How to apply filters to *ngFor in Angular ?
Last Updated :
15 Nov, 2023
In this article, we will see How to apply filters to *ngFor in AngularJS, along with understanding their basic implementation through the examples. NgFor is used as a Structural Directive that renders each element for the given collection each element can be displayed on the page. Implementing the filters to the ‘*ngFor’ directive conditionally helps to render the items or manipulate the collection of items in an iterative manner. To accomplish this task, we can implement the Pipes, which will take the array of items along with additional parameters for filtering
Syntax
<span *ngFor="item of itemsList">
<div *ngIf="yourCondition(item)">
<!-- Your code -->
</div>
</span>
Setting the Angular Application
Step 1: Create an Angular application using the following command.
ng new appname
Step 2: After creating your project folder i.e. appname, move to it using the following command.
cd appname
Project Structure
It will look like the following:
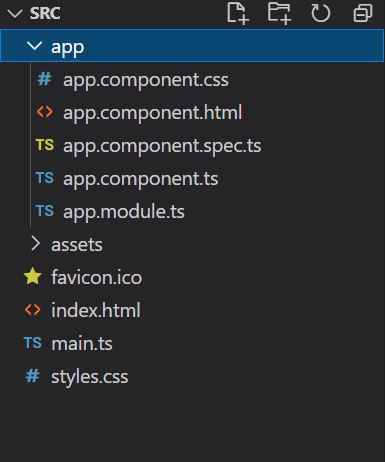
Example 1: In this example, we will use pipe and filter objects. Out of 4 objects, we will filter 2 objects and render them on the screen.
HTML
< h2 style = "color: green" >GeeksforGeeks</ h2 >
< h2 >How to apply filters to *ngFor in AngularJS?</ h2 >
< div * ngFor = "let item of gfg |keyvalue;let i=index" >
< div * ngIf = "i<2" >
< b style = "color: green;" >{{i+1}} </ b >
< b >{{item.key}}:</ b > {{item.value}}
</ div >
</ div >
|
Javascript
import { Component, OnInit }
from '@angular/core' ;
import { KeyValue }
from '@angular/common' ;
import { Pipe, PipeTransform }
from '@angular/core' ;
@Component({
selector: 'app-root' ,
templateUrl: "./app.component.html" ,
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
gfg: any = {
"HTML" : "Hyper Text Markup Language" ,
"CSS" : "Cascade Style Sheet" ,
"XML" : "Xtensive Markup Language" ,
"JS" : "Javascript"
}
}
|
Javascript
import { NgModule }
from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { HttpClientModule }
from '@angular/common/http' ;
import { AppComponent }
from './app.component' ;
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
HttpClientModule,
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
|
Output:
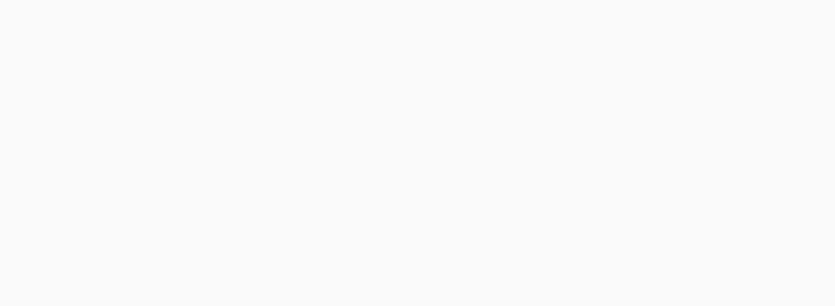
Example 2: In this example, we will apply the filter to the element using ngFor such that only the element with key=”CSS” will be displayed on the screen.
HTML
< h2 style = "color: green" >
GeeksforGeeks
</ h2 >
< h2 >
How to apply filters to
*ngFor in AngularJS?
</ h2 >
< div * ngFor = "let item of gfg |keyvalue;let i=index" >
< div * ngIf = "displayCondition(item.key)" >
< b style = "color: green;" >{{i+1}} </ b >
< b >{{item.key}}:</ b > {{item.value}}
</ div >
</ div >
|
Javascript
import { Component, OnInit }
from '@angular/core' ;
import { KeyValue }
from '@angular/common' ;
import { Pipe, PipeTransform }
from '@angular/core' ;
@Component({
selector: 'app-root' ,
templateUrl: "./app.component.html" ,
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
gfg: any = {
"HTML" : "Hyper Text Markup Language" ,
"CSS" : "Cascade Style Sheet" ,
"XML" : "Xtensive Markup Language" ,
"JS" : "Javascript"
}
displayCondition(obj: any) {
if (obj == "CSS" ) {
return true ;
}
return false ;
}
}
|
Javascript
import { NgModule }
from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { HttpClientModule }
from '@angular/common/http' ;
import { AppComponent }
from './app.component' ;
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
HttpClientModule,
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
|
Output:
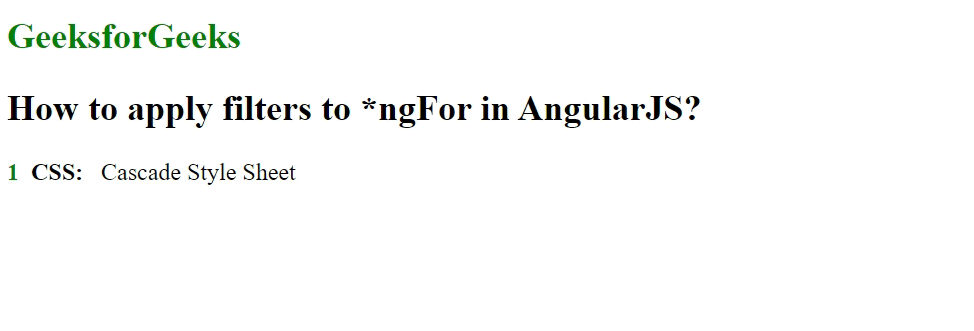
Share your thoughts in the comments
Please Login to comment...