How to Loop through Object with *ngFor in Angular ?
Last Updated :
29 Nov, 2023
JSON stands for JavaScript Object Notation. It is a format for structuring data. This format is used by different web applications to communicate with each other. In this article, we will learn Loop through Object with *ngFor in Angular.
Steps for Installing & Configuring the Angular Application
Step 1: Create an Angular application using the following command.
ng new appname
Step 2: After creating your project folder i.e. appname, move to it using the following command.
cd appname
Project Structure
It will look like the following:
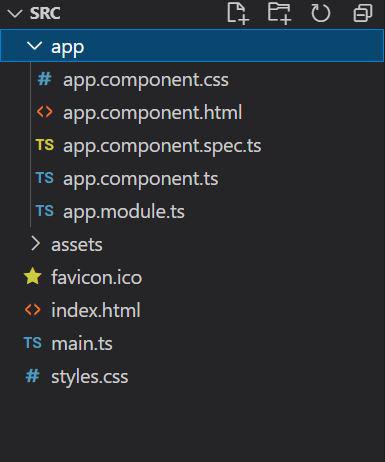
Using Pipe
Angular has added a new built-in pipe to help you iterate through JSON objects, in the common module of the Angular package.
Example: This example illustrates iterating over them using ngFor in Angular.
HTML
< h2 style = "color: green" >GeeksforGeeks</ h2 >
< h2 >Loop through Object with *ngFor </ h2 >
< div * ngFor = "let item of organism | keyvalue" >
organism: < b >{{item.key}}</ b > and Type: < b >{{item.value}}</ b >
</ div >
|
Javascript
import { Component, OnInit } from '@angular/core' ;
import { KeyValue } from '@angular/common' ;
@Component({
selector: 'app-root' ,
templateUrl: "./app.component.html" ,
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
organism: { [key: string]: string } =
{
'Wood' : 'Abiotic' ,
'Plants' : 'Biotic' ,
'Fan' : 'Abiotic' ,
'Animals' : 'Biotic'
};
}
|
Javascript
import { NgModule }
from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { HttpClientModule }
from '@angular/common/http' ;
import { AppComponent }
from './app.component' ;
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
HttpClientModule,
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
|
Output:
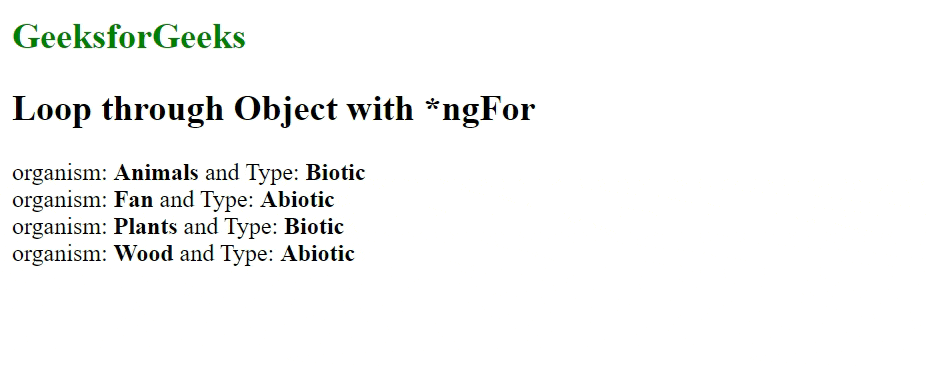
Using Object.keys() Method
The Object.keys() method returns an Array Iterator object with the keys of an object. We will get those keys of our object and iterate over the object using ngFor.
Example: This is another example that illustrates iterating over them using ngFor in Angular.
HTML
< h2 style = "color: green" >GeeksforGeeks</ h2 >
< h2 >Loop through Object with *ngFor </ h2 >
< li * ngFor = "let key of keys()" >{{key}}:{{states[key]}}</ li >
|
Javascript
import { Component, OnInit } from '@angular/core' ;
import { HttpClient } from '@angular/common/http' ;
@Component({
selector: 'app-root' ,
templateUrl: "./app.component.html" ,
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
states: Dictionary;
constructor() {
this .states = {
'Uttar Pradesh' : 'Lucknow' , 'Tripura' : 'Agartala' ,
'West Bengal' : 'Kolkata' , 'Rajasthan' : 'Jaiput'
};
}
keys(): Array<string> {
return Object.keys( this .states);
}
}
interface Dictionary {
[index: string]: string
}
|
Javascript
import { NgModule }
from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { HttpClientModule }
from '@angular/common/http' ;
import { AppComponent }
from './app.component' ;
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
HttpClientModule,
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
|
Output:
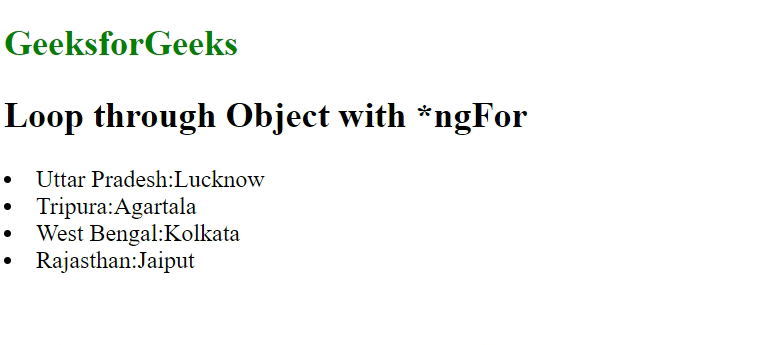
Share your thoughts in the comments
Please Login to comment...