How to access the Value of a Promise in JavaScript
Last Updated :
17 Dec, 2023
In this article, we will see how to access the value of Promise in JavaScript. The Promise is a feature of ES6 introduced in 2015. The concept of Promises is generally used when we want to work asynchronously.
The value of a Promise can be accessed in JavaScript using the following methods.
Syntax:
let promise = new Promise((resolve, reject)=>{//enter the code})
Approach: Using .then( ) Method
- In this code example, we have created a variable createPromise which is a promise having two callbacks provided by javascript.
- resolve: when the promise resolves successfully.
- reject: when the promise is rejected for any reason.
- Then creating setTimeout has a callback function that will run after two seconds.
- By using the .then( ) method access the value of the Promise.
Example: The code example shows how to access the value of a Promise in JavaScript using the .then method.
Javascript
console.log( "wait for 2 seconds to get promise resolved" )
const createPromise = new Promise(
(resolve, reject) => {
setTimeout( function () {
resolve(
"the promise is resolved after 2 seconds" );
}, 2000)
});
createPromise.then((promisedata) => {
console.log( "Promise is resolved successfully" , promisedata)
})
|
Output:
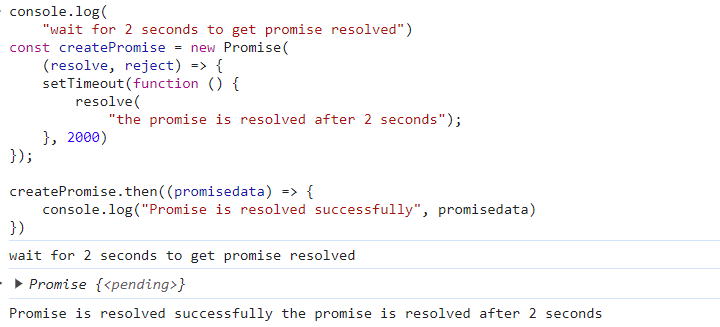
access the Value of a Promise in JavaScript Using .then Method
Approach 2: Using the Async/Await Method
- An async function looks like simple JavaScript function but it has the feature of working with await expressions.
- promisefun is a async function and in the body of that function there is an await expression that waits promise to resolve once it resolved the user access the value of the promise.
- We have used the setTimeout and in callback function we have resolve the promise with the string after two seconds.
- Then, call the async function as promiseFun().
Example: The example show access the value of a Promise in JavaScript using async/await method.
Javascript
console.log( "wait for 2 seconds to get promise resolved" )
async function promiseFun() {
const createPromise = new Promise(
(res, rej) => {
setTimeout( function () {
res(
"the promise resolved" );
}, 2000)
});
const waitPromise = await createPromise
console.log(waitPromise)
}
promiseFun()
|
Output:
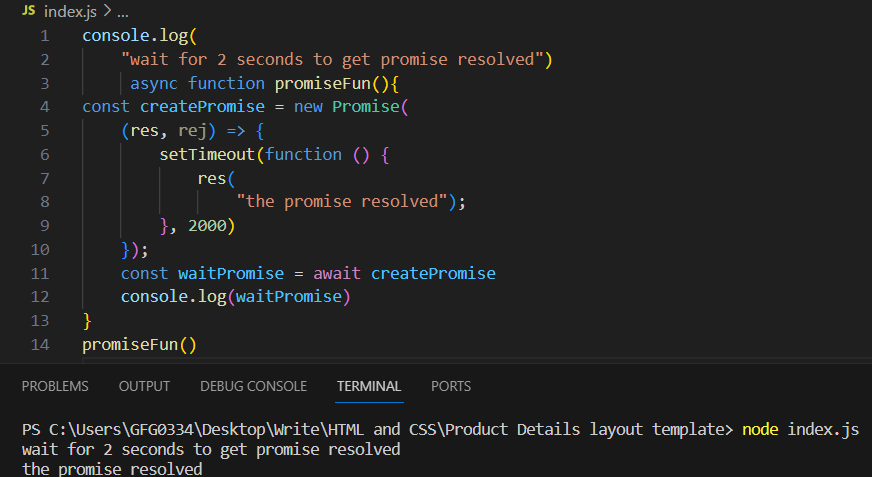
Output
Share your thoughts in the comments
Please Login to comment...