How do CSS Modules help in styling React components?
Last Updated :
08 Mar, 2024
CSS Modules help in styling React components by providing a way to create modular stylesheets where each component’s styles are contained within its own module.
This reduces the risk of naming clashes, ensures that changes in one component won’t affect others, and makes it clear which styles are associated with each component. This simplifies debugging and maintenance.
To Create a Basic React Application Setup refer to the Folder Structure for a React JS Project article.
Utilizing CSS Modules
React CSS Modules is useful in large and complex apps to attain a more organized, maintainable, and scalable codebase, improving development efficiency and user experience. It’s useful as it provides a standardized way of handling styles.
CSS Modules is implemented by creating distinct class names for each component and adding those names to the HTML when the component is displayed. CSS Module stylesheet has the extension, which is typically .module.css which is the only difference between a standard stylesheet and CSS Module.
Example of using CSS Modules in React Components
Code: Here, we refer to the classes .bgColor, .greenText, and .redText from the imported styles, and the styles get implemented on the elements. We can use the necessary effects by referencing each class in the stylesheet using the styles object.
CSS
.bgColor {
background : lightgreen;
}
.redText {
color : red ;
font-size : 28px ;
}
.greenText {
color : darkgreen;
font-size : 45px ;
}
|
Javascript
import styles from './gfg.module.css'
function App() {
return (
<>
<div className={styles.bgColor}>
<h1 className={styles.greenText}>GFG</h1>
<h3 className={styles.redText}>CSS Module is simple</h3>
</div>
</>
)
}
export default App
|
Output:
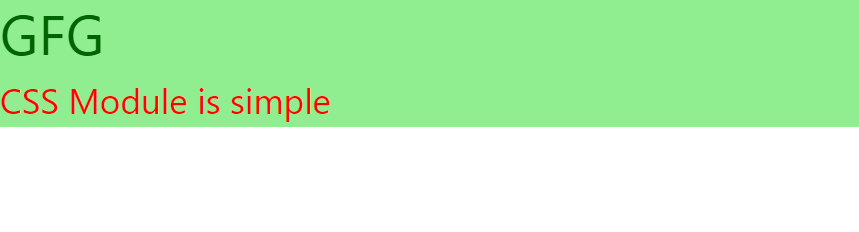
Advantages of using CSS Modules:
- Modularity: Each component’s styles are contained within its own module, reducing the risk of naming clashes.
- Scoped Styles: Styles are scoped locally, ensuring that changes in one component won’t affect others.
- Readability: Referencing styles as objects in your code makes it clear which styles are associated with each component.
- Ease of Maintenance: When you update or refactor a component, its styles remain isolated. You won’t accidentally break other components’ styling. This simplifies debugging and maintenance.
Share your thoughts in the comments
Please Login to comment...