How to make GET call to an API using Axios in JavaScript?
Last Updated :
21 Jul, 2021
Axios is a promise-based HTTP client designed for Node.js and browser. With Axios, we can easily send asynchronous HTTP requests to REST APIs and perform create, read, update and delete operations. It is an open-source collaboration project hosted on Github. It can be imported in plain Javascript or with any library accordingly.
The following script src will include axios.js in the head section of your HTML code
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
When we send a request to the API using axios, it returns a response. The response object consists of:
- data: the data returned from the server.
- status: the HTTP code returned from the server.
- statusText: the HTTP status returned by the server.
- headers: headers obtained from the server.
- config: the original request configuration.
- request: the request object.
For the purpose of demonstration, we will be hosting an API on the localhost:
http://127.0.0.1:5000
Python Script: You will be requiring the following packages to run the API, flask, requests, jsonify, flask_cors. The code for the Python API is as follows:
Python3
from flask import Flask, jsonify, request
from flask_cors import CORS
app = Flask(__name__)
CORS(app)
@app .route( '/test' , methods = [ 'GET' ])
def test():
return jsonify({ "Result" : "Welcome to GeeksForGeeks" })
if __name__ = = '__main__' :
app.run(debug = True )
|
Note: You can host this API by simply running the above python code.
JS Script: Include axios.js and the corresponding JS file in the HTML file. In the JS file, write the following code which makes a GET request using Axios to the API. A GET request to the API requires the path to the API method.
javascript
function makeGetRequest(path) {
axios.get(path).then(
(response) => {
var result = response.data;
console.log(result);
},
(error) => {
console.log(error);
}
);
}
|
- Output: It will call the API with a GET request. The response will be obtained on the console window.
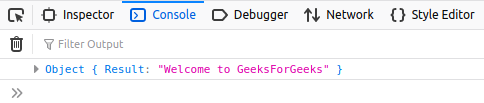
Share your thoughts in the comments
Please Login to comment...