How does the Provider component make the Redux store available to all components?
Last Updated :
09 Feb, 2024
The Provider component in Redux, provided by the react-redux library, is responsible for making the Redux store available to all components in a React application. It does so by leveraging React’s Context API to propagate the Redux store down the component tree.
Prerequisites:
Steps to Create React Application And Installing Module:
Step 1: Create a React application using the following command:
npx create-react-app foldername
Step 2: After creating your project folder i.e. foldername, move to it using the following command:
cd foldername
Step 3: Installing Redux toolkit:
npm i @reduxjs/toolkit
Step 4: After setting up react environment on your system, we can start by creating an App.jsx file and create a file by the name of components in which we will write our desired function.
Project Structure:
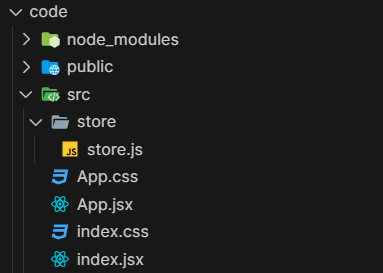
The updated dependencies in package.json file will look like:
"dependencies": {
"@reduxjs/toolkit": "^2.1.0",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-redux": "^9.1.0",
"redux": "^5.0.1"
}
Approach:
Follow the below steps to make the Redux store available to all components how using Provider component:
- Setup Redux Store
- Using the Provider Component from react-redux
- Accessing the Redux Store in Components
- In the index.js file:
- We import the Provider component from react-redux and the Redux store from the store.js file.
- We wrap the root component (<App />) with the Provider component.
- We pass the Redux store as a prop to the Provider component. This makes the store available to all components within the component tree.
- In the App.js file:
- We define the App component, which displays the current count value from the Redux store and provides buttons to increment and decrement the count.
- We use the connect() function from react-redux to connect the App component to the Redux store.
- We define mapStateToProps to map the Redux state to props, and mapDispatchToProps to map dispatch actions to props.
- The connected App component receives the count prop from the Redux store and increment and decrement functions to dispatch actions.
Example: Below is the basic implementation of the above steps:
Javascript
import React from 'react' ;
import { connect } from 'react-redux' ;
const App = (
{
count, increment,
decrement
}) => {
return (
<div>
<h1>Counter: {count}</h1>
<button onClick={increment}>
Increment
</button>
<button onClick={decrement}>
Decrement
</button>
</div>
);
};
const mapStateToProps =
(state) => ({
count: state.count
});
const mapDispatchToProps =
(dispatch) => ({
increment: () => dispatch({ type: 'INCREMENT' }),
decrement: () => dispatch({ type: 'DECREMENT' })
});
export default connect(mapStateToProps, mapDispatchToProps)(App);
|
Javascript
import React from 'react' ;
import ReactDOM from 'react-dom' ;
import { Provider } from 'react-redux' ;
import App from './App' ;
import store from './store/store' ;
ReactDOM.render(
<Provider store={store}>
<App />
</Provider>,
document.getElementById( 'root' )
);
|
Javascript
import { configureStore } from '@reduxjs/toolkit' ;
const initialState = {
count: 0
};
const reducer =
(state = initialState, action) => {
switch (action.type) {
case 'INCREMENT' :
return { ...state, count: state.count + 1 };
case 'DECREMENT' :
return { ...state, count: state.count - 1 };
default :
return state;
}
};
const store = configureStore({ reducer });
export default store;
|
Output:
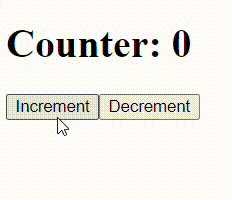
Output
Share your thoughts in the comments
Please Login to comment...