Use of *ngIf and *ngFor Directives in Angular
Last Updated :
28 Mar, 2024
Angular is a very popular framework for building scalable web applications. It provides many features and tools to make the development process smooth. Two important directives in Angular are *ngIf and *ngFor. *ngIf is used to conditionally render HTML elements, while *ngFor is used to iterate over a collection of data. In this article, we will learn the use of *ngIf and *ngFor directives in Angular.
Steps to Create Angular Application:
Step 1: Create a new Angular application using the Angular CLI with the following command:
ng new my-angular-app
Step 2: Navigate to the project directory:
cd my-angular-app
Folder Structure:
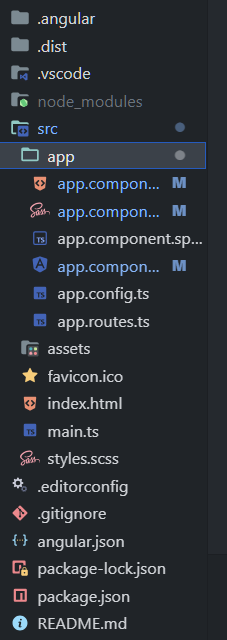
Folder Structure
The updated dependencies in package.json file will look like:
"dependencies": {
"@angular/animations": "^17.3.0",
"@angular/common": "^17.3.0",
"@angular/compiler": "^17.3.0",
"@angular/core": "^17.3.0",
"@angular/forms": "^17.3.0",
"@angular/platform-browser": "^17.3.0",
"@angular/platform-browser-dynamic": "^17.3.0",
"@angular/router": "^17.3.0",
"rxjs": "~7.8.0",
"tslib": "^2.3.0",
"zone.js": "~0.14.3"
}
1. *ngIF directive in Angular:
ngIf is used to display or hide the DOM Element based on the expression value assigned to it. The expression value may be either true or false.
Syntax:
<element *ngIf="condition">...</element>
Uses of *ngIf Directive:
- Conditional Rendering:
*ngIf
is commonly used for conditional rendering of elements based on various conditions, such as user authentication status, data availability, or user interactions. - Error Handling:
*ngIf
can be used to display error messages or fallback content when certain conditions are not met, providing a seamless user experience. - Dynamic UI Components:
*ngIf
enables the dynamic rendering of UI components based on application state or user inputs, allowing for a more interactive and personalized user experience.
Example: Now we use *ngIf to conditionally render a message, based to a particular condition(either true or false) it changes the DOM. Here we have used a vaiable named “show”. If show is true then the text will be shown present in the div, if it is false then text will not be shown in the div.
HTML
<!-- app.component.html -->
<div class="center">
<h2 class="green">
GeeksForGeeks
</h2>
<h2 class="title">
*NgIf directive in <span class="angular">Angular</span>
</h2>
<div class="bgGreen">
<p *ngIf="show">
This text will be shown <br> if the "show" varable is true
</p>
</div>
</div>
CSS
/* app.component.css */
.center {
display: grid;
justify-content: center;
align-items: center;
text-align: center;
line-height: 1.5rem;
}
.green {
color: rgb(28, 143, 28);
font-size: 2.5rem;
text-align: center;
}
.title {
color: rgb(57, 23, 180);
text-align: center;
}
.angular {
color: rgb(237, 40, 40);
font-size: 2.1rem;
}
.bgGreen {
background-color: rgb(28, 143, 28);
color: #ffff;
}
JavaScript
// app.component.ts
import { Component } from '@angular/core';
import { RouterOutlet } from '@angular/router';
import { CommonModule } from '@angular/common';
import { NgIf } from '@angular/common';
@Component({
selector: 'app-root',
standalone: true,
imports: [RouterOutlet, CommonModule, NgIf],
templateUrl: './app.component.html',
styleUrl: './app.component.scss'
})
export class AppComponent {
title = 'structuralD';
show = true;
}
Output:
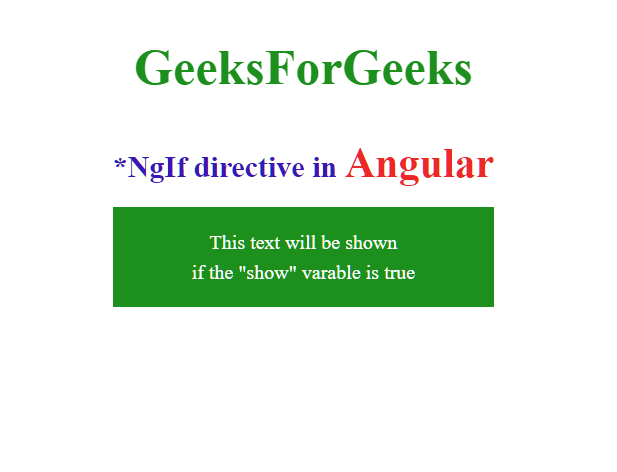
*ngIf directive in Angular
2. *ngFor directive in Angular:
*ngFor is used to loop through the dynamic lists in the DOM. Simply, it is used to build data presentation lists and tables in HTML DOM.
