API(Application Programming Interface) is a set of protocols, rules, and tools that allow different software applications to access allowed functionalities, and data, and interact with each other. API is a service created for user applications that request data or some functionality from servers.
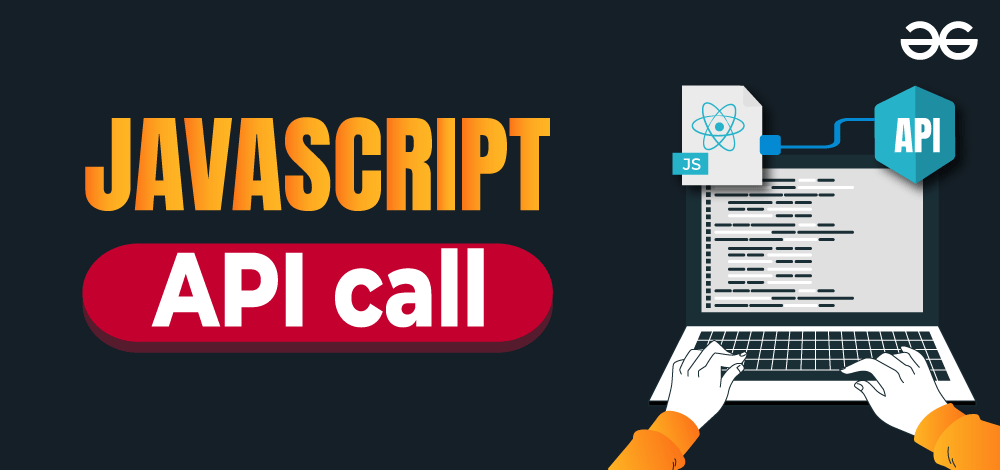
To give specific access to our system to other applications that may be useful to them, developers create APIs and give them endpoints to interact and access the server data. While working with JavaScript it is common to interact with APIs to fetch data or send requests to the server.
4 Ways to Make an API Call in JavaScript:
1. API Call in JavaScript Using XMLHttpRequest
XMLHttpRequest is an object used to make API calls in JavaScript. Before the release of ES6 which came with Fetch and libraries like Axios in 2015, it is the only way to call API.
XMLHttpRequests are still used in multiple places because all new and old browsers support this.Â
- To implement advanced features like request cancellation and progress tracking, XMLHttpRequest is used.
- To maintain old codebases which are written before the announcement of ES6, we use XMLHttpRequest
Example: In this example, XMLHttpRequest is used to make an API call.
Javascript
const xhttpr = new XMLHttpRequest();
xhttpr.open( 'GET' , 'Api_address' , true );
xhttpr.send();
xhttpr.onload = ()=> {
if (xhttpr.status === 200) {
const response = JSON.parse(xhttpr.response);
} else {
}
};
|
Explanation of the above code
Step A: Create an instance of XMLHttpRequest object.
const xhttpr = new XMLHttpRequest();
Step B: Make an open connection with the API endpoint by providing the necessary HTTP method and URL.
xhttpr.open('GET', 'Api_address', true);
Here ‘true’ represents that the request is asynchronous.
Step C: Send the API request
xhttpr.send();
Step D: Create a function onload when the request is completely received or loaded:
xhttpr.onload = ()=> {
if (xhttpr.status === 200) {
const response = JSON.parse(xhttpr.response);
// Process the response data here
} else {
// Handle error
}
};
status – HTTP status code of the response.
Since it is recommended to use newer alternatives like fetch and Axios. But using XMLHttpRequest will give you an understanding of the request-response lifecycle and how HTTP request works. This is how JavaScript API is called using XMLHttpRequest.
2. API Call in JavaScript Using the fetch() method
fetch is a method to call an API in JavaScript. It is used to fetch resources from a server. All modern browsers support the fetch method. It is much easy and simple to use as compared to XMLHttpRequest.
It returns a promise, which contains a single value, either response data or an error. fetch() method fetches resources in an asynchronous manner.
Example: In this example, fetch() is used to make an API call.
