Lazy Loading in React and How to Implement it ?
Last Updated :
24 Nov, 2023
Lazy Loading in React JS helps to optimize the performance of React applications. The data is only rendered when visited or scrolled it can be images, scripts, etc. Lazy loading helps to load the web page quickly and presents the limited content to the user that is needed for the interaction lazy loading can be more helpful in applications that have high-resolution images or data that alters the loading time of the application.
Lazy Loading in React:
Lazy loading is a technique in React that allows you to load components, modules, or assets asynchronously, improving the loading time of your application. React provides a built-in React.lazy() method and Suspense component to achieve lazy loading.
Syntax for Lazy Loading:
// Implement Lazy Loding with React.Lazy method
const MyComponent = React.lazy(() => import('./MyComponent'));
Steps to Implement Lazy Loading:
- Recognize the component you want to Lazy Load. These are mostly Large or complex which is not necessary for all the users when the page loads.
- Import the `lazy()` and Suspense components from the React package
- Use the `lazy()` function to dynamically import the component you want to lazy load:
Note that the argument to the `lazy()` function should be a function that returns the result of the import() function.
- Wrap the lazy-loaded component in a `Suspense` component, which will display a fallback UI while the component is being loaded:
<Suspense fallback={<div>Loading...</div>}>
<MyComponent />
</Suspense>
Example: This example uses the Suspense component and lazy method to implement the lazyloading for specific components.
Javascript
import React from "react" ;
import { Suspense, lazy } from "react" ;
const Component1 = lazy(() => import(
'../src/LazyContent/myComponent1' ))
const Component2 = lazy(() => import(
'../src/LazyContent/myComponent2' ))
function App() {
return (
<>
<h1> Lazy Load</h1>
<Suspense fallback=
{<div>Component1 are loading please wait...</div>}>
<Component1 />
</Suspense>
<Suspense fallback=
{<div>Component2 are loading please wait...</div>}>
<Component2 />
</Suspense>
</>
);
}
export default App;
|
Output:
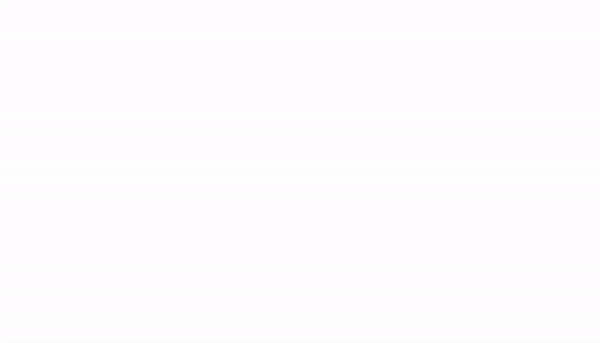
Lazy-Loading
Explanation:
Lazy Loading in React can be implemented with the help of the built-in function `React. lazy()`. This is also known as code splitting, In which `React.lazy` along with webpack bundler divides the code into separate chunks, when the component is requested the chunk is loaded on demand. The use of React Suspense is to define fallback content to be displayed during asynchronous components or data loading, as shown above. React Suspense provides better feedback to the user and improves the user experience as a user is not facing any blank screen or space while the content is being loaded. React Suspense is designed to handle the loading of the components that make asynchronous API requests. React Suspense can be used by wrapping the `<Suspense>` component and specifying the fallback content displayed while the component or data is loading.
Code-splitting: It is an effective technique for optimizing the performance and efficiency of web applications, especially those with large code bases. By reducing the amount of code that needs to be loaded when a page first loads, code splitting can improve the user experience and make your application more responsive and fast.
Advantages of using Lazy loading in react applications:
- Lazy loading allows you to use server resources more efficiently by loading only the resources you need. This is very important for high-traffic applications or when server resources are tight.
- A quicker initial load time can be achieved by using lazy loading, which minimizes the amount of code that must be downloaded and parsed when the page first loads. This can speed up your application’s first load time greatly.
Conclusion:
React Lazy Loading is a powerful technique that significantly improves the performance of web applications built with React. One of the key benefits of lazy loading is that it can help improve the Time to Interactive (TTI) metric, which is the time it takes for a page to become interactive and responsive. By delaying the loading of non-critical components until the page has finished loading, lazy loading reduces TTI and provides a more engaging user experience.
Share your thoughts in the comments
Please Login to comment...