Angular | keyup event
Last Updated :
11 Jun, 2020
Introduction
Here, we will be explaining the (keyup) event and options that can be used with (keyup) in Angular2. There are various options that can be used with (keyup) event but first let me explain you what is (keyup).
(keyup):
(keyup) is an Angular event binding to respond to any DOM event. It is a synchronous event that is triggered as the user is interacting with the text-based input controls.
When a user presses and releases a key, the (keyup) event occurs. For using in text-based input controls it is generally used to get values after every keystroke.
Basic Syntax of (keyup)
< input (keyup)="function(parameter)">
|
Angular provides a corresponding DOM event object in the $event variable which can also be used with (keyup) event to pass values or event.
For passing event syntax will be:
< input (keyup)="keyFunc($event)">
|
Approach for using (keyup):
Using (keyup) we can call user-defined function which contains the logic to display the text.
- create an input element which will take input.
- In that input element call the user-defined function on (keyup) event by passing $event.
- Take that $event to user-defined function in TypeScript file and take value from event variable by event.target.value and add that to local variable to see synchronous changes on keystroke.
Example:
Here in this implementation, $event is passed to user defined function onKeyUp() in typescript file. The function add every value in input after every keystroke to the text variable defined, the text variable is then displayed.
component.html file
< input (keyup)="onKeyUp($event)">
< p >{{text}}</ p >
|
component.ts file:
import { Component, OnInit } from '@angular/core' ;
@Component({
selector: 'app-txtchk' ,
templateUrl: './txtchk.component.html' ,
styleUrls: [ './txtchk.component.css' ]
})
export class TxtchkComponent implements OnInit {
text = '' ;
ngOnInit(): void {
}
onKeyUp(x) {
this .text += x.target.value + ' | ' ;
}
}
|
Output:
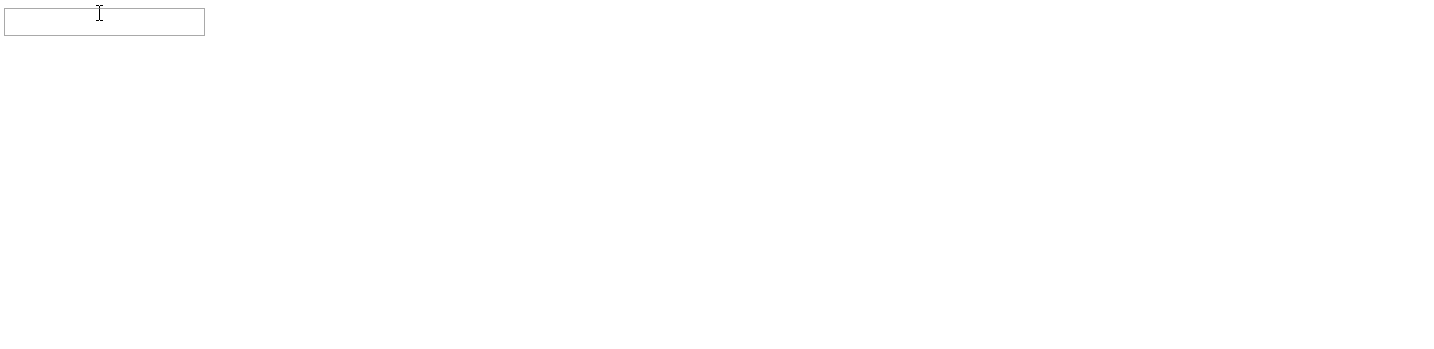
Options for (keyup):
There are many options that can be used with (keyup) event:
- (keyup.Space)
(keyup.Space) event is used to generate event when spacebar key is pressed.lets take above example just changing the (keyup) to (keyup.Space).
