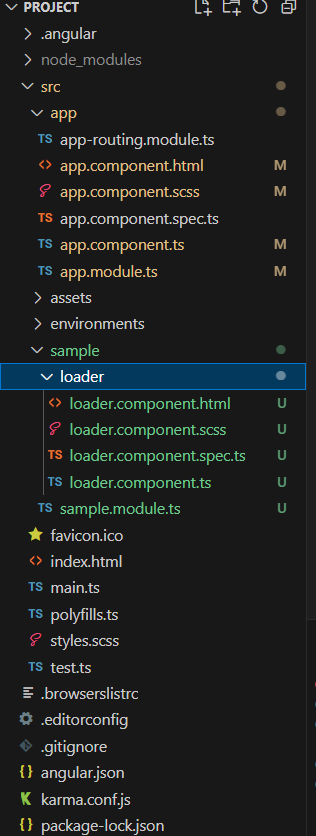
The updated Dependencies in package.json file will look like:
"dependencies": {
"@angular/animations": "^17.3.0",
"@angular/common": "^17.3.0",
"@angular/compiler": "^17.3.0",
"@angular/core": "^17.3.0",
"@angular/forms": "^17.3.0",
"@angular/platform-browser": "^17.3.0",
"@angular/platform-browser-dynamic": "^17.3.0",
"@angular/router": "^17.3.0",
"rxjs": "~7.8.0",
"tslib": "^2.3.0",
"zone.js": "~0.14.3"
}
Step 6: Usage of component
HTML
<!-- app.component.html -->
<div>
<h3>Usage of Component from other module:</h3>
<app-loader></app-loader>
</div>
HTML
<!-- loader.component.html -->
<p>loader component from other module works!</p>
JavaScript
//AppModule.ts
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
import { FormsModule } from '@angular/forms';
import { SampleModule } from 'src/sample/sample.module';
import { BrowserAnimationsModule } from '@angular/platform-browser/animations';
@NgModule({
declarations: [AppComponent],
imports: [
BrowserModule,
AppRoutingModule,
FormsModule,
SampleModule,
BrowserAnimationsModule,
],
providers: [],
bootstrap: [AppComponent],
})
export class AppModule { }
JavaScript
//sample.module.ts
import { NgModule } from '@angular/core';
import { CommonModule } from '@angular/common';
import { LoaderComponent } from './loader/loader.component';
@NgModule({
declarations: [LoaderComponent],
imports: [CommonModule],
exports: [LoaderComponent],
})
export class SampleModule { }
Output:
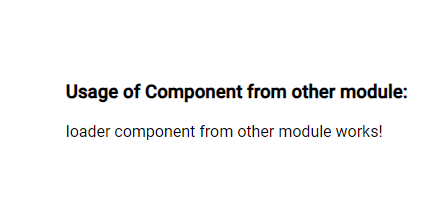
Share your thoughts in the comments
Please Login to comment...