Comparing Redux and Context API in React: A Comprehensive Analysis
Last Updated :
13 Nov, 2023
In this article, we are going to learn about the difference between the Redux and Context APIs. We will cover each of them entirely with their live examples, and then we will cover the differences between them.
Context API
Instead of manually passing props down at the entry-level, you can use the React Context API to transmit data along the component tree. This can be helpful for exchanging information that several components at various levels of the tree need, such as the current user, the current location, or the current theme.
Context API Core Concepts
- createContext function: Context uses the React.createContext() function to create a Context object. This object can then be consumed by components down the tree via the Context.Provider and Context.Consumer.
- Provider component: Provider component makes the context available to the components down the tree. It accepts a value prop to be passed to consuming components.
- consumer component : The consumer component allows consuming the context value. It accepts a function that returns a React node and passes the context value as argument.
Steps to implement Context API.
Step 1: Implement a Context.
Javascript
import React, { createContext } from "react" ;
const Context = createContext({
paragraph: "" ,
});
export default Context;
|
Step 2: Create a Context Provider component:
Javascript
import React, { useState } from "react" ;
import Context from "./Context" ;
const Provider = ({ children }) => {
const [paragraph, setParagraph] = useState( "" );
const value = {
paragraph,
setParagraph,
};
return <Context.Provider value={value}>{children}
</Context.Provider>};
export default Provider;
|
Step 3: Create a Button component that consumes the context:
Javascript
import React, { useContext } from "react" ;
import Context from "./Context" ;
const Button = () => {
const { paragraph, setParagraph } = useContext(Context);
const updateParagraph = () => {
setParagraph( "ReactJS Tutorials" );
};
return (
<div className= "container" >
<h2> Geeksforgeeks</h2>
<h4>{paragraph}</h4>
<div className= "button" >
<button onClick={updateParagraph}>Click Here</button>
</div>
</div>
);
};
export default Button;
|
Step 4: Wrap the all components in app.js and update the App.css file
Javascript
import React from "react" ;
import Provider from "./Provider" ;
import Button from "./Button" ;
import "./App.css"
function App() {
return (
<Provider>
<Button />
</Provider>
);
}
export default App;
|
CSS
.container {
display : flex;
flex- direction : column;
align-items: center ;
justify- content : center ;
height : 100 vh;
}
.button {
margin-top : 20px ;
}
button {
padding : 10px 20px ;
font-size : 16px ;
background-color : #4caf50 ;
color : #ffffff ;
border : none ;
border-radius: 4px ;
cursor : pointer ;
}
button:hover {
background-color : #45a049 ;
}
h 2 {
color : #45a049 ;
}
|
Steps to run application:
Step 1: Open the terminal and type the following command.
npm start
Step 2: Open browser and search the given URL.
http://localhost:3000/
Output:
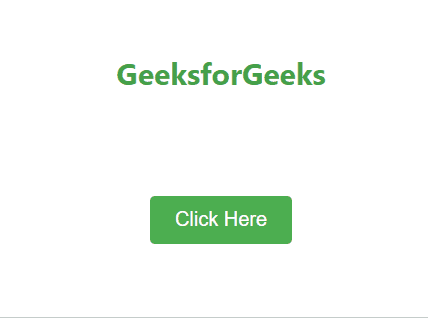
Redux
Redux is a state management library and It is most frequently used for creating user interfaces with libraries like React or Angular. For JavaScript applications, Redux is a predictable state container.
Redux Core Concepts
- Action: Actions are plain JavaScript objects that describe what happened. They contain a type property that indicates the type of action being performed.
- Reducer: Reducers specify how the state tree updates in response to actions. They are pure functions that take the previous state and an action and return the next state.
- Store: The store holds the whole state tree of the application. The store has the state property and methods like dispatch, subscribe, etc.
Steps to implement Redux.
Step 1: Install redux in your project via following command.
npm i redux react-redux
Step 2: Create a store.
Javascript
import { createStore } from 'redux' ;
const initialState = {
count: 0,
};
const counterReducer = (state = initialState, action) => {
switch (action.type) {
case 'INCREMENT' :
return { count: state.count + 1 };
case 'DECREMENT' :
return { count: state.count - 1 };
default :
return state;
}
};
const store = createStore(counterReducer);
export default store;
|
Step 3: Create an action:
Javascript
export const increment = () => ({
type: 'INCREMENT' ,
});
export const decrement = () => ({
type: 'DECREMENT' ,
});
|
Step 4: Create a Counter Component:
Javascript
import React from 'react' ;
import { connect } from 'react-redux' ;
import { increment, decrement } from './actions' ;
import "./App.css"
const Counter = ({ count, increment, decrement }) => (
<div className= 'container' >
<h1>Geeksforgeeks</h1>
<h3>Counter: {count}</h3>
<div className= "button" >
<button onClick={increment}>Increment by 1</button>
<button onClick={decrement} >Decrement by 1</button>
</div>
</div>
);
const mapStateToProps = (state) => ({
count: state.count,
});
export default connect(mapStateToProps, { increment, decrement })(Counter);
|
Step 5: Wrap the all components in index.js and update the App.css file
Javascript
import React from 'react' ;
import ReactDOM from 'react-dom' ;
import { Provider } from 'react-redux' ;
import store from './store' ;
import Counter from './Counter' ;
ReactDOM.render(
<Provider store={store}>
<Counter />
</Provider>,
document.getElementById( 'root' ));
|
CSS
.container {
display : flex;
flex- direction : column;
justify- content : center ;
align-items: center ;
height : 100 vh;
}
.button {
position : relative ;
right : 24px ;
display : flex;
margin-left : 50px ;
margin-top : 20px ;
gap: 20px ;
}
.button button {
padding : 10px 20px ;
font-size : 14px ;
background-color : #4caf50 ;
color : white ;
border : none ;
border-radius: 5px ;
cursor : pointer ;
}
.button button:hover {
background-color : #45a049 ;
}
h 1 {
color : #45a049 ;
}
|
Steps to run application:
Step 1: Open the terminal and type the following command.
npm start
Step 2: Open browser and search the given URL.
http://localhost:3000/
Output: Open a web browser and navigate to http://localhost:3000.
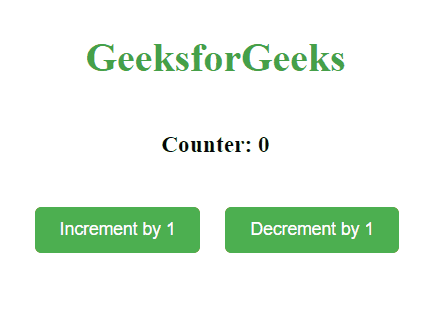
Difference between Redux and Context API:
Feature
|
Redux
|
Context API
|
Middleware
|
Middlewares present.
|
Middlewares absent.
|
State management approach
|
Centralized
|
Decentralized
|
Data Flow
|
Unidirectional flow of data.
|
Bidirectional flow of data.
|
API
|
Actions, reducers, middleware
|
Context.Provider, Context.Consumer
|
Debugging
|
Dedicated Redux development tools for debugging.
|
No tools for debugging.
|
Conclusion:
- Redux is preferred for managing larger and more complex states in applications.
- Context API is more suitable for managing smaller and localized states.
- It’s important to choose the appropriate state management solution based on the specific requirements and complexity of your application.
Share your thoughts in the comments
Please Login to comment...