Example Implementation
component.html file
< input (keyup.Space)="onKeyUp($event)">
< p >{{text}}</ p >
|
component.ts file
import { Component, OnInit } from '@angular/core' ;
@Component({
selector: 'app-txtchk' ,
templateUrl: './txtchk.component.html' ,
styleUrls: [ './txtchk.component.css' ]
})
export class TxtchkComponent implements OnInit {
text = '' ;
ngOnInit(): void {
}
onKeyUp(x) {
this .text += x.target.value + ' | ' ;
}
}
|
Output
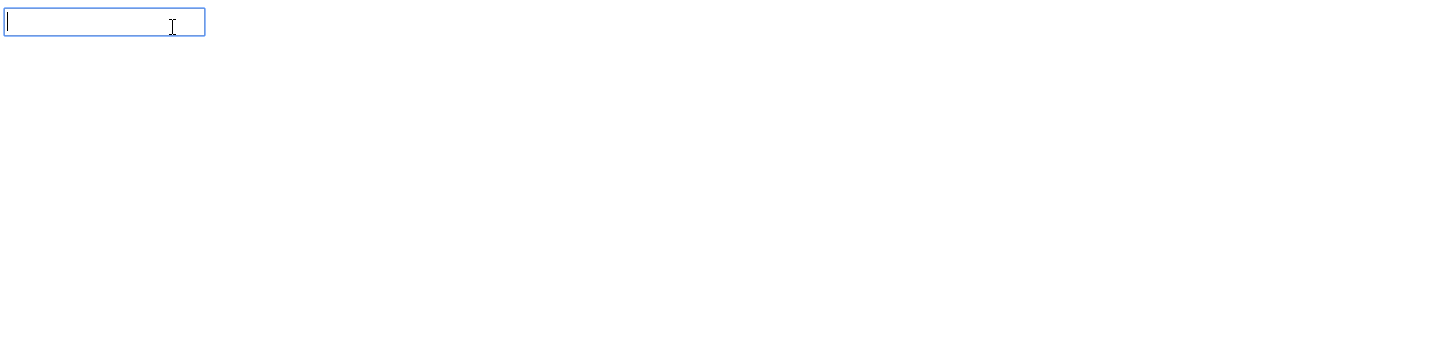
Every time spacebar is triggered the value of text variable is updated.
- (keyup.enter)
(keyup.enter) event is used to generate event when Enter key is pressed. lets take above example just changing the (keyup) to (keyup.enter).
Example Implementation
component.html file
< input (keyup.enter)="onKeyUp($event)">
< p >{{text}}</ p >
|
component.ts file
import { Component, OnInit } from '@angular/core' ;
@Component({
selector: 'app-txtchk' ,
templateUrl: './txtchk.component.html' ,
styleUrls: [ './txtchk.component.css' ]
})
export class TxtchkComponent implements OnInit {
text = '' ;
ngOnInit(): void {
}
onKeyUp(x) {
this .text += x.target.value + ' | ' ;
}
}
|
Output
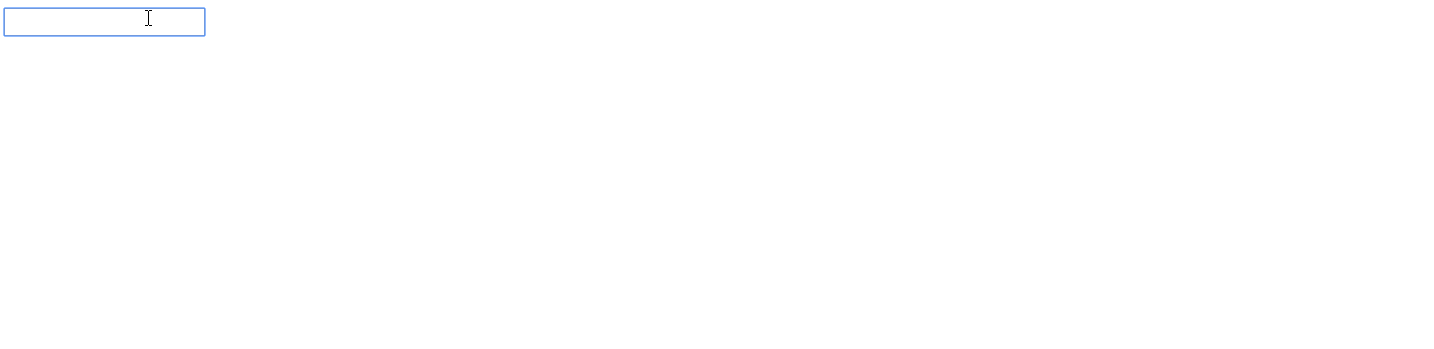
Every time enter is triggered the value of text variable is updated.
