Implementing Lazy Loading in Angular
Last Updated :
01 Apr, 2024
Angular applications often consist of various modules and components, which can result in longer initial load times, especially for larger applications. To address this issue and improve performance, Angular provides lazy loading—a technique that defers the loading of certain modules until they are needed.
In this article, we’ll learn more about lazy loading in Angular and how we can implement it in our project.
What is Lazy Loading?
Lazy loading is a strategy used to optimize the loading time of an Angular application by loading modules only when they are required. Instead of loading all modules and their associated components at once during the initial application load, lazy loading allows modules to be loaded asynchronously as the user navigates to the corresponding routes. This results in faster initial load times and improved overall performance, especially for larger applications with complex routing structures.
Why Use Lazy Loading ?
It offers several benefits, which includes:
- Improved initial load time: By loading only the required components initially, the application’s initial load time is reduced which results in a better user experience.
- Reduced memory footprint: Since only the required components are loaded, the application’s memory footprint is smaller, leading to better overall performance.
- Better code organization: It encourages modular code organization, making it easier to maintain and scale the application.
It plays a crucial role in optimizing Angular applications for speed performance, and scalability, which enhances user experience.
How to Lazy Load Routes ?
Step 1: Create a new Angular application using the following command.
ng new sample-app
cd sample-app
Step 2: Create the 4 components using the following commands.
ng g c home
ng g c services
ng g c about-us
ng g c contact-us
Folder Structure:
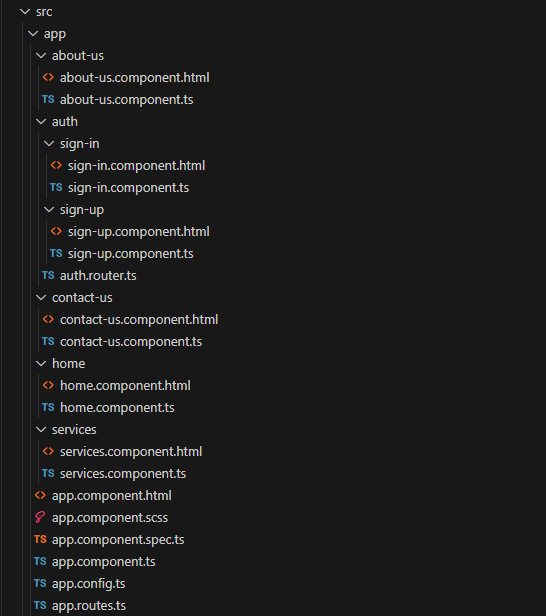
Dependencies:
"dependencies": {
"@angular/animations": "^17.3.0",
"@angular/common": "^17.3.0",
"@angular/compiler": "^17.3.0",
"@angular/core": "^17.3.0",
"@angular/forms": "^17.3.0",
"@angular/platform-browser": "^17.3.0",
"@angular/platform-browser-dynamic": "^17.3.0",
"@angular/router": "^17.3.0",
"rxjs": "~7.8.0",
"tslib": "^2.3.0",
"zone.js": "~0.14.3"
}
Now we can update our app.router.ts file to add the actual routes. Also don’t forget to add router-outlet tag in the app.component.html and import RouterOutlet to app.component.ts. Finally, we can add the buttons to handle navigation between different routes, for this to work we will need to import the RouterLink.
With Angular 16+, it has become much simpler to lazy load any route, we can make use of the loadComponent property to load a component or loadChildren to load nested routes.
In the below example, we have four components, out of which home and services are loaded initially with the app. While Contact Us and About Us component are loaded lazily.