Javascript
fetch( 'Api_address' )
.then(response => {
if (response.ok) {
return response.json();
} else {
throw new Error( 'API request failed' );
}
})
.then(data => {
console.log(data);
})
. catch (error => {
console.error(error);
});
|
Explanation of the above code
Step A: Make an API request to the URL endpoint.
Pass the API URL to the fetch() method to request the API which will return the Promise.
fetch('Api_address')
Step B: Handle the response and parse the data.
Use the .then() method to handle the response. Since the response object has multiple properties and methods to access data, use the appropriate method to parse the data. If the API return JSON data, then use .then() method.
.then(response => {
if (response.ok) {
return response.json(); // Parse the response data as JSON
} else {
throw new Error('API request failed');
}
})
Step C: Handle the parsed data
Make another .then() method to handle the parsed data. Inside that, you can use that data according to your need.
.then(data => {
// Process the response data here
console.log(data); // Example: Logging the data to the console
})
Step D: Handle the Error
To handle errors, use .catch() method at the end of the change.
.catch(error => {
// Handle any errors here
console.error(error); // Example: Logging the error to the console
});
3. API call in JavaScript using Axios
Axios is an open-source library for making HTTP requests to servers. It is a promise-based approach. It supports all modern browsers and is used in real-time applications. It is easy to install using the npm package manager.
It has better error handling than the fetch() method. Axios also does automatic transformation and returns the data in JSON format.
Example: In this example, Axios is used to make an API call.
Javascript
import axios from 'axios' ;
axios.get( 'APIURL' )
.then(response => {
const responseData = response.data;
})
. catch (error => {
});
|
Explanation: While sending the HTTP request it will respond to us with the error or the data which is already parsed to JSON format(which is the property of Axios). We can handle data with the .then() method and at the end we will use .catch() method to handle the error.
Note: Here we have to pass the URL and unlike fetch or XMLHttpRequest, we cannot pass the path to the resource.
4. API call in JavaScript Using the jQuery AJAX
jQuery is a library used to make JavaScript programming simple and if you are using it then with the help of the $.ajax() method you can make asynchronous HTTP requests to get data.
To learn more about jQuery AJAX, refer to this article: What is the use of jQuery ajax() method?
Example: In this example, jQuery AJAX is used to make an API call.
Javascript
$.ajax({
url: 'APIURL' ,
method: 'GET' ,
success: function (response) {
const parsedData = JSON.parse(response);
},
error: function (xhr, status, error) {
}
});
|
Explanation of the above example
Step A: Makinig an API request.
To make an HTTP request use the $.ajax() method and pass a URL inside it by giving the necessary method.
$.ajax({
url: 'APIURL',
method: 'GET',
})
Step B: Handling and Parsing the response.
We will use a callback function success to handle the response and if the response is present we will parse the data to JSON format.
success: function(response) {
const parsedData = JSON.parse(response);
// Process the parsed data here
}
Step C: Handling Error.
To handle an error during the API call, we will use the error callback function.Â
Here xhr object contains information about the request.
error: function(xhr, status, error) {
// Handle any errors
}
To handle the response, it has two callback functions success and error.Â
Conclusion
While working with API in JavaScript, you have multiple options to send requests. XMLHttpRequest is the oldest way to call API using creating an instance and handling the response using Event Handler. But after the release of ES6 in 2015, developers were given the option of the fetch() method and Axios library, which are more efficient. Both return Promises which can be handled using the .then()method or async/await method. But in the Axios library, it is easy to handle errors than the fetch() method. However, the choice of method depends on the user preference and the complexity of the project.
For Creating an API, Please refer to this article: Tips for Building an API
FAQs
1. What are the different ways to make an API call in JavaScript?
1. Using XMLHttpRequest
2. fetch() method
3. Using Axios library
4. Using jQuery AJAX
2. What are the different types of API?
There are 4 different types of APIs used in web services:
1. Public
2. Partner
3. Private
4. Composite
3. What are API call methods?
An API call is a process of sending a request to API by a user application and that API retrieves the requested data from the server and sends the response to the user application.
Share your thoughts in the comments
Please Login to comment...