- (keyup.a(anyCustomCharacter))
This event is triggered when you press any character you have mentioned in keyup event.Character can be (A-Z, a-z, 0-9 or any other special character). exp. (keyup.z): Here change will be displayed on z character triggered.
Taking same code just changing (keyup) to (keyup.z).
Example Implementation
component.html file
< input (keyup.z)="onKeyUp($event)">
< p >{{text}}</ p >
|
component.ts file
import { Component, OnInit } from '@angular/core' ;
@Component({
selector: 'app-txtchk' ,
templateUrl: './txtchk.component.html' ,
styleUrls: [ './txtchk.component.css' ]
})
export class TxtchkComponent implements OnInit {
text = '' ;
ngOnInit(): void {
}
onKeyUp(x) {
this .text += x.target.value + ' | ' ;
}
}
|
Output
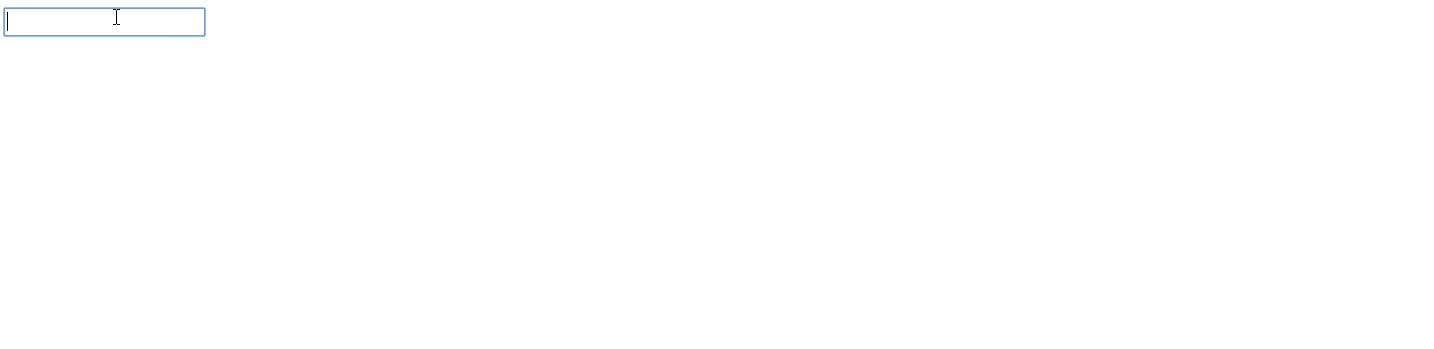
Every time z is triggered the value of text variable is updated.
- (keyup.esc)
(keyup.esc) event is used to generate event when esc key is pressed. lets take above example just changing the (keyup) to (keyup.esc).
Example Implementation
component.html file
< input (keyup.esc)="onKeyUp($event)">
< p >{{text}}</ p >
|
component.ts file
import { Component, OnInit } from '@angular/core' ;
@Component({
selector: 'app-txtchk' ,
templateUrl: './txtchk.component.html' ,
styleUrls: [ './txtchk.component.css' ]
})
export class TxtchkComponent implements OnInit {
text = '' ;
ngOnInit(): void {
}
onKeyUp(x) {
this .text += x.target.value + ' | ' ;
}
}
|
Output
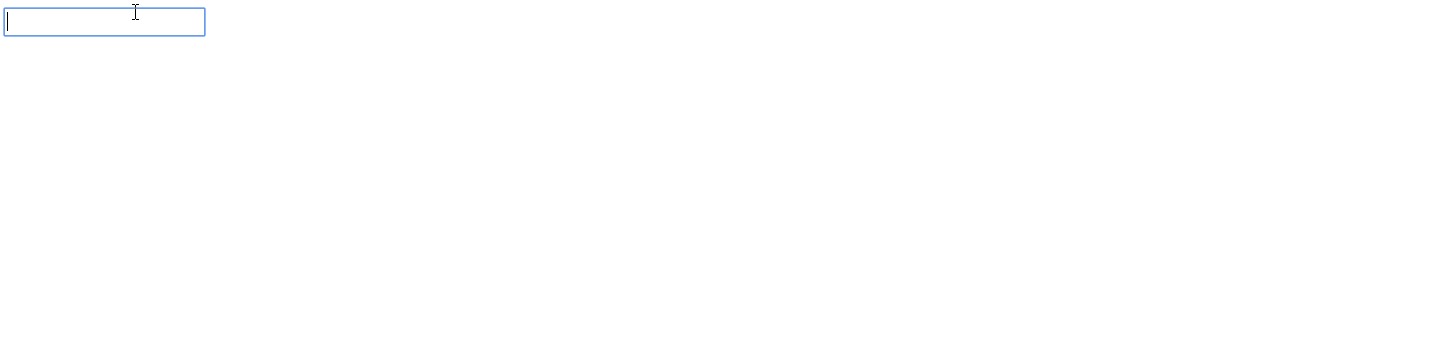
Every time esc key is triggered the value of text variable is updated.
