How to scroll to an element inside a div using javascript?
Last Updated :
15 Dec, 2023
To scroll to an element inside a div using JavaScript, you can use the `scrollTop` property of the parent div, setting it to the target element’s `offsetTop`. This adjustment allows you to smoothly navigate to a specific element within a scrollable container without using a full-page scroll. There are lots of methods to scroll to an element. The following are the methods available in JavaScript to scroll to an element.Â
The scrollIntoView() is used to scroll to the specified element in the browser.Â
Syntax:Â
element.scrollIntoView()
Example: In this example, we will see the implementation Using scrollIntoView() to scroll to an element. Â
html
<!DOCTYPE html>
< html >
< head >
< style >
#condiv {
height: 500px;
width: 500px;
overflow: auto;
background: #82c93a;
}
#ele {
top: 70%;
height: 200px;
width: 200px;
background-color: green;
position: absolute;
}
</ style >
</ head >
< body >
< p >Click the button to scroll to the element.</ p >
< button onclick = "scrolldiv()" >Scroll</ button >
< div id = "condiv" >
< div id = "ele" >
GeeksforGeeks
</ div >
</ div >
< script >
function scrolldiv() {
var elem = document.getElementById("ele");
elem.scrollIntoView();
}
</ script >
</ body >
</ html >
|
Output:Â
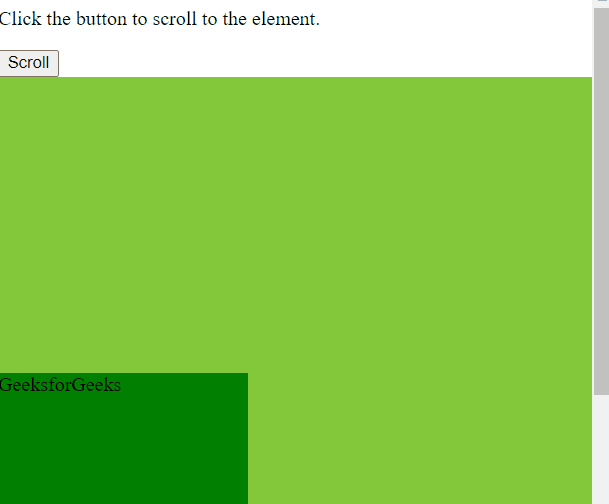
Output
The scroll() is used to scroll to the specified element in the browser.Â
Syntax:
element.scroll(x-cord,y-cord)
Example: In this example, we will see the implementation Using scroll() to scroll to an element.Â
html
<!DOCTYPE html>
< html >
< head >
< style >
#condiv {
height: 500px;
width: 500px;
overflow: auto;
background: #82c93a;
}
#ele {
top: 70%;
height: 200px;
width: 200px;
background-color: green;
position: absolute;
}
</ style >
</ head >
< body >
< p >Click the button to scroll to the element.</ p >
< button onclick = "scrolldiv()" >Scroll</ button >
< div id = "condiv" >
< div id = "ele" >
GeeksforGeeks
</ div >
</ div >
< script >
function scrolldiv() {
window.scroll(0,
findPosition(document.getElementById("ele")));
}
function findPosition(obj) {
var currenttop = 0;
if (obj.offsetParent) {
do {
currenttop += obj.offsetTop;
} while ((obj = obj.offsetParent));
return [currenttop];
}
}
</ script >
</ body >
</ html >
|
Output:Â
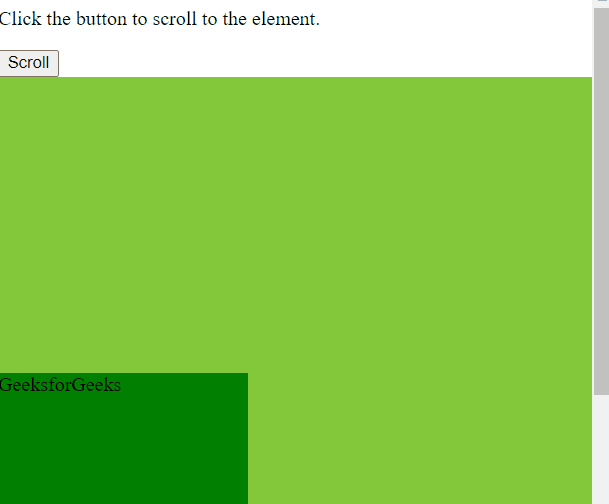
Output
The scrollTo() is used to scroll to the specified element in the browser.Â
Syntax:
element.scrollTo(x-cord,y-cord)
Example: In this example, we will see the implementation Using scrollTo() to scroll to an element.Â
html
<!DOCTYPE html>
< html >
< head >
< style >
#condiv {
height: 500px;
width: 500px;
overflow: auto;
background: #82c93a;
}
#ele {
top: 70%;
height: 200px;
width: 200px;
background-color: green;
position: absolute;
}
</ style >
</ head >
< body >
< p >Click the button to scroll to the element.</ p >
< button onclick = "scrolldiv()" >Scroll</ button >
< div id = "condiv" >
< div id = "ele" >
GeeksforGeeks
</ div >
</ div >
< script >
function scrolldiv() {
window.scrollTo(0,
findPosition(document.getElementById("ele")));
}
function findPosition(obj) {
var currenttop = 0;
if (obj.offsetParent) {
do {
currenttop += obj.offsetTop;
} while ((obj = obj.offsetParent));
return [currenttop];
}
}
</ script >
</ body >
</ html >
|
Output:Â
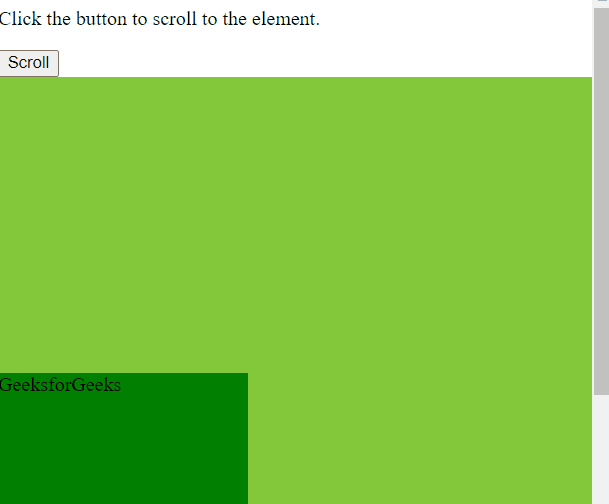
Output
Share your thoughts in the comments
Please Login to comment...