How to Loop through array of JSON object with *ngFor in Angular ?
Last Updated :
15 Dec, 2023
JavaScript Object Notation (JSON) is a text-based, human-readable interchange format used for representing simple data structures and objects in web browser-based code. In order to Loop through an array of JSON objects, the *ngFor directive can be utilized that helps to dynamically generate HTML content based on the data in those objects. For instance, we have an array of JSON objects, shown below:
[
{
"name": "Java",
"feature": [
{
"name": "ObjectOriented"
},
{
"name": "Abstraction"
},
{
"name": "Encapsulation"
}
]
},
{
"name": "HTML",
"feature": [
{
"name": "FrontEnd"
},
{
"name": "Styling"
}
]
}
]
These JSON objects are in the form of key-value pairs and are also present in nested JSON format. In this article, we will learn How to iterate over these Array of JSON objects/ nested JSON objects using ngFor Directive in Angular.
Creating Angular application & Module Installation
Step 1: Create an Angular application using the following command.
ng new appname
Step 2: After creating your project folder i.e. appname, move to it using the following command.
cd appname
Project Structure
It will look like the following:
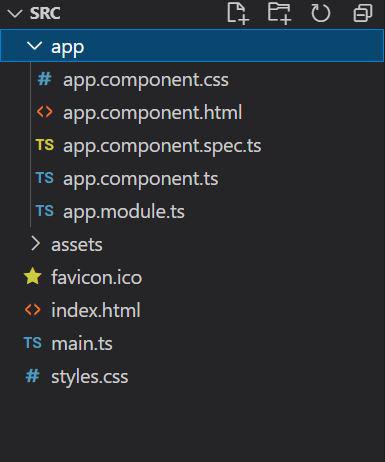
Example 1: In this example, we will iterate through an array of JSON objects and display them in a list.
HTML
< h2 style = "color: green" >GeeksforGeeks</ h2 >
< h2 >
Loop through array of
JSON object with *ngFor
</ h2 >
< div * ngFor = "let item of gfg " >
{{item.name}}
< ul >
< p * ngFor = "let element of item.feature" >
< li >{{element.name}} </ li >
</ p >
</ ul >
</ div >
|
Javascript
import { Component, OnInit } from '@angular/core' ;
import { KeyValue } from '@angular/common' ;
@Component({
selector: 'app-root' ,
templateUrl: "./app.component.html" ,
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
gfg = [
{
"name" : "Java" ,
"feature" : [
{ "name" : "ObjectOriented" },
{ "name" : "Abstraction" },
{ "name" : "Encapsulation" }
]
},
{
"name" : "HTML" ,
"feature" : [
{ "name" : "FrontEnd" },
{ "name" : "Styling" }
]
}
]
}
|
Javascript
import { NgModule }
from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { HttpClientModule }
from '@angular/common/http' ;
import { AppComponent }
from './app.component' ;
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
HttpClientModule,
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
|
Output:
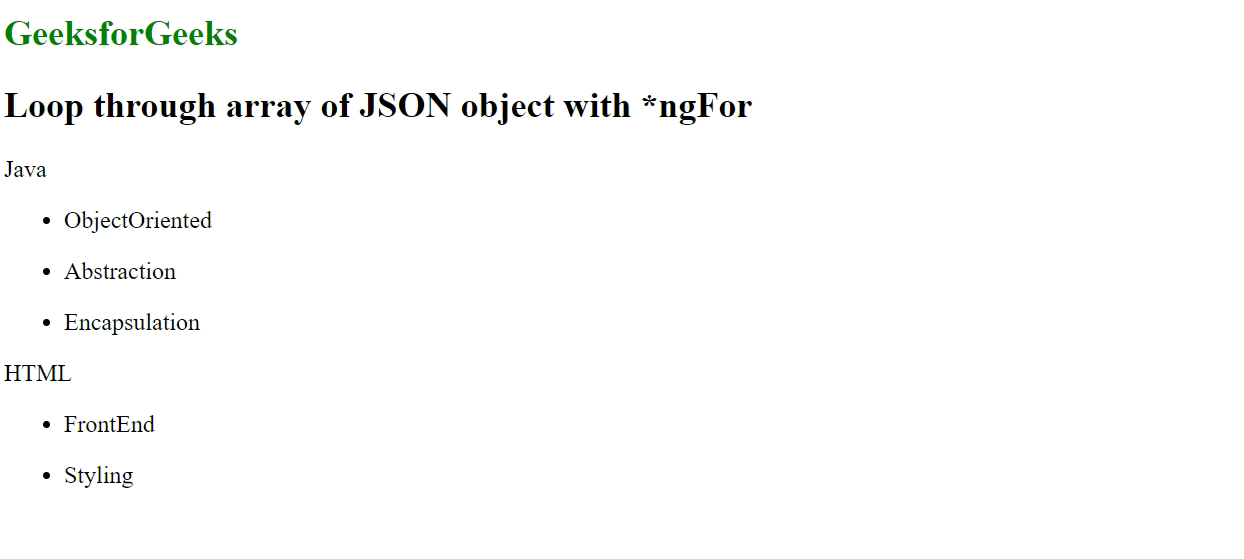
Example 2: In this example, we will use the function Object.Keys() and get the values of the keys and then iterate over them.
