How to make axios send cookies in its requests automatically?
Last Updated :
25 Dec, 2023
Cookies are small pieces of data stored on the client’s computer for various tasks. If we want to make Axios send cookies in its request automatically, then we can use the ‘withCrendentials‘ options. We need to set it to true in our Axios configuration. This makes sure that cookies are included in both requests and responses. In this article, we will see the practical implementation to automatically make Axios send cookies in its requests.
Prerequisites:
What is Axios?
Axios is the HTTP client that is used for making asynchronous requests in the Node.js and the browser environment. We can also use the Axios in the server-side communication. Using this, we can provide a clean and efficient way to handle the HTTP requests and responses received from the client request.
Approach to make axios send cookies in requests automatically:
- We have to set the cookies (name1 and name2) using the cookie-parser middleware.
- Then we made the Axios GET request on the /make-request route to the example endpoint, automatically sending the cookies with the withCredenitals:true option.
- Then the server responds with the JSON data which is received from the API.
Steps to make Axios send cookies in its requests automatically
Step 1: In the first step, we will create the new folder by using the below command in the VScode terminal.
mkdir folder-name
cd folder-name
Step 2: After creating the folder, initialize the NPM using the below command. Using this the package.json file will be created.
npm init -y
Step 3: Install all the required dependencies using the below command:
npm install express axios cookie-parser
Step 4: Now create the below Project Structure of our project which includes the file as app.js.
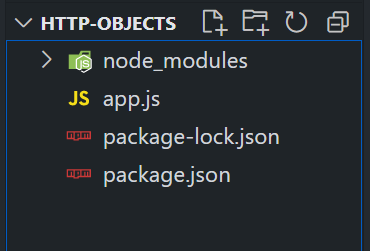
The updated dependencies in package.json file will look like:
"dependencies": {
"axios": "^1.6.2",
"cookie-parser": "^1.4.6",
"express": "^4.18.2"
}
Step 5: Use the below app.js code to make Axios send cookies in its requests automatically.
Example: Write the following code in App.js file.
Javascript
const axios = require( 'axios' );
const express = require( 'express' );
const cookieParser = require( 'cookie-parser' );
const app = express();
const port = 3000;
app.use(cookieParser());
app.get( '/make-request' , async (req, res) => {
try {
res.cookie( 'name1' , 'gfg1' );
res.cookie( 'name2' , 'gfg2' );
const response = await
axios.get(
headers: {
Cookie: req.headers.cookie,
},
});
const postData = response.data;
res.json(postData);
} catch (error) {
console.error('Error making Axios request: ', error.message);
res.status(500).json({ error: ' Internal Server Error' });
}
});
app.listen(port, () => {
console.log(`Server is running at http:
});
|
Step to run the application: Run the Server using the following command.
node server.js
Output:
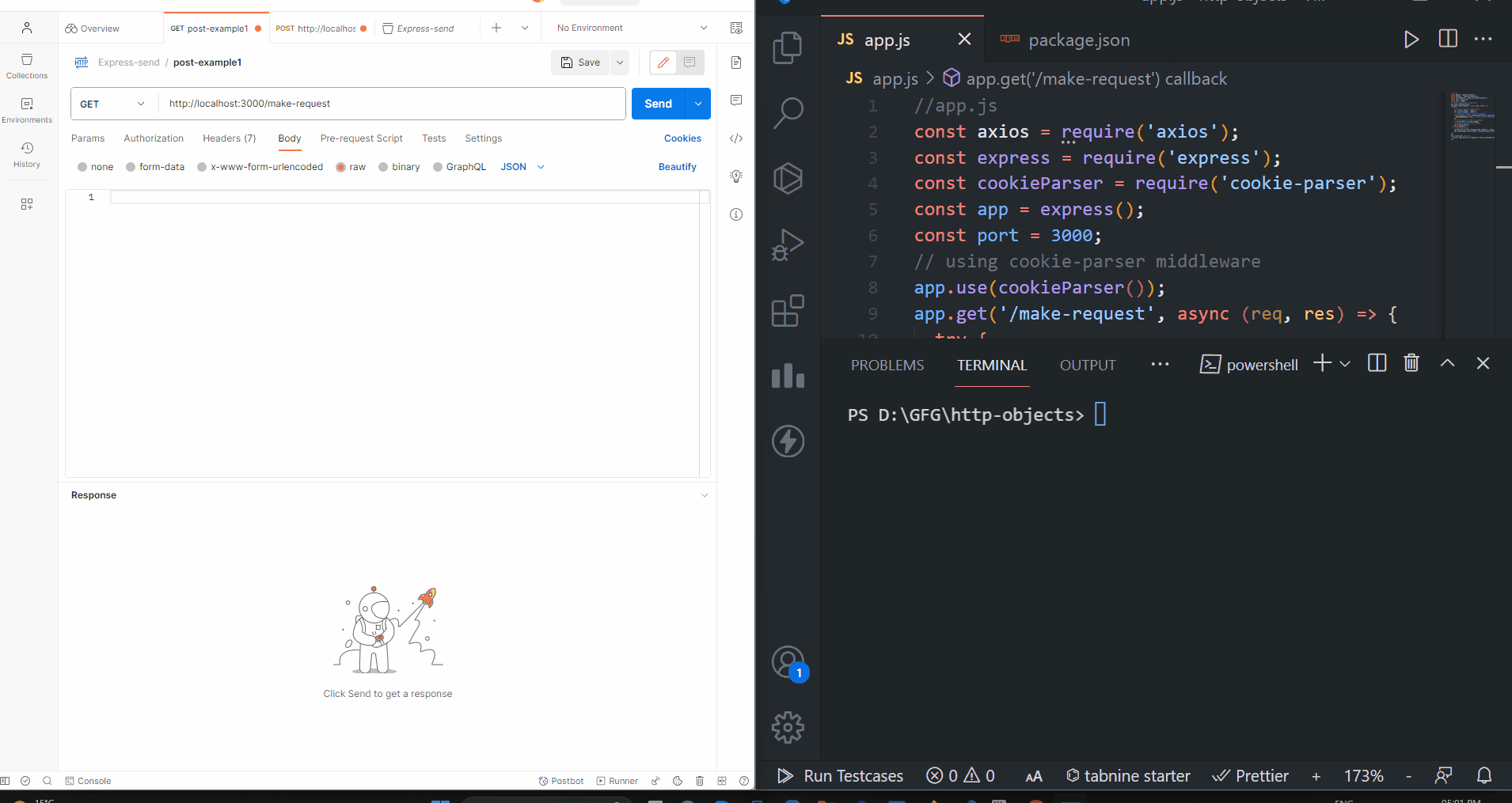
Share your thoughts in the comments
Please Login to comment...