HTML
<!-- app.component.html -->
<button routerLink="home">Home</button>
<button routerLink="services">Services</button>
<button routerLink="about-us">About Us</button>
<button routerLink="contact-us">Contact Us</button>
<router-outlet></router-outlet>
JavaScript
//app.router.ts
import { Routes } from '@angular/router';
export const routes: Routes = [
{
path: '',
redirectTo: 'home',
pathMatch: 'full',
},
{
path: 'home',
component: HomeComponent
},
{
path: 'services',
component: ServicesComponent,
},
{
path: 'about-us',
loadComponent: () => import('./about-us/about-us.component').then((c) => c.AboutUsComponent)
},
{
path: 'contact-us',
loadComponent: () => import('./contact-us/contact-us.component').then((c) => c.ContactUsComponent)
}
]
JavaScript
// app.component.ts
import { Component } from '@angular/core';
import { RouterLink, RouterOutlet } from '@angular/router';
@Component({
selector: 'app-root',
standalone: true,
imports: [RouterOutlet, RouterLink],
templateUrl: './app.component.html',
styleUrl: './app.component.scss'
})
export class AppComponent {
title = 'sample-app';
}
Output:
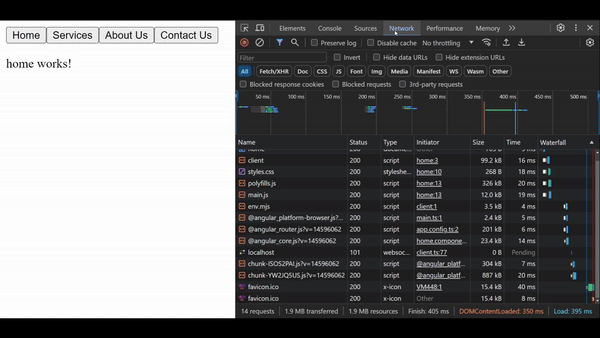
Lazy loading Angular routes
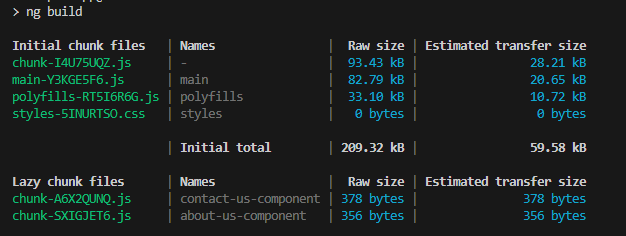
Angular application build output
We can see the output of our build process, contact-us-component and about-us-component are loaded into different output file each so that they can be served only when required.
How to Lazy Load Nested Routes ?
For nested routes, we can use the loadChildren property instead of loadComponent, so that they are loaded only when required.
Using below commands we can create sign-in and sign-up components, and add an auth.router.ts to handle routing for auth components.
ng g c auth/sign-in
ng g c auth/sign-up
JavaScript
// app.router.ts
import { Routes } from '@angular/router';
export const routes: Routes = [
{
path: '',
redirectTo: 'auth',
pathMatch: 'full',
},
{
path: 'auth',
loadChildren: () => import('./auth/auth.router')
.then(m => m.AuthRoutes)
}
];
JavaScript
// auth.router.ts
import { Routes } from '@angular/router';
export const AuthRoutes: Routes = [
{
path: '',
redirectTo: 'sign-in',
pathMatch: 'full',
},
{
path: 'sign-in',
loadComponent: () =>
import('./sign-in/sign-in.component')
.then((c) => c.SignInComponent)
},
{
path: 'sign-up',
loadComponent: () =>
import('./sign-up/sign-up.component')
.then((c) => c.SignUpComponent)
}
];
Output:
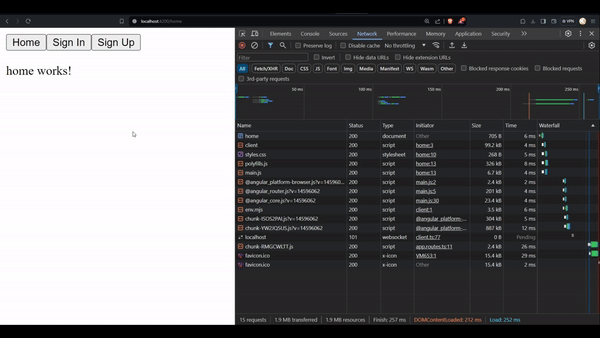
Lazy loading Angular nested routes
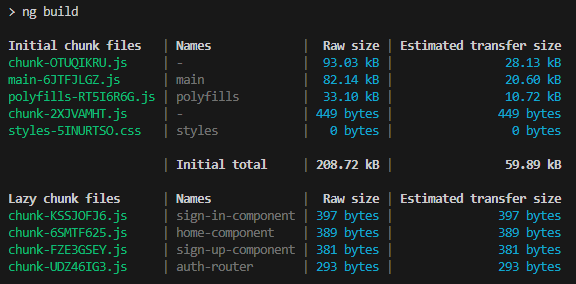
Angular application build output
Here when we visit auth/sign-in we observe that two chunks are loaded, that is because we had lazy loaded our nested routes and also the sign-in route. Also in the build output we have different chunks for each of our lazy loaded asset.
Share your thoughts in the comments
Please Login to comment...