How to use the Context API to avoid prop drilling?
Last Updated :
06 Feb, 2024
This article demonstrates the use of Context API to avoid prop drilling in React. We will learn about the following concepts:
What are Props ?
Props are basically information (properties) that is passed from the parent component to other components (children) in React.They are objects that are used to share and communicate data between different components in a react application.
What is Prop Drilling ?
Prop drilling is the situation where developers pass props (data) to multiple levels of components until the component where required data is reached. For example, if we have a GrandParent, a Parent and a Child component and we need data pass the GrandParent component to the Child component then using prop drilling we need to pass the data from GrandParent component to the Parent component and from the parent component to the child component.Prop drilling leads to several issues, such as an increase in the complexity of the project and a performance issue as passing down large objects into several components decreases the overall performance.
Context API:
To address the issue of prop drilling contextAPI is used. It allows the developers to easily share the information with different components that are not directly connected.
contextAPI consists of two properties that is provider and consumer. The provider provides a state to the children, and the consumer uses the state that has been passed.
Use the Context API to Avoid Prop drilling:
- Create Context: Use
createContext
to define a context.
- Wrap Component Tree: Wrap your component tree with
Context Provider
. Pass the shared state or values as the value
prop.
- Access Context Value: In child components,
useContext
to access the context value.
- Avoid Prop Drilling: This allows components deep in the tree to access shared state without passing props through intermediary components.
Steps to Create Project:
Step 1: Create React application:
npx create-react-app my-app
Step 2: Navigate to the root folder by using the command:
cd my-app
Step 3: Create two files ChildA.js and ChildB.js in the src folder as shown
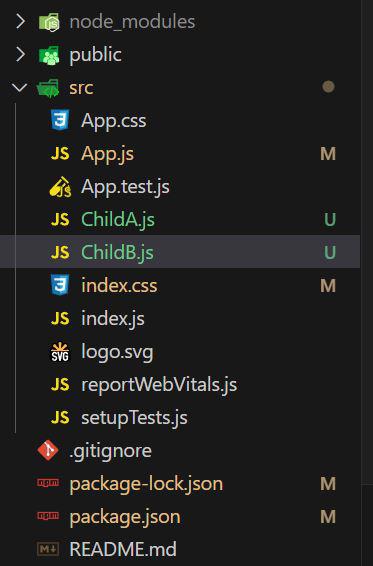
Approach:
- Create a context in App.js to manage the shared data.
- Set up a provider in App.js using the created context.
- Wrap the ChildB component inside the provider, as you intend to pass data only to ChildB.
- Create a consumer inside ChildB.js using the same context.
- Access the shared data using the consumer.
Example: Write the following code in respective files.
Javascript
import './App.css' ;
import React, { createContext } from 'react' ;
import ChildB from './ChildB' ;
const ContextData = createContext();
function App() {
const data = "RootComponent" ;
return (
<div>
<ContextData.Provider value={data}>
<ChildB />
</ContextData.Provider>
</div>
);
}
export default App;
export { ContextData };
|
Javascript
import React from 'react'
import ChildB from './ChildB'
function ChildA() {
return (
<ChildB />
)
}
export default ChildA
|
Javascript
import React from 'react'
import { ContextData } from './App'
function ChildB() {
return (
<div>
<ContextData.Consumer>
{
(data) => {
return <h1>I am from {data}</h1>
}
}
</ContextData.Consumer>
</div>
)
}
export default ChildB;
|
Start your application using the following command:
npm start
Output:
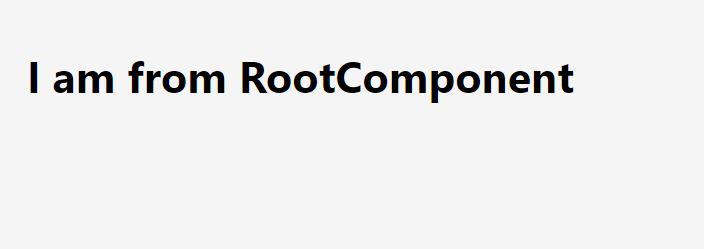
Share your thoughts in the comments
Please Login to comment...