How To handle Loading States & Asynchronous Dependencies in Lazy Loaded Components ?
Last Updated :
28 Mar, 2024
To handle loading states and asynchronous dependencies we can use Suspense Component provided by React. When we load a component lazily, it loads only when it’s needed and it takes some time to load it. During this period, when a component is loading there must be a fallback UI in place of it like a loading spinner or any other placeholder content like a Shimmer UI.
Steps to Setup React App:
Step 1: Create a React application and Navigate to the project directory with the following command:
npx create-react-app my-project
cd my-project
Project Structure:
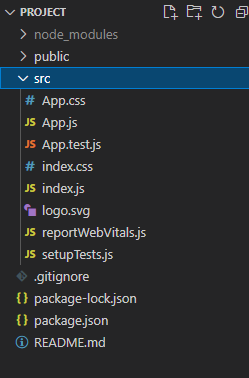
Project Structure
The updated dependencies in package.json file will look like.
"dependencies": {
"react": "^18.2.0",
"react-dom": "^18.2.0",
}
Handling loading states in lazy loaded components?
First, we need to load a component lazily after that we can use Suspense Component where was can pass fallback UI as a prop and inside Suspense Component we can render our lazy loaded component.
Example: Below is the code example
JavaScript
// App.js
import { Suspense, lazy } from "react";
const MyLazyComponent = lazy(() => import("./MyLazyComponent"));
const App = () => {
return (
// loading state is shown while the component is being loaded
<Suspense fallback={<div>Loading...</div>}>
<MyLazyComponent />
</Suspense>
);
};
export default App;
JavaScript
// MyLazyComponent.js
const MyLazyComponent = () => {
return (
<div>MyLazyComponent</div>
)
}
export default MyLazyComponent;
Output:
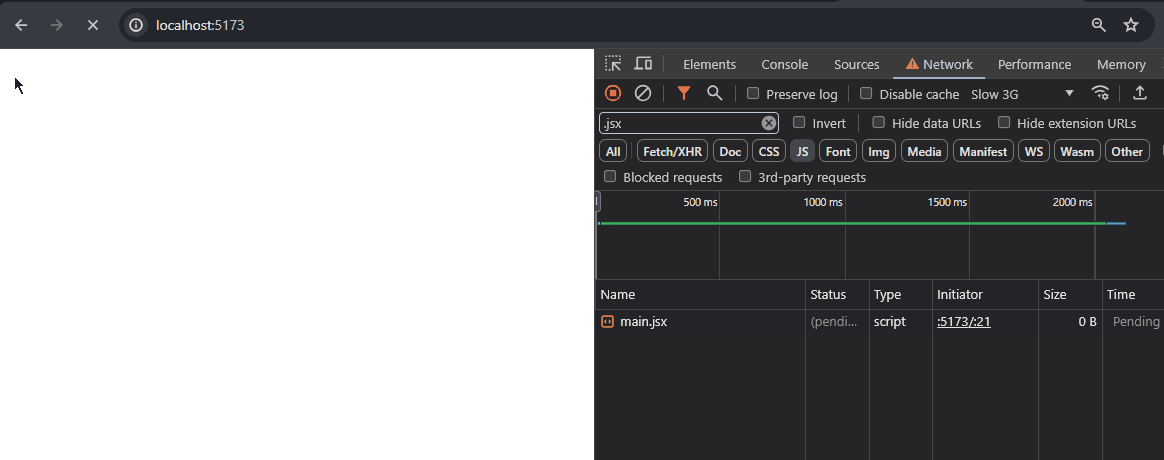
Loading States
Handling asynchronous dependencies in lazy loaded component?
First, we need to load a component lazily, since we need an asynchronous dependency we will fetch some random data and when that data gets loaded we will load the lazy loaded component. That means our lazy loaded component is dependent on some asynchronous task.
Example: Below is the code example:
JavaScript
//App.js
import {
Suspense, lazy,
useEffect, useState
} from "react";
const MyLazyComponent =
lazy(() => import("./MyLazyComponent"));
const App = () => {
const [userName, setUserName] = useState("");
useEffect(() => {
async function fetchUserName() {
try {
const response = await fetch(
"https://api.github.com/users/Tapesh-1308"
);
if (!response.ok) {
throw new Error("Failed to fetch data");
}
const userData = await response.json();
setUserName(userData.name);
} catch (error) {
console.error("Error fetching data:", error);
}
}
fetchUserName();
}, []);
return (
<Suspense fallback={<div>Loading...</div>}>
{
userName !== "" &&
<MyLazyComponent userName={userName} />
}
</Suspense>
);
};
export default App;
JavaScript
//MyLazyComponent.js
const MyLazyComponent = ({ userName }) => {
return (
<div>
UserName: {userName}
</div>
)
}
export default MyLazyComponent
Output:
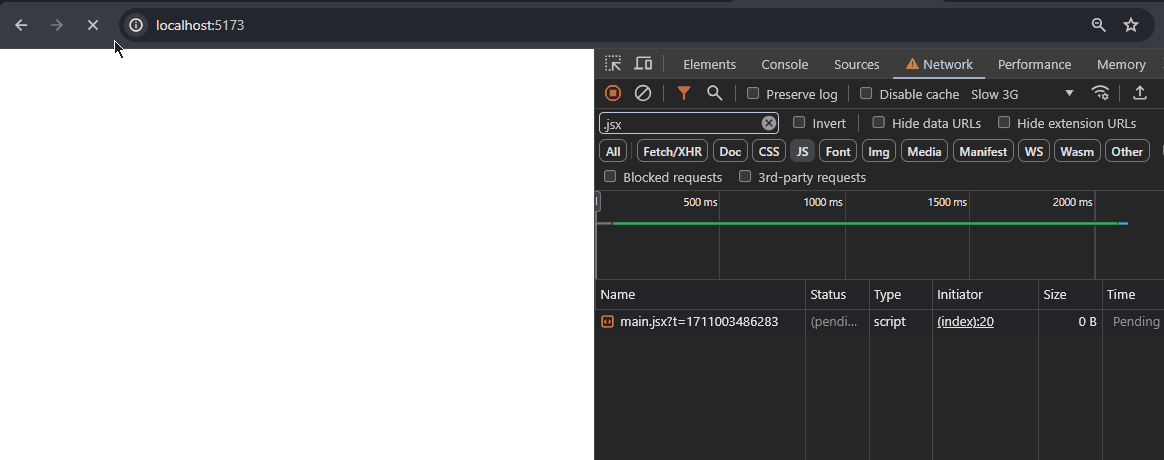
Asynchronous Dependencies
Share your thoughts in the comments
Please Login to comment...