Similar to above there are many other options that can be used with (keyup)
- (keyup.shift)
(keyup.shift) event is used to generate event when shift key is pressed.
Example Implementation
component.html file
< input (keyup.shift)="onKeyUp($event)">
< p >{{text}}</ p >
|
component.ts file
import { Component, OnInit } from '@angular/core' ;
@Component({
selector: 'app-txtchk' ,
templateUrl: './txtchk.component.html' ,
styleUrls: [ './txtchk.component.css' ]
})
export class TxtchkComponent implements OnInit {
text = '' ;
ngOnInit(): void {
}
onKeyUp(x) {
this .text += x.target.value + ' | ' ;
}
}
|
Output
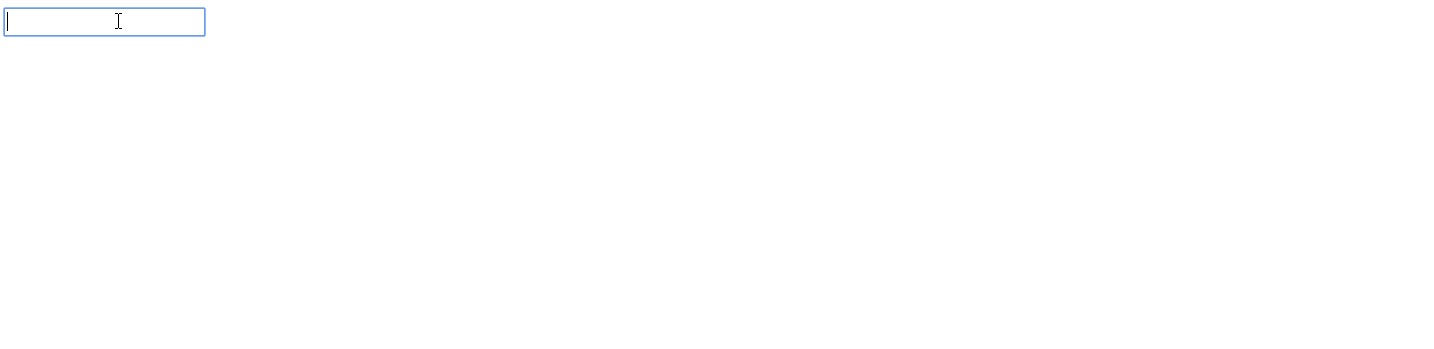
- (keyup.control)
(keyup.control) event is used to generate event when control key is pressed.
