p5.js log() function
Last Updated :
23 Aug, 2023
The log() function in p5.js is used to get the natural logarithm (of base “e”) of any number taken as input for the parameter of log() function.
Syntax:
log(x)
Parameters: This function accepts a single parameter x which is any number greater than zero (0) taken as the input whose natural log is going to be calculated.
Return Value: It returns the natural log of any input number greater than zero (0).
Below program illustrates the log() function in p5.js:
Example: This example uses log() function to get the natural log value of any input number.
Javascript
function setup() {
createCanvas(550, 130);
}
function draw() {
background(220);
let a = 5;
let b = 7.7;
let c = 0;
let d = -5;
let v = log(a);
let w = log(b);
let x = log(c);
let y = log(d);
textSize(16);
fill(color( 'red' ));
text( "Natural logarithm value of 5 is : " + v, 50, 30);
text( "Natural logarithm value of 7.7 is : " + w, 50, 50);
text( "Natural logarithm value of 0 is : " + x, 50, 70);
text( "Natural logarithm value of -5 is : " + y, 50, 90);
}
|
Output:
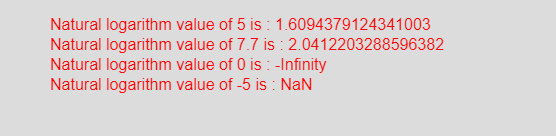
Note: If we take input as a negative value and zero then it returns the output as “NaN” and -Infinity respectively.
Reference: https://p5js.org/reference/#/p5/log
Share your thoughts in the comments
Please Login to comment...