HTML
< h2 style = "color: green" >GeeksforGeeks</ h2 >
< h2 >
Loop through array of JSON
object with *ngFor
</ h2 >
< div * ngFor = "let item of keys() " >
{{item[1].name}}
< ul >
< li * ngFor = "let element of item[1].feature" >
{{element.name}}
</ li >
</ ul >
</ div >
|
Javascript
import { Component, OnInit } from '@angular/core' ;
import { KeyValue } from '@angular/common' ;
@Component({
selector: 'app-root' ,
templateUrl: "./app.component.html" ,
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
gfg = [
{
"name" : "Java" ,
"feature" : [
{ "name" : "ObjectOriented" },
{ "name" : "Abstraction" },
{ "name" : "Encapsulation" }
]
},
{
"name" : "HTML" ,
"feature" : [
{ "name" : "FrontEnd" },
{ "name" : "Styling" }
]
}
]
keys(): Array<any> {
console.log(Object.entries( this .gfg))
return Object.entries( this .gfg);
}
}
|
Javascript
import { NgModule }
from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { HttpClientModule }
from '@angular/common/http' ;
import { AppComponent }
from './app.component' ;
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
HttpClientModule,
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
|
Output:
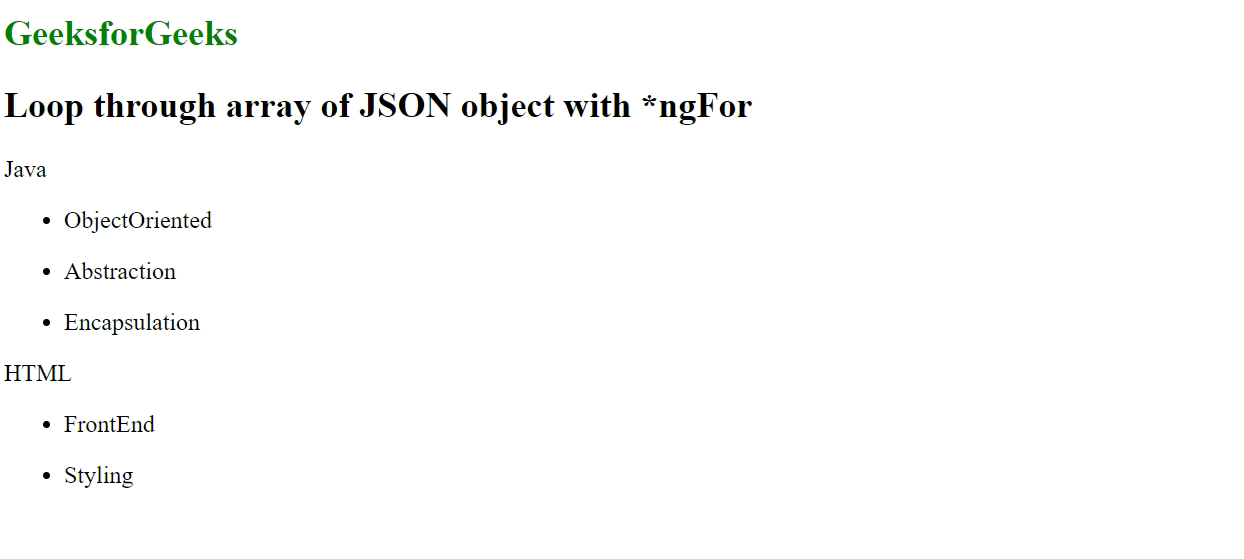
Share your thoughts in the comments
Please Login to comment...