Example Implementation
component.html file
< input (keyup.control)="onKeyUp($event)">
< p >{{text}}</ p >
|
component.ts file
import { Component, OnInit } from '@angular/core' ;
@Component({
selector: 'app-txtchk' ,
templateUrl: './txtchk.component.html' ,
styleUrls: [ './txtchk.component.css' ]
})
export class TxtchkComponent implements OnInit {
text = '' ;
ngOnInit(): void {
}
onKeyUp(x) {
this .text += x.target.value + ' | ' ;
}
}
|
Output
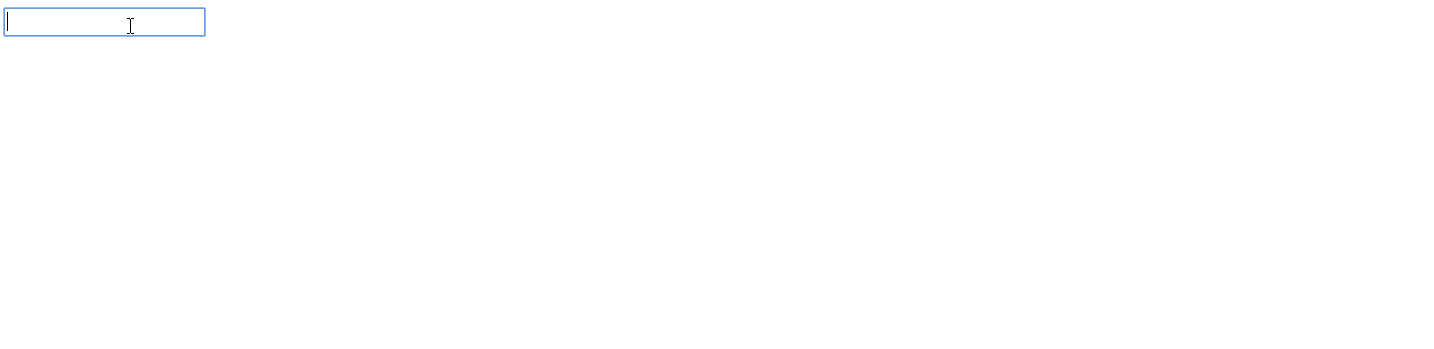
- (keyup.alt)
(keyup.alt) event is used to generate event when alt key is pressed.
Example Implementation
component.html file
< input (keyup.alt)="onKeyUp($event)">
< p >{{text}}</ p >
|
component.ts file
import { Component, OnInit } from '@angular/core' ;
@Component({
selector: 'app-txtchk' ,
templateUrl: './txtchk.component.html' ,
styleUrls: [ './txtchk.component.css' ]
})
export class TxtchkComponent implements OnInit {
text = '' ;
ngOnInit(): void {
}
onKeyUp(x) {
this .text += x.target.value + ' | ' ;
}
}
|
Output
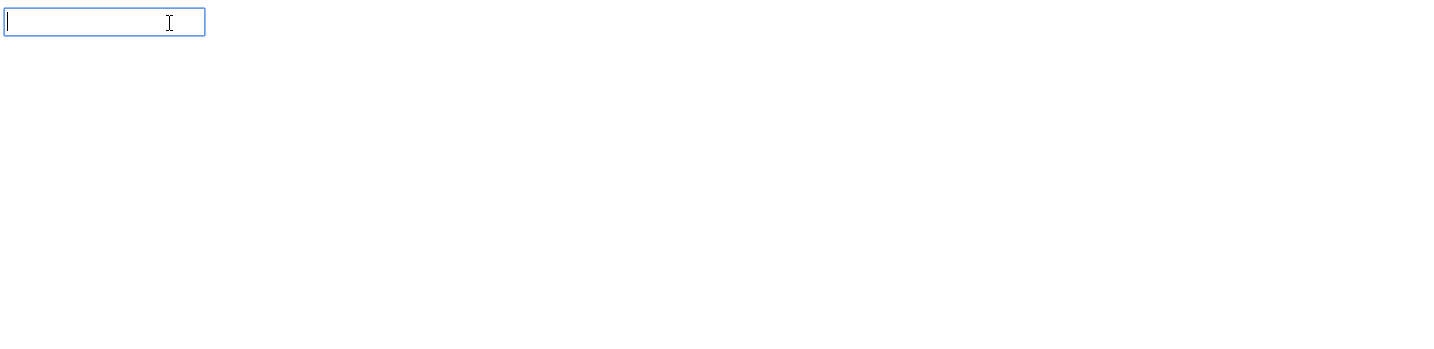
- (keyup.backspace)
(keyup.backspace) event is used to generate event when backspace key is pressed . Its basic syntax is:-
Example Implementation
component.html file
