How to limit the ngFor Loop to Two Iterations in Angular ?
Last Updated :
29 Nov, 2023
The NgFor is used as a structural directive that renders each element for the given collection each element can be displayed on the page. In this article, we will learn how to Limit the ngFor loop to two iterations.
Steps for Installing & Configuring the Angular Application
Step 1: Create an Angular application using the following command.
ng new appname
Step 2: After creating your project folder i.e. appname, move to it using the following command.
cd appname
Project Structure
It will look like the following
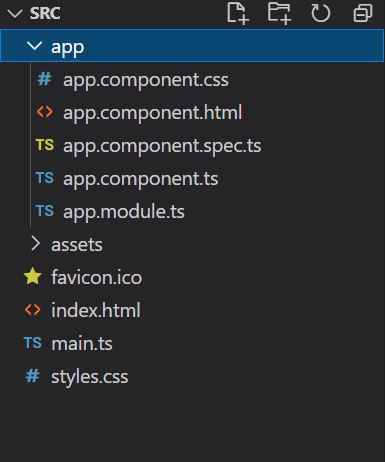
Using ngIf
In this approach, we will use ngIf with index, and print the value only if index<2. Here, we have 4 objects but only 2 will be iterated.
Example: This example illustrates limiting the ngFor loop to 2 iterations.
Javascript
import { Component, OnInit } from '@angular/core' ;
import { KeyValue } from '@angular/common' ;
import { Pipe, PipeTransform } from '@angular/core' ;
@Component({
selector: 'app-root' ,
templateUrl: "./app.component.html" ,
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
gfg: any = {
"HTML" : "Hyper Text Markup Language" ,
"CSS" : "Cascade Style Sheet" ,
"XML" : "Xtensive Markup Language" ,
"JS" : "Javascript"
}
}
|
Javascript
import { NgModule }
from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { HttpClientModule }
from '@angular/common/http' ;
import { AppComponent } from './app.component' ;
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
HttpClientModule,
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
|
HTML
< h2 style = "color: green" >GeeksforGeeks</ h2 >
< h2 >Limit the ngFor loop to two iterations</ h2 >
< div * ngFor = "let item of gfg |keyvalue;let i=index" >
< div * ngIf = "i<2" >
< b style = "color: green;" >{{i+1}} </ b >
< b >{{item.key}}:</ b > {{item.value}}
</ div >
</ div >
|
Output
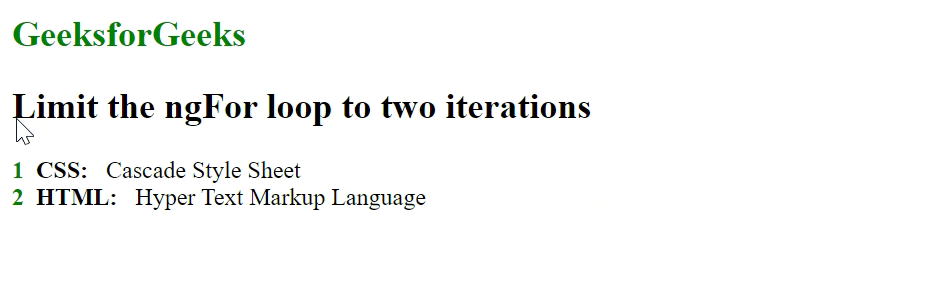
Using slice
In this approach, we will slice the given Array of objects and then use keyvalue to iterate over the object.
Example: This is another example that illustrates limiting the ngFor loop to 2 iterations.
app.component.html
Javascript
import { Component, OnInit } from '@angular/core' ;
import { KeyValue } from '@angular/common' ;
import { Pipe, PipeTransform } from '@angular/core' ;
@Component({
selector: 'app-root' ,
templateUrl: "./app.component.html" ,
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
gfg: Array<{ course: string, text: string }> = [
{ course: "HTML" , text: "Hyper Text Markup Language" },
{ course: "CSS" , text: "Cascade Style Sheet" },
{ course: "XML" , text: "Xtensive Markup Language" },
{ course: "JS" , text: "Javascript" }
]
gfg2: any
sliceObject() {
this .gfg2 = this .gfg.slice(0, 2)
}
getObject(obj: any) {
return Object.values(obj)
}
console = console
}
|
Javascript
import { NgModule }
from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { HttpClientModule }
from '@angular/common/http' ;
import { AppComponent } from './app.component' ;
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
HttpClientModule,
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
|
HTML
< h2 style = "color: green" >GeeksforGeeks</ h2 >
< h2 >Limit the ngFor loop to two iterations</ h2 >
{{sliceObject()}}
< div * ngFor = "let item of gfg2 |keyvalue;" >
< b style = "color: red;" >{{item.key}}: </ b >
{{getObject(item.value)}}
</ div >
|
Output:
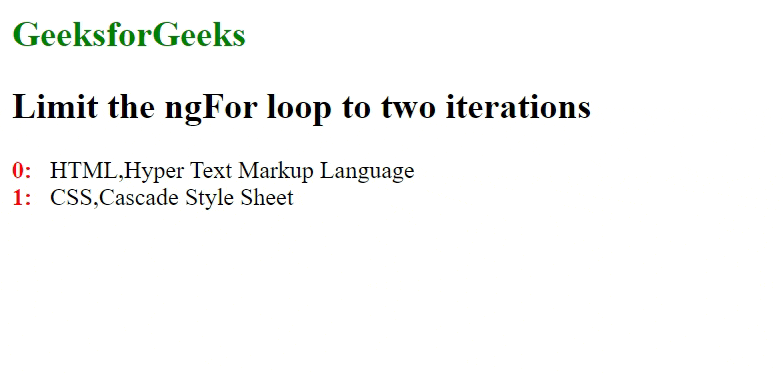
Share your thoughts in the comments
Please Login to comment...