Microprocessor Tutorials
Last Updated :
22 Apr, 2024
A microprocessor is fabricated on a single integrated circuit (IC) or chip that is used as a central processing unit (CPU). It is used as an electronic device, giving output instructions and executing data. In the microprocessor tutorial page, We will cover some basic topics like the introduction to microprocessors, what are microprocessors, 8085 and 8086 programs, I/O interfacing, microcontrollers, and Peripheral devices.
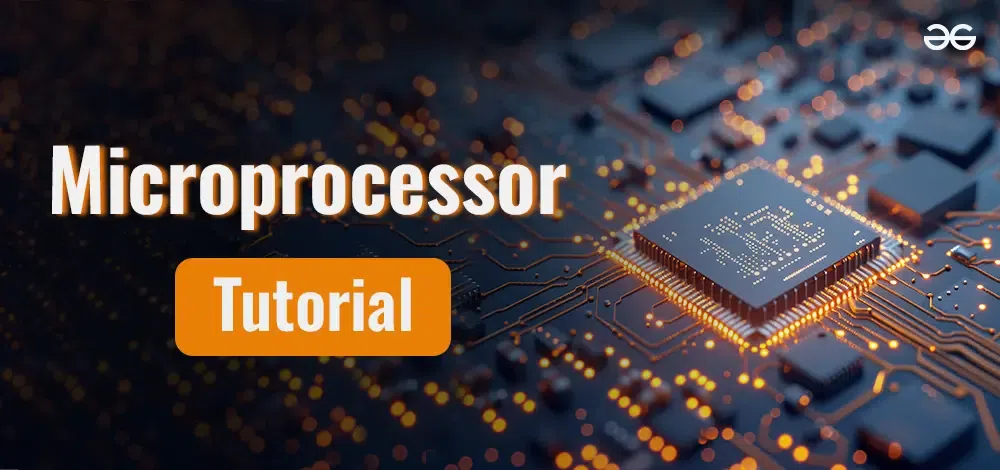
What is a Microprocessor?
A microprocessor is a concise electronic device comprised of semiconductor elements that is fabricated on a single integrated circuit (IC) or chip which is used as a central processing unit (CPU) that works as a brain of any Electronic Component so it can be used to Process Data is called a Microprocessor. It is used to give output instructions and execute data.
A microprocessor is a form of computer processor comprising a single integrated circuit, responsible for executing logic functions and controlling data processing operations. It integrates the logic, arithmetic, and control circuitry essential for fulfilling the duties of a computer’s central processing unit within a compact architecture.
Introduction to Microprocessor
The Central processing unit (CPU) which is used to work as a brain of any Electronic Component that is used to Process Data is called a Microprocessor. A huge amount of Electronic Components such as Transistors, Capacitors, and Resistors are fabricated on a Single chip. All the Components together perform various Operations such as Arithmetic, Logical, Control, and Input / Output that are essential to executing Instructions and processing Data. This tutorial will discuss the architecture, pin diagram, and other key areas of microprocessors.
8085 Microprocessor
The 8085 microprocessor is used as an 8-bit that was developed by Intel in 1976. This type of microprocessor was designed for general-purpose computing and is most essential to develop early computers. The 805 architecture works as an 8-bit architecture that is used to process data in 8-bit portions at a time. It also features a 16-bit address bus, allowing it to access up to 64 KB of memory.Â
8086 Microprocessor
The 8086 microprocessor was developed by Intel in 1978. It is involved in continuous improvements in the areas of microprocessors. The 8086 architecture, features a 16-bit that is used to process data in 16-bit portions at a time. The 8086 consists of advanced instruction set, and includes more memory that divides the memory into segments.Â
I/O Interfacing
I/O interfacing consists of various external devices such as sensors, displays, and actuators that are used in external devices which connects to the microprocessor to enable communication and data exchange in I/O Interfacing. The main purpose of I/O interfacing is to enable communication between the microprocessor and external devices.
- Memory and I/O Interfacing
- I/O Interfacing
- 8279 Block Diagram
- Pin Diagram of 8279
- 8257 DMA Controller
- Working of the 8257 DMA controller
- What is a Serial Communications Interface (SCI)?
- Parallel Communication Interface
- Serial vs Parallel Communication
Microcontroller
A microcontroller is a concise integrated circuit (IC) that is used to combine a microprocessor with memory (volatile and non-volatile memories), input or output peripherals, and also some essential components that are involved to perform particular tasks in interfacing with the microcontroller. It works as a brain of the device, where it is designed to execute specific tasks within an embedded systems.
Peripheral Devices
Peripheral devices are referred to as External hardware components that interface with the CPU to provide information, produce results, or carry out specific tasks. An essential component of various electronic systems and applications, peripheral devices allow microprocessors to function more effectively and allow devices to communicate with the external environment.
Difference between
In computing devices, the three terms Microprocessor, microcontroller and microcomputer which relates to the differences with respect to their design, functionality, and applications. Microprocessors are used as the processing unit of a computer system, where microcontrollers can be used to integrate processing and control functions for embedded systems, and microcomputers are used to execute instructions in the computer systems that assembled surrounding microprocessors.
Conclusion
Well, in the end of this Microprocessor tutorial. In this complete guide of microprocessor we’ve explored how microprocessors work, how it fetch instructions, process data, and execute complex tasks. Dive deeper into specific processor architectures, or delve into how microprocessors work in harmony with other components. The path to mastering computer science is an exciting adventure – keep learning, keep exploring, and keep pushing the boundaries of your knowledge!
Microprocessor Tutorial – FAQ
Does this microprocessor tutorial good for beginner
Yes, this microprocessor tutorial is best for beginners as well for the professionals
What is the importance of microprocessors?
Microprocessors are essential components of modern computing systems, powering everything from personal computers and smartphones to embedded systems and IoT devices. They enable efficient processing of data and execution of complex tasks, driving technological advancements in various fields.
What are the future trends in microprocessor technology?
Future trends in microprocessor technology include the development of more powerful and energy-efficient processors, integration of specialized accelerators for tasks like artificial intelligence and machine learning, advancements in semiconductor manufacturing processes such as EUV lithography, and the proliferation of heterogeneous computing architectures combining CPUs, GPUs, and other accelerators for improved performance.
What are the components of a microprocessor?
The main components of a microprocessor include the arithmetic logic unit (ALU), control unit, registers, and input/output interfaces. The ALU performs arithmetic and logical operations, the control unit manages the execution of instructions, registers store data temporarily, and input/output interfaces connect the microprocessor to external devices.
How does a microprocessor work?
Microprocessors work by fetching instructions from memory, decoding them, executing the operations specified by the instructions, and then storing the results back in memory. This process is performed continuously, allowing the microprocessor to carry out complex tasks at high speeds. Microprocessors use a combination of electronic components, including transistors and logic gates, to perform these operations.
Which language is good for microprocessor?
Due to low-level of access to hardware and provide high performance C and C++ are the popular programming language for microcontroller.
Share your thoughts in the comments
Please Login to comment...