CSS Modules : Local Scope for styles in React
Last Updated :
15 Mar, 2024
CSS Modules enable you to write styles in CSS files and then consume them as JavaScript objects, offering additional processing capabilities and enhancing safety. They have gained popularity due to their automatic generation of unique class and animation names, alleviating concerns about selector name collisions.
What is CSS Modules?
CSS Modules are CSS files where all class names and animation names are scoped locally by default. They allow you to write styles in CSS files, but when consumed, they are processed into JavaScript objects. CSS Modules are widely favored because they automatically ensure that class and animation names are unique, mitigating concerns about selector name collisions.
When to use CSS Modules?
For beginner to React, CSS Modules come highly recommended. They enable you to write conventional CSS files that remain portable, while also providing performance advantages such as bundling only the referenced code. Use CSS Modules when you want to keep your styles scoped to specific components without worrying about naming conflicts, making it easier to manage and maintain your CSS code.
Concept of Local Scope for styles in CSS modules:
- In CSS Modules, the concept of local scope for styles refers to the practice of encapsulating styles within individual components.
- Each component gets its unique CSS class names automatically generated during the build process. These class names are then scoped locally to the component, meaning they won’t affect styles outside of that component.
- This local scoping helps prevent conflicts and makes it easier to manage styles within large applications.
- Essentially, it allows you to style components in isolation without worrying about unintended side effects on other parts of your application.
Local Scope in CSS Modules:
Styles in traditional CSS are applied worldwide, which makes it difficult to prevent accidental style overrides and naming conflicts. By establishing local scope, which keeps styles defined inside a module separate from the rest of the application, CSS Modules solve this problem.
Example: Let’s consider a simple example to illustrate the local scope concept in CSS Modules. Assume you have a button component with a CSS file named “button.module.css”. In this example, the styles for the button are defined with a class named “.button“. However, the key difference is that these styles are locally scoped to the “button” module.
CSS
/* button.module.css */
.button {
background-color: #3498db;
color: #ffffff;
padding: 10px 15px;
border: none;
border-radius: 5px;
cursor: pointer;
}
.button:hover {
background-color: #217dbb;
}
Usage in JavaScript/React Component:
To use the styles defined in the “button.module.css” file, you import the module in your JavaScript or React component. Here, the “styles.button” syntax ensures that the styles are locally scoped to the “Button” component, avoiding any unintended global style conflicts.
CSS
/* button.module.css */
.button {
background-color: #3498db;
color: #ffffff;
padding: 10px 15px;
border: none;
border-radius: 5px;
cursor: pointer;
}
.button:hover {
background-color: #217dbb;
}
Javascript
// Button.js
import React from 'react';
import styles from './button.module.css';
const Button = () => {
return (
<button className={styles.button}>
Click me
</button>
);
};
export default Button;
JavaScript
//app.js
import './App.css';
import Button from './Button';
function App() {
return (
<div className="App">
<Button/>
</div>
);
}
export default App;
Output:
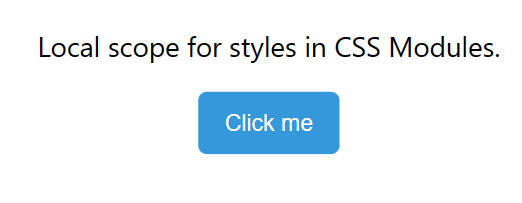
Local scope for styles in CSS modules.
Advantages of Local Scope:
- Isolation: Since each module runs separately, unintentional stylistic interference from other program components is avoided.
- Maintainability: Local scope facilitates better code structure and readability by making it simpler to manage and maintain styles for specific components.
- Reusability: Styles that are locally scoped allow components to be reused without worrying about clashing in multiple situations, making them more portable.
Conclusion:
With CSS Modules, we can effectively scope styles to specific components in our application without relying on large naming conventions, making it simpler to monitor the usage of styles across our codebase. We’re enthusiastic about leveraging them in our future endeavors and would encourage others to explore their potential for enhancing CSS maintainability.
Share your thoughts in the comments
Please Login to comment...