< input (keyup.backspace)="onKeyUp($event)">
< p >{{text}}</ p >
|
component.ts file
import { Component, OnInit } from '@angular/core' ;
@Component({
selector: 'app-txtchk' ,
templateUrl: './txtchk.component.html' ,
styleUrls: [ './txtchk.component.css' ]
})
export class TxtchkComponent implements OnInit {
text = '' ;
ngOnInit(): void {
}
onKeyUp(x) {
this .text += x.target.value + ' | ' ;
}
}
|
Output
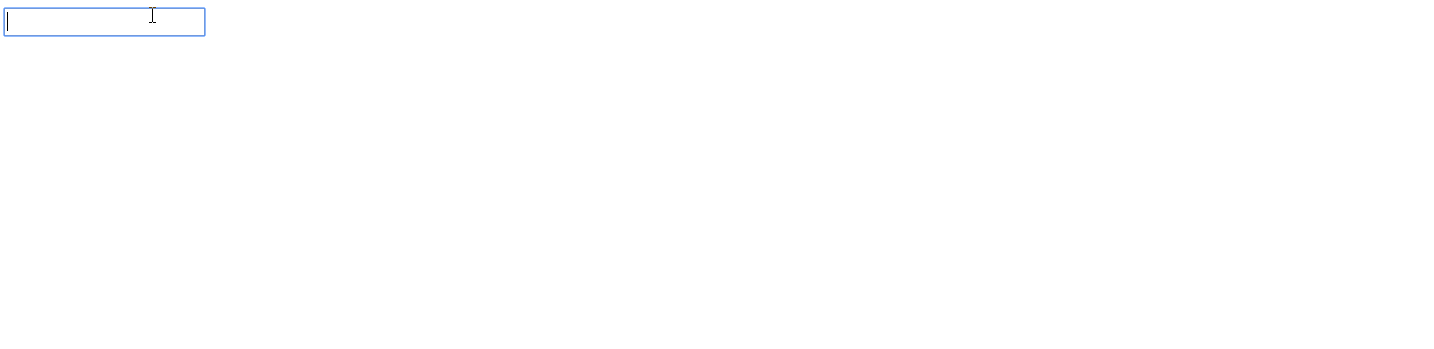
- (keyup.arrowup)
(keyup.arrowup) event is used to generate event when arrowup key is pressed.
Example Implementation
component.html file
< input (keyup.arrowup)="onKeyUp($event)">
< p >{{text}}</ p >
|
component.ts file
import { Component, OnInit } from '@angular/core' ;
@Component({
selector: 'app-txtchk' ,
templateUrl: './txtchk.component.html' ,
styleUrls: [ './txtchk.component.css' ]
})
export class TxtchkComponent implements OnInit {
text = '' ;
ngOnInit(): void {
}
onKeyUp(x) {
this .text += x.target.value + ' | ' ;
}
}
|
Output
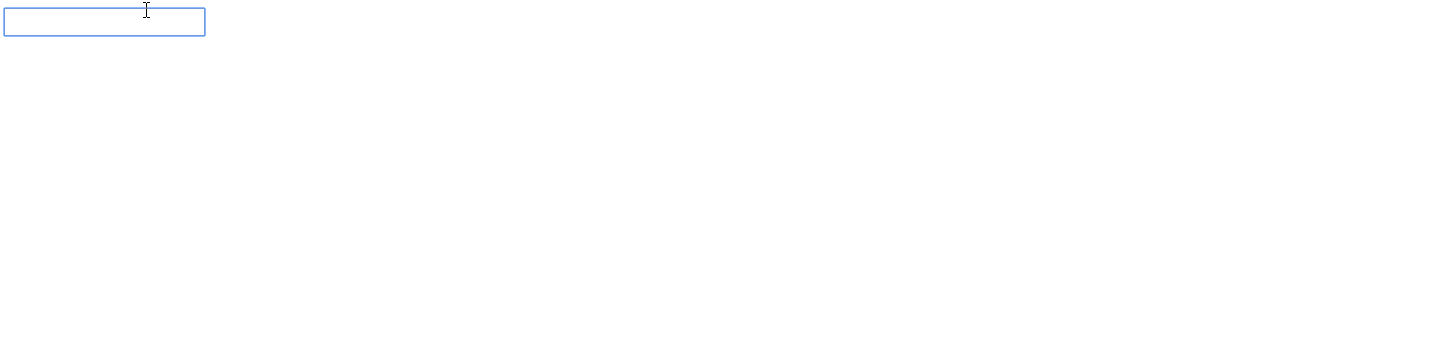
Hence, (keyup) can be used with many options, all their implementations are nearly same, just usage differs to the option used.
Share your thoughts in the comments
Please Login to comment...