Syntax:
<element *ngFor="let item of items">...</element>
Uses of *ngFor Directive:
- Rendering Lists:
*ngFor
is primarily used for rendering lists of items retrieved from APIs, databases, or local data sources, providing a dynamic and data-driven user interface. - Dynamic Table Rows:
*ngFor
can be used to generate table rows dynamically based on the data in the underlying collection, allowing for the creation of dynamic and responsive tables. - Iterating Over Object Properties:
*ngFor
can iterate over the properties of an object, allowing you to render key-value pairs dynamically in the UI.
In Angular, *ngFor is used to iterate over a list of items. Here in ngfor, we need a local varable which represents each item in the object boxes which we have created in app.components.ts file. Now we will iterate over each item in boxes and show its proprties (size, color, height) using *ngFor directive in angular. For showing the object we need to provide a template for each item in boxes.
Example:
HTML
<!-- app.component.html -->
<div class="center">
<h2 class="green">
GeeksForGeeks
</h2>
<h2 class="title">
*NgFor directive in <span class="angular">Angular</span>
</h2>
<div class="bgGreen">
<div *ngFor="let box of boxes">
{{box.size}},
{{box.color}},
{{box.height}}
</div>
</div>
</div>
CSS
/* app.component.css */
.center {
display: grid;
justify-content: center;
align-items: center;
text-align: center;
line-height: 1.5rem;
}
.green {
color: rgb(28, 143, 28);
font-size: 2.5rem;
text-align: center;
}
.title {
color: rgb(57, 23, 180);
text-align: center;
}
.angular {
color: rgb(237, 40, 40);
font-size: 2.1rem;
}
.bgGreen {
background-color: rgb(28, 143, 28);
color: #ffff;
}
JavaScript
// app.component.ts
import { Component } from '@angular/core';
import { RouterOutlet } from '@angular/router';
import { CommonModule } from '@angular/common';
import { NgFor } from '@angular/common';
@Component({
selector: 'app-root',
standalone: true,
imports: [RouterOutlet, CommonModule, NgFor],
templateUrl: './app.component.html',
styleUrl: './app.component.scss'
})
export class AppComponent {
title = 'structuralD';
boxes = [
{ size: "SM", color: "white", height: 5 },
{ size: "M", color: "grey", height: 10 },
{ size: "L", color: "Green", height: 15 },
{ size: "XL", color: "Orange", height: 20 },
{ size: "XXL", color: "Black", height: 25 }
];
}
Output:
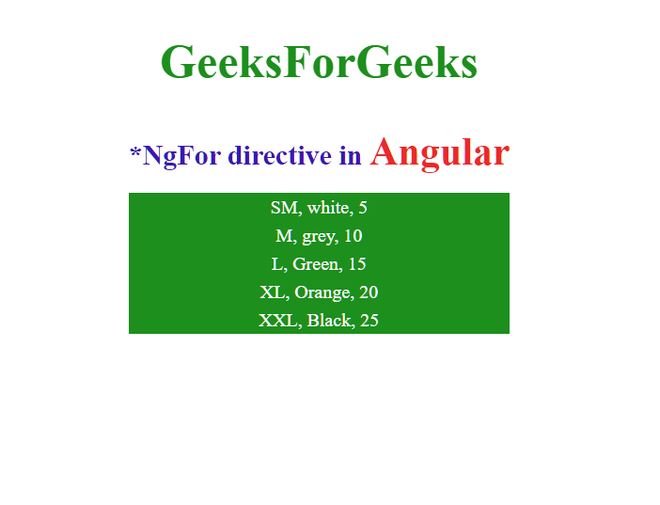
*ngFor directive in Angular
Conclusion
*ngIf is very useful directive in Angular for controlling the visibility of HTML elements in DOM and *ngFor directives is a powerful directive to iterate over data collections. By understanding their syntax and usage of *ngIf and *ngFor directives in Angular, we can enhance the interactivity and flexibility of your Angular applications.
Share your thoughts in the comments
Please Login to comment...