How to limit ngFor repeat to some number of items in Angular ?
Last Updated :
14 Nov, 2023
In AngularJS, we can use the ngFor directive to loop over a list of items and display them on a webpage. Although, there could be some cases where we don’t want to use all the elements in the list but want to display only a limited number of initial items, or the paginated items from the list. In this article, we will see how to limit the ngFor directive to repeat to some number of items only, along with understanding their basic implementation through the illustrations.
We will explore the above approaches with the help of suitable examples.
Implementing the SlicePipe
In this approach, we will use SlicePipe in Angular which returns a subset, or a slice, of elements from a list. It takes the indexes, i.e. start and end index and returns the elements from the start to the end index, with the end index element not included. Here, we will add items array & loop over the items array using the ngFor directive, and limit the items to just 5 using SlicePipe.
Example: This example describes the imiting the ngFor repeat to some number of items in Angular.
Javascript
import { Component } from '@angular/core' ;
@Component({
selector: 'app-root' ,
templateUrl: './app.component.html' ,
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
items = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
}
|
HTML
< div * ngFor = "let item of items | slice:0:5" >
{{ item }}
</ div >
|
Output:
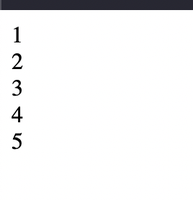
Using a function in the component
In this approach, we will create a function that returns a subset of elements from the list. We will define the function in the component typescript file, and then use the function in the html template. This function will internally use the slice javascript method to slice the elements, from start to end index, and return those elements as a list.
Example: In this example, we will add a getLimitedItems function inside the app.component.ts file, that takes in a start index, and an end index, and returns the items between those indices. Then, we will use the getLimitedItems function insde the html template to get the first 5 items from the items list.
Javascript
import { Component } from '@angular/core' ;
@Component({
selector: 'app-root' ,
templateUrl: './app.component.html' ,
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
items = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
getLimitedItems(startIndex: number,
endIndex: number): number[] {
return this .items.slice(startIndex, endIndex);
}
}
|
HTML
< div * ngFor = "let item of getLimitedItems(0, 5)" >
{{ item }}
</ div >
|
Output:
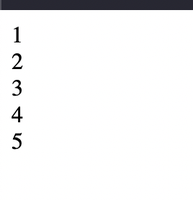
Share your thoughts in the comments
